题目链接:https://leetcode.com/problems/palindrome-partitioning-ii/
Given a string s, partition s such that every substring of the partition is a palindrome.
Return the minimum cuts needed for a palindrome partitioning of s.
Example:
Input: "aab" Output: 1 Explanation: The palindrome partitioning ["aa","b"] could be produced using 1 cut.
思路:用DP
设DP[i][j]表示第i个字符到第j个字符是否构成回文,若是,则DP[i][j]=true;若否,则DP[i][j]=false;
那么DP[i][j]构成回文需满足:
1、输入字符串s[i]==s[j];
2、条件1满足并不能保证i到j构成回文,还须:(j-i)<=1或者DP[i+1][j-1]=1;
即,i、j相邻或者i=j,也就是相邻字符相等构成回文或者字符自身构成回文,如果i、j不相邻或者相等,i到j构成回文的前提就是DP[i+1][j-1]=true。
所以状态转移方程为:s.charAt(i)==s.charAt(j)&&(j-i<=1||dp[i+1][j-1])
扫描二维码关注公众号,回复:
5884004 查看本文章
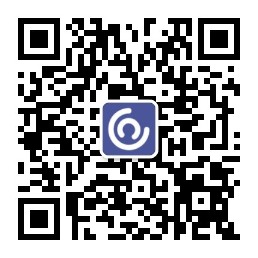
AC 6ms Java:
class Solution {
public int minCut(String s) {
boolean[][] dp=new boolean[s.length()][s.length()];
int[] cut=new int[s.length()];
for(int j=0;j<s.length();j++){
int min=j;//这里设定一个最大值,比如共有两个字符(下标分别为0,1),那么最多只cut 1 次。
for(int i=0;i<=j;i++){
if(s.charAt(i)==s.charAt(j)&&(j-i<=1||dp[i+1][j-1])){
dp[i][j]=true;
min=i==0?0:Math.min(cut[i-1]+1,min);
}
}
cut[j]=min;
}
return cut[s.length()-1];
}
}