Stars
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 54352 | Accepted: 23386 |
Description
Astronomers often examine star maps where stars are represented by points on a plane and each star has Cartesian coordinates. Let the level of a star be an amount of the stars that are not higher and not to the right of the given star. Astronomers want to know the distribution of the levels of the stars.
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
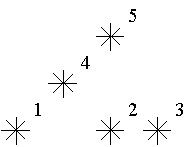
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
Input
The first line of the input file contains a number of stars N (1<=N<=15000). The following N lines describe coordinates of stars (two integers X and Y per line separated by a space, 0<=X,Y<=32000). There can be only one star at one point of the plane. Stars are listed in ascending order of Y coordinate. Stars with equal Y coordinates are listed in ascending order of X coordinate.
Output
The output should contain N lines, one number per line. The first line contains amount of stars of the level 0, the second does amount of stars of the level 1 and so on, the last line contains amount of stars of the level N-1.
Sample Input
5 1 1 5 1 7 1 3 3 5 5
Sample Output
1 2 1 1 0
Hint
This problem has huge input data,use scanf() instead of cin to read data to avoid time limit exceed.
题目意思:
给你n给点的star的坐标,star左下角star的个数是star的等级(包括左下边界,即x相同或者y相同
最好输出等级1到等级n的star个数
给你n给点的star的坐标,star左下角star的个数是star的等级(包括左下边界,即x相同或者y相同
最好输出等级1到等级n的star个数
分析:树状树主要用来解决区间问题
所以这题可以用树状数组写
所以这题可以用树状数组写
一开始给出的数据是已经按照y升序排序好了的,如果y相同则按照x升序排序
所以统计某个等级的个数就是统计x前面比它小的星星的数量
但是有需要注意的地方
树状数组是不能统计0的
所以每个x都要++
这也也不会影响统计的结果
所以统计某个等级的个数就是统计x前面比它小的星星的数量
但是有需要注意的地方
树状数组是不能统计0的
所以每个x都要++
这也也不会影响统计的结果
code:
#include<queue> #include<set> #include<cstdio> #include <iostream> #include<algorithm> #include<cstring> #include<cmath> using namespace std; #define max_v 320010 int a[max_v]; int c[max_v]; //C数组C[i] = a[i – 2^k + 1] + … + a[i] ,k为二进制下某尾0的个数。 int lowbit(int x)//返回2的k次方的值 { return x&(-x); } void update(int x,int d)//更新树状数组 { while(x<max_v) { c[x]=c[x]+d; x=x+lowbit(x); } } int getsum(int x)//就是把C数组全部加起来 { int res=0; while(x>0) { res=res+c[x]; x=x-lowbit(x); } return res; } int main() { int n,x,y; while(~scanf("%d",&n)) { memset(c,0,sizeof(c)); memset(a,0,sizeof(a)); for(int i=0;i<n;i++) { scanf("%d %d",&x,&y);//下标可能从0开始,所以要x+1 a[getsum(x+1)]++;//求出其左下角star个数 update(x+1,1);//更新树状数组 } for(int i=0;i<n;i++) { printf("%d\n",a[i]); } } return 0; } /* 题目意思: 给你n给点的star的坐标,star左下角star的个数是star的等级(包括左下边界,即x相同或者y相同 最好输出等级1到等级n的star个数 分析:树状树主要用来解决区间问题 所以这题可以用树状数组写 一开始给出的数据是已经按照y升序排序好了的,如果y相同则按照x升序排序 所以统计某个等级的个数就是统计x前面比它小的星星的数量 但是有需要注意的地方 树状数组是不能统计0的 所以每个x都要++ 这也也不会影响统计的结果 */