格雷编码是一个二进制数字系统,在该系统中,两个连续的数值仅有一个位数的差异。
给定一个代表编码总位数的非负整数 n,打印其格雷编码序列。格雷编码序列必须以 0 开头。
示例 1:
输入: 2 输出:[0,1,3,2]
解释: 00 - 0 01 - 1 11 - 3 10 - 2 对于给定的 n,其格雷编码序列并不唯一。 例如,[0,2,3,1]
也是一个有效的格雷编码序列。 00 - 0 10 - 2 11 - 3 01 - 1
示例 2:
输入: 0 输出:[0] 解释: 我们定义
格雷编码序列必须以 0 开头。给定
编码总位数为n 的格雷编码序列,其长度为 2n
。当 n = 0 时,长度为 20 = 1。 因此,当 n = 0 时,其格雷编码序列为 [0]。
思路: n=0,0 n=1, 0 1
n=2,00
01
11
10
n=3,000
001
扫描二维码关注公众号,回复:
4167675 查看本文章
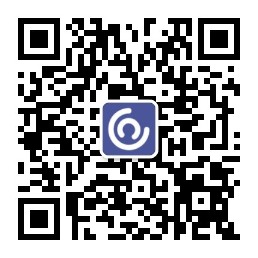
011
010
110
111
101
100
可以发现,n+1时,只要把数字翻转过来,最高位置为1即可满足条件,代码如下:
C
/**
* Return an array of size *returnSize.
* Note: The returned array must be malloced, assume caller calls free().
*/
int* grayCode(int n, int* returnSize)
{
int count=pow(2,n);
int* res=(int*)malloc(sizeof(int)*count);
res[0]=0;
int k=1;
if(n>0)
{
res[1]=1;
k=2;
int i=2;
while(i<=n)
{
int m=k;
int cc=pow(2,i-1);
for(int j=0;j<m;j++)
{
res[k++]=cc+res[m-1-j];
}
i++;
}
}
*returnSize=k;
return res;
}
C++
class Solution {
public:
vector<int> grayCode(int n)
{
vector<int> res;
if(0==n)
{
res.push_back(0);
}
else
{
res.push_back(0);
res.push_back(1);
int i=2;
while(i<=n)
{
int m=res.size();
int cc=pow(2,i-1);
for(int j=0;j<m;j++)
{
res.push_back(cc+res[m-1-j]);
}
i++;
}
}
return res;
}
};
python
class Solution:
def grayCode(self, n):
"""
:type n: int
:rtype: List[int]
"""
res=[0]
if n>0:
res.append(1)
i=2
while i<=n:
m=len(res)
cc=2**(i-1)
for j in range(m):
res.append(cc+res[m-1-j])
i+=1
return res