1153 Decode Registration Card of PAT (25分)
A registration card number of PAT consists of 4 parts:
the 1st letter represents the test level, namely, T for the top level, A for advance and B for basic;
the 2nd - 4th digits are the test site number, ranged from 101 to 999;
the 5th - 10th digits give the test date, in the form of yymmdd;
finally the 11th - 13th digits are the testee's number, ranged from 000 to 999.
Now given a set of registration card numbers and the scores of the card owners, you are supposed to output the various statistics according to the given queries.
Input Specification:
Each input file contains one test case. For each case, the first line gives two positive integers N (≤104) and M (≤100), the numbers of cards and the queries, respectively.
Then N lines follow, each gives a card number and the owner's score (integer in [0,100]), separated by a space.
After the info of testees, there are M lines, each gives a query in the format Type Term, where
Type being 1 means to output all the testees on a given level, in non-increasing order of their scores. The corresponding Term will be the letter which specifies the level;
Type being 2 means to output the total number of testees together with their total scores in a given site. The corresponding Term will then be the site number;
Type being 3 means to output the total number of testees of every site for a given test date. The corresponding Term will then be the date, given in the same format as in the registration card.
Output Specification:
For each query, first print in a line Case #: input, where # is the index of the query case, starting from 1; and input is a copy of the corresponding input query. Then output as requested:
for a type 1 query, the output format is the same as in input, that is, CardNumber Score. If there is a tie of the scores, output in increasing alphabetical order of their card numbers (uniqueness of the card numbers is guaranteed);
for a type 2 query, output in the format Nt Ns where Nt is the total number of testees and Ns is their total score;
for a type 3 query, output in the format Site Nt where Site is the site number and Nt is the total number of testees at Site. The output must be in non-increasing order of Nt's, or in increasing order of site numbers if there is a tie of Nt.
If the result of a query is empty, simply print NA.
Sample Input:
8 4
B123180908127 99
B102180908003 86
A112180318002 98
T107150310127 62
A107180908108 100
T123180908010 78
B112160918035 88
A107180908021 98
1 A
2 107
3 180908
2 999
Sample Output:
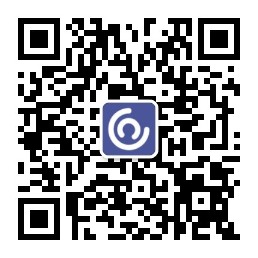
Case 1: 1 A
A107180908108 100
A107180908021 98
A112180318002 98
Case 2: 2 107
3 260
Case 3: 3 180908
107 2
123 2
102 1
Case 4: 2 999
NA
思路
模拟题,
主要是考察对sort 和 map的使用
对应的三种情况 1 将对应等级学生的信息输出
2 输出对应学生site成绩的总和
3 输出对应日期 学生site 和 site对应的数量
并且要按题意排序输出
本题要注意设计代码的时间复杂度, 测试点4改了一小时,, 注意用unordered_map(然而自己的错误点在重复做了一些无用操作)
具体超时原因 已经在超时代码中指出。
正确代码
#include<bits/stdc++.h>
using namespace std;
struct Node {
string id;
int score;
string site, data;
Node(string id, int score, string site, string data): id(id), score(score), site(site), data(data) {
}
};
struct query {
string site;
int num;
};
vector<Node> v;
bool cmpA(const Node &a, const Node &b) {
if(a.score != b.score) return a.score > b.score;
else return a.id < b.id;
}
bool cmpC(const query &a, const query &b) {
if(a.num != b.num) return a.num > b.num;
else return a.site < b.site;
}
int main()
{
int n, m;
scanf("%d%d", &n, &m);
string id;
int score;
for(int i = 0; i < n; i++) {
cin >> id >> score;
//Node node = Node(id, score, id.substr(0, 1), id.substr(1, 3), id.substr(4, 6));
v.push_back({id, score, id.substr(1, 3), id.substr(4, 6)});
}
int type;
string term;
int cnt = 1;
while(m--) {
scanf("%d", &type);
cin >> term;
vector<Node> temp;
int sum = 0;
unordered_map<string, int> ma;
int len = term.size();
for(int i = 0; i < n; i++) {
if(len == 1 && v[i].id[0] == term[0]) temp.push_back(v[i]);
else if(len == 3 && v[i].site == term) {
sum += v[i].score;
temp.push_back(v[i]);
}
else if(len == 6 && v[i].data == term) {
ma[v[i].site]++;
}
}
printf("Case %d: %d %s\n", cnt++, type, term.c_str());
if(temp.size() == 0 && type != 3 || ma.size() == 0 && type == 3) {
printf("NA\n");
continue;
}
if(type == 1) {
sort(temp.begin(), temp.end(), cmpA);
for(int i = 0; i < temp.size(); i++) {
printf("%s %d\n", temp[i].id.c_str(), temp[i].score);
}
} else if(type == 2) {
printf("%d %d\n", temp.size(), sum);
} else if(type == 3) {
//cout << ma.size() << endl;
vector<query> q;
for(auto it : ma) { //
q.push_back({it.first, it.second});
}
sort(q.begin(), q.end(), cmpC);
for(int i = 0; i < q.size(); i++) {
printf("%s %d\n", q[i].site.c_str(), q[i].num);
}
}
}
return 0;
}
错误代码(超时)
#include<bits/stdc++.h>
using namespace std;
struct Node {
string id;
int score;
};
struct query {
string site;
int num;
};
vector<Node> v;
bool cmpA(Node a, Node b) {
if(a.score != b.score) return a.score > b.score;
else return a.id < b.id;
}
bool cmpC(query a, query b) {
if(a.num != b.num) return a.num > b.num;
else return a.site < b.site;
}
int main()
{
int n, m;
scanf("%d%d", &n, &m);
string id;
int score;
for(int i = 0; i < n; i++) {
cin >> id >> score;
v.push_back({id, score});
}
int type;
string term;
int cnt = 1;
while(m--) {
scanf("%d", &type);
cin >> term;
vector<Node> temp;
int len = term.size();
for(int i = 0; i < n; i++) { // 此处是错误的位置
if(len == 1 && v[i].id[0] == term[0]) temp.push_back(v[i]);
else if(len == 3 && v[i].id.substr(1, 3) == term) temp.push_back(v[i]); // 此处就可以算出总和
else if(len == 6 && v[i].id.substr(4, 6) == term) temp.push_back(v[i]); //此处就需要将所求值加入到 unordered_map 不应该再重复计算
}
printf("Case %d: %d %s\n", cnt++, type, term.c_str());
if(temp.size() == 0) {
printf("NA\n");
continue;
}
if(type == 1) {
sort(temp.begin(), temp.end(), cmpA);
for(int i = 0; i < temp.size(); i++) {
printf("%s %d\n", temp[i].id.c_str(), temp[i].score);
}
} else if(type == 2) {
int sum = 0;
for(int i = 0; i < temp.size(); i++) {
sum += temp[i].score;
}
printf("%d %d\n", temp.size(), sum);
} else if(type == 3) {
vector<query> q;
map<string, int> ma;
for(int i = 0; i < temp.size(); i++) {
ma[temp[i].id.substr(1, 3)]++;
}
for(auto it = ma.begin(); it != ma.end(); it++) { //
q.push_back({it->first, it->second});
}
sort(q.begin(), q.end(), cmpC);
for(int i = 0; i < q.size(); i++) {
printf("%s %d\n", q[i].site.c_str(), q[i].num);
}
}
}
return 0;
}