PAT A1009 Product of Polynomials
题目描述:
This time, you are supposed to find A×B where A and B are two polynomials.
Input Specification:
Each input file contains one test case. Each case occupies 2 lines, and each line contains the information of a polynomial:
K N1 aN1 N2 aN2 ... NK aNK
where K is the number of nonzero terms in the polynomial, Ni and aNi (i=1,2,⋯,K) are the exponents and coefficients, respectively. It is given that 1≤K≤10, 0≤NK<⋯<N2<N1≤1000.
Output Specification:
For each test case you should output the product of A and B in one line, with the same format as the input. Notice that there must be NO extra space at the end of each line. Please be accurate up to 1 decimal place.
Sample Input:
2 1 2.4 0 3.2
2 2 1.5 1 0.5
Sample Output:
3 3 3.6 2 6.0 1 1.6
参考代码:
参考1:
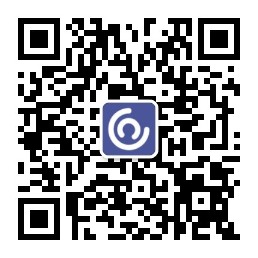
1 /**************************************************** 2 PAT A1009 Product of Polynomials 3 ****************************************************/ 4 #include <iostream> 5 #include <algorithm> 6 #include <iomanip> 7 #include <vector> 8 #include <cmath> 9 10 using namespace std; 11 12 struct Term { 13 int Exponent = 0; 14 double Coefficient = 0.0; 15 }; 16 17 bool cmp(Term A, Term B) { 18 return A.Exponent > B.Exponent; 19 } 20 21 //整理得到的方程 22 void ArrangePoly(vector<Term>& poly) { 23 //合并指数相同项 24 for (vector<Term>::iterator itr = poly.begin() + 1; itr != poly.end(); ++itr) { 25 if (itr->Exponent == (itr - 1)->Exponent) { 26 (itr - 1)->Coefficient += itr->Coefficient; 27 itr = poly.erase(itr); 28 itr--; 29 } 30 } 31 32 //移除系数绝对值小于0.1(包括0)项 33 for (vector<Term>::iterator itr = poly.begin(); itr != poly.end(); ++itr) { 34 if (itr->Coefficient == 0 || fabs(itr->Coefficient) < 0.1) { 35 itr = poly.erase(itr); 36 itr--; 37 } 38 } 39 } 40 41 //计算两个方程相乘之后的新方程 42 vector<Term> GetPolynomial(const vector<Term> poly1, const vector<Term> poly2) { 43 Term temp; 44 vector<Term> poly3; 45 46 for (int i = 0; i < poly1.size(); ++i) { 47 for (int j = 0; j < poly2.size(); ++j) { 48 temp.Exponent = poly1[i].Exponent + poly2[j].Exponent; 49 temp.Coefficient = poly1[i].Coefficient * poly2[j].Coefficient; 50 51 poly3.push_back(temp); 52 } 53 } 54 55 //结果按照指数从大到小的顺序排列,以便后续的处理 56 sort(poly3.begin(), poly3.end(), cmp); 57 ArrangePoly(poly3); 58 59 return poly3; 60 } 61 62 int main() { 63 int N1 = 0, N2 = 0; 64 65 cin >> N1; 66 vector<Term> func1(N1); 67 for (int i = 0; i < N1; ++i) 68 cin >> func1[i].Exponent >> func1[i].Coefficient; 69 70 cin >> N2; 71 vector<Term> func2(N2); 72 for (int i = 0; i < N2; ++i) 73 cin >> func2[i].Exponent >> func2[i].Coefficient; 74 75 vector<Term> func3(GetPolynomial(func1, func2)); 76 77 func3.size() == 0 ? cout << '0' : cout << func3.size() << ' '; 78 for (int i = 0; i < func3.size(); ++i) { 79 cout << setiosflags(ios::fixed) << setprecision(0) << func3[i].Exponent << ' '; 80 cout << setiosflags(ios::fixed) << setprecision(1) << func3[i].Coefficient; 81 if (i != func3.size() - 1) cout << ' '; 82 } 83 84 return 0; 85 }