版权声明:本文为博主原创文章,未经博主允许不得转载。有事联系:[email protected] https://blog.csdn.net/qq_17550379/article/details/89325779
给定二叉树的根节点 root
,找出存在于不同节点 A
和 B
之间的最大值 V
,其中 V = |A.val - B.val|
,且 A
是 B
的祖先。
(如果 A 的任何子节点之一为 B,或者 A 的任何子节点是 B 的祖先,那么我们认为 A 是 B 的祖先)
示例:

输入:[8,3,10,1,6,null,14,null,null,4,7,13]
输出:7
解释:
我们有大量的节点与其祖先的差值,其中一些如下:
|8 - 3| = 5
|3 - 7| = 4
|8 - 1| = 7
|10 - 13| = 3
在所有可能的差值中,最大值 7 由 |8 - 1| = 7 得出。
提示:
- 树中的节点数在
2
到5000
之间。 - 每个节点的值介于
0
到100000
之间。
解题思路
首先不难想到暴力法,也就是从每个节点出发向其子节点寻找最大差值。
class Solution:
def maxAncestorDiff(self, root: TreeNode) -> int:
if not root:
return 0
self.res = 0
self.helper(root, root.val)
return max(self.res, self.maxAncestorDiff(root.left), self.maxAncestorDiff(root.right))
def helper(self, node, val):
s = [node]
while s:
t = s.pop()
self.res = max(self.res, abs(t.val - val))
if t.right:
s.append(t.right)
if t.left:
s.append(t.left)
但是这种做法非常慢。有没有更快的解法呢?实际上这个问题就是找树的每条路径中的最小值和最大值。找树的路径怎么去做?这就是之前问题Leetcode 257:二叉树的所有路径(最详细的解法!!!),但是我们并不需要将所有的路径多找出来。我们只需要定义两个变量mx
和mn
分别表示路径中的最大值和最小值,然后深度遍历整棵树的过程中更新值即可。最后结果就是最大值减去最小值。
class Solution:
def maxAncestorDiff(self, root: TreeNode, mn=float('inf'), mx=0) -> int:
return max(self.maxAncestorDiff(root.left, min(mn, root.val), max(mx, root.val)), \
self.maxAncestorDiff(root.right, min(mn, root.val), max(mx, root.val))) \
if root else mx - mn
reference:
我将该问题的其他语言版本添加到了我的GitHub Leetcode
如有问题,希望大家指出!!!
扫描二维码关注公众号,回复:
5955114 查看本文章
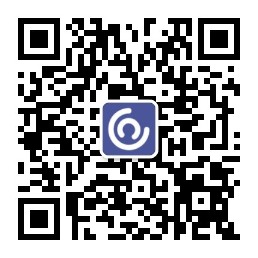