法一:深度优先遍历,发现环
class Solution {
typedef struct GraphNode{
int val;
vector<GraphNode* > Neighbors;
int label;
}GraphNode;
public:
void judgeCircle(GraphNode* graph, vector<int>& visit,bool& circleMark){
if(!graph) return;
if(!circleMark) return;
if((graph->Neighbors).size() == 0) {
visit[graph->label] = 1;
return;
}
for(int i=0; i< (graph->Neighbors).size(); i++){
if( visit[(graph->Neighbors)[i]->label] == -1){
visit[(graph->Neighbors)[i]->label] = 0;
judgeCircle((graph->Neighbors)[i], visit, circleMark);
visit[(graph->Neighbors)[i]->label] = 1;
}
else if(visit[(graph->Neighbors)[i]->label] == 0){
circleMark = false;
return;
}
visit[(graph->Neighbors)[i]->label] = 1;
}
}
bool canFinish(int numCourses, vector<vector<int>>& prerequisites) {
vector<GraphNode*> graph;
vector<int> visit;
bool circleMark = true;
for(int i = 0; i< numCourses; i++){
GraphNode* p = new GraphNode;
p->label = i;
graph.push_back(p);
visit.push_back(-1);
}
for(int i = 0; i< prerequisites.size(); i++){
graph[prerequisites[i][0]]->label = prerequisites[i][0];
graph[prerequisites[i][0]]->Neighbors.push_back(graph[prerequisites[i][1]]) ;
}
for(int i = 0; i< graph.size(); i++){
visit[graph[i]->label] = 0;
judgeCircle(graph[i], visit, circleMark);
visit[graph[i]->label] = 1;
if(!circleMark) return false;
}return true;
}
};
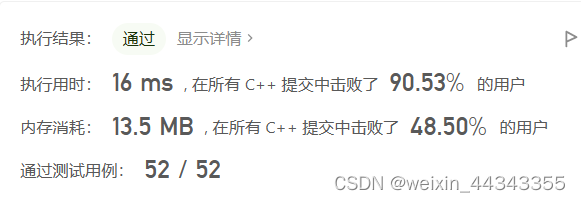
法二:利用拓扑排序,遍历所有入度为0的点,看是否能遍历完所有的点
class Solution {
typedef struct GraphNode{
int val;
vector<GraphNode* > Neighbors;
int label;
}GraphNode;
public:
bool canFinish(int numCourses, vector<vector<int>>& prerequisites) {
vector<GraphNode*> graph;
vector<int> depth;
queue<GraphNode*> visit;
for(int i = 0; i<numCourses; i++){
GraphNode* p = new GraphNode;
p->label = i;
graph.push_back(p);
depth.push_back(0);
}
for(int i = 0; i< prerequisites.size(); i++){
graph[prerequisites[i][1]]->Neighbors.push_back(graph[prerequisites[i][0]]);
depth[prerequisites[i][0]]++;
}
while(true){
for(int i = 0; i< numCourses; i++){
if(depth[i]==0){
visit.push(graph[i]);
}
}
if(visit.size() == 0) {
break;
}
while(visit.size()){
for(int i = 0; i< visit.front()->Neighbors.size(); i++){
depth[visit.front()->Neighbors[i]->label] --;
}
depth[visit.front()->label] = -1;
visit.pop();
}
}
for(int i = 0; i< numCourses; i++){
if(depth[i]>0){
return false;
}
}
return true;
}
};
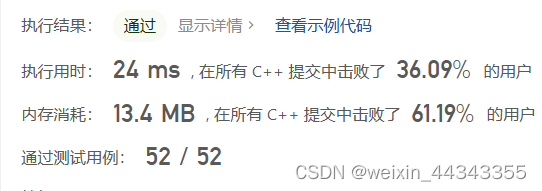