1153 Decode Registration Card of PAT (25 分)
A registration card number of PAT consists of 4 parts:
- the 1st letter represents the test level, namely,
T
for the top level,A
for advance andB
for basic; - the 2nd - 4th digits are the test site number, ranged from 101 to 999;
- the 5th - 10th digits give the test date, in the form of
yymmdd
; - finally the 11th - 13th digits are the testee's number, ranged from 000 to 999.
Now given a set of registration card numbers and the scores of the card owners, you are supposed to output the various statistics according to the given queries.
Input Specification:
Each input file contains one test case. For each case, the first line gives two positive integers N (≤104) and M (≤100), the numbers of cards and the queries, respectively.
Then N lines follow, each gives a card number and the owner's score (integer in [0,100]), separated by a space.
After the info of testees, there are M lines, each gives a query in the format Type Term
, where
Type
being 1 means to output all the testees on a given level, in non-increasing order of their scores. The correspondingTerm
will be the letter which specifies the level;Type
being 2 means to output the total number of testees together with their total scores in a given site. The correspondingTerm
will then be the site number;Type
being 3 means to output the total number of testees of every site for a given test date. The correspondingTerm
will then be the date, given in the same format as in the registration card.
Output Specification:
For each query, first print in a line Case #: input
, where #
is the index of the query case, starting from 1; and input
is a copy of the corresponding input query. Then output as requested:
- for a type 1 query, the output format is the same as in input, that is,
CardNumber Score
. If there is a tie of the scores, output in increasing alphabetical order of their card numbers (uniqueness of the card numbers is guaranteed); - for a type 2 query, output in the format
Nt Ns
whereNt
is the total number of testees andNs
is their total score; - for a type 3 query, output in the format
Site Nt
whereSite
is the site number andNt
is the total number of testees atSite
. The output must be in non-increasing order ofNt
's, or in increasing order of site numbers if there is a tie ofNt
.
If the result of a query is empty, simply print NA
.
Sample Input:
8 4
B123180908127 99
B102180908003 86
A112180318002 98
T107150310127 62
A107180908108 100
T123180908010 78
B112160918035 88
A107180908021 98
1 A
2 107
3 180908
2 999
Sample Output:
Case 1: 1 A
A107180908108 100
A107180908021 98
A112180318002 98
Case 2: 2 107
3 260
Case 3: 3 180908
107 2
123 2
102 1
Case 4: 2 999
NA
思路:
1、考察容器的比较器,和散列。
2、String的split(int begin,int end)方法不能截取最后部分的字符串,只能用split(int begin)方法
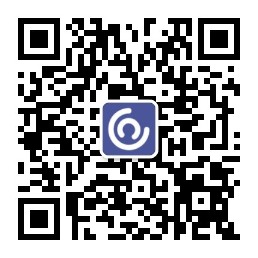
3、注意字符串和数字的转换,日期,考生编号都是6位,不要转换成数字,会丢掉前面的0位。
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.text.DecimalFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Comparator;
public class Main {
static int N;
static int M;
static Student[] stu;
public static void main(String[] args) throws IOException {
// TODO Auto-generated method stub
BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
String str = in.readLine();
String[] temp = str.split(" ");
N = Integer.parseInt(temp[0]);
stu = new Student[N];
// System.out.println(N);
M = Integer.parseInt(temp[1]);
for (int i = 0; i < N; i++) {
str = in.readLine();
String[] result = str.split("\\s+");
String level = result[0].substring(0, 1);
int site = Integer.parseInt(result[0].substring(1, 4));
String date = (result[0].substring(4, 10));
String num = result[0].substring(10);
String score = (result[1]);
stu[i] = new Student(level, site, date, num, score);
}
for (int i = 0; i < M; i++) {
str = in.readLine();
String[] st = str.split("\\s+");
int type = Integer.parseInt(st[0]);
if (type == 1)
judge1(st[1], i + 1);
else if (type == 2) {
judge2(Integer.parseInt(st[1]), i + 1);
} else
judge3((st[1]), i + 1);
}
}
private static void judge1(String string, int count) {
// TODO Auto-generated method stub
ArrayList<Student> al = new ArrayList<>();
for (Student st : stu) {
if (st.level.equals(string))
al.add(st);
}
al.sort(new Comparator<Student>() {
@Override
public int compare(Student s1, Student s2) {
if(Integer.parseInt(s1.score)>Integer.parseInt(s2.score))
return -1;
else if(Integer.parseInt(s1.score)<Integer.parseInt(s2.score)) {
return 1;
}
else
return ("" + s1.level + s1.site + s1.date + s1.num)
.compareTo("" + s2.level + s2.site + s2.date + s2.num);
// TODO Auto-generated method stub
}
});
System.out.println("Case " + count + ": 1 " + string);
if (al.size() == 0)
System.out.println("NA");
else {
for (Student st : al) {
System.out.println(st.level + st.site + st.date + st.num + " " + st.score);
}
}
}
private static void judge2(int site, int num) {
// TODO Auto-generated method stub
int testee = 0;
int sum = 0;
for (Student st : stu) {
if (st.site == site) {
testee++;
sum += Integer.parseInt(st.score);
}
}
System.out.println("Case " + num + ": 2 " + site);
if (testee != 0)
System.out.println(testee + " " + sum);
else
System.out.println("NA");
}
private static void judge3(String date, int count) {
// TODO Auto-generated method stub
Node[] nd = new Node[1000];
for (int i = 0; i < 1000; i++) {
nd[i] = new Node();
}
int flag = 0;
for (Student st : stu) {
if (st.date.equals( date)) {
int i = st.site;
nd[i].site = i;
nd[i].num++;
flag = 1;
}
}
Arrays.sort(nd, new Comparator<Node>() {
@Override
public int compare(Node nd1, Node nd2) {
// TODO Auto-generated method stub
if (nd1.num > nd2.num)
return -1;
else if (nd1.num < nd2.num)
return 1;
else {
return nd1.site - nd2.site;
}
}
});
System.out.println("Case " + count + ": 3 "+date);
if (flag == 0) {
System.out.println("NA");
} else
for (Node nd1 : nd) {
if (nd1.num != 0) {
System.out.println(nd1.site + " " + nd1.num);
}
}
}
}
class Node {
int site = 0;
int num = 0;
}
class Student {
public Student(String level, int site, String date, String num, String score) {
// TODO Auto-generated constructor stub
this.level = level;
this.site = site;
this.date = date;
this.num = num;
this.score = score;
}
String level;
int site;
String date;
String num;
String score;
}