版权声明:本文为博主原创文章,转载请注明出处。 https://blog.csdn.net/u012898245/article/details/80866478
数据结构之数组
面试题3:在一个二维数组中,每一行都按照从左到右递增的顺序排序,每一列都按照从上到下递增的顺序排序。请完成一个函数,输入这样的一个二维数组和一个整数,判断数组中是否含有该整数。
二维数组中的元素行列分别递增,就像这样:
{{1, 2, 3, 9},
{5, 6, 7, 10},
{6, 8, 9, 12},
{8, 13, 14, 15}}
1.代码实现:
package com.practice;
public class SearchTwoDimensionalArray {
/**
* 在二维数组中查找指定元素
*
* @param array 二维数组
* @return boolean
*/
public static boolean searchTarget(int[][] array, int target) {
if (array == null || array.length < 1) {
return false;
}
// 获取二维数组的长度
int rows = array.length;
int columns = array[rows - 1].length;
// 判断是否在二维数组里面
if (target < array[0][0] || target > array[rows - 1][columns - 1]) {
return false;
}
// 从右到左
int row = 0;
int column = columns - 1;
while (row < rows && column >= 0) {
if (array[row][column] == target) {
return true;
}
if (array[row][column] > target) {
column--;
} else {
row++;
}
}
return false;
}
public static void main(String args[]) {
int[][] array = {{1, 2, 3, 4}, {5, 6, 7, 8}, {10, 11, 12, 13}, {15, 16, 17, 18}};
System.out.println(searchTarget(array, 18));
}
}
2 题目解析:
前提:二维数组行列的数据分别都是递增的
输入:以上面的数组为输入,查找数组中是否存在整数8
从右上角开始判断,右上角的元素是第一行最大的,同时是最后一列最小的元素,右上角的元素是9
9>8,所以9所在的那一列都是大于8的,去掉最后一列;
扫描二维码关注公众号,回复:
3042934 查看本文章
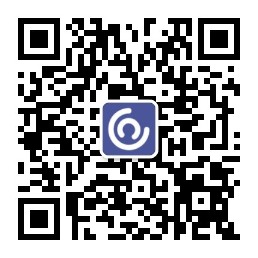
剩下的从第一行倒数第二列开始比对,元素是3<8,当前行的所有元素都小于8了,删掉;
从第二行,倒数第二列的右上角开始,取7<8,当前行的所有元素都是小于8的,删掉;
从第三行,倒数第二列开始,取9>8,当前列的所有元素都是大于8的,删掉;
从第三行,倒数第三列右上角开始,取8=8,循环结束,返回true;
对,就这个思路。