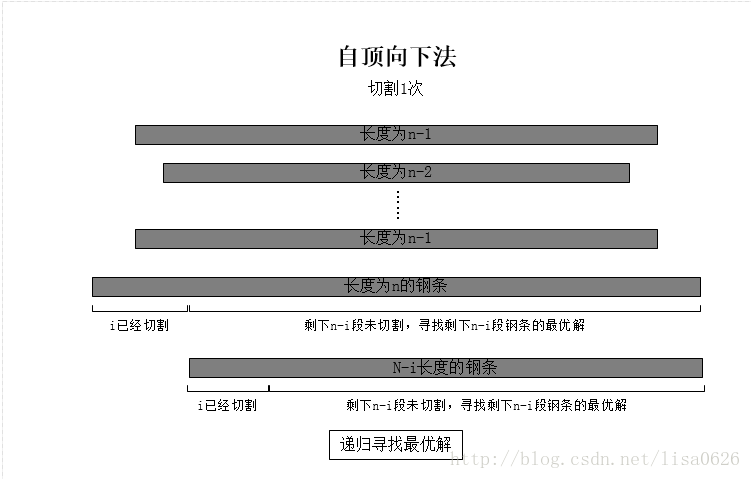
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 带备忘的自顶向下
{
class Program
{
static void Main(string[] args)
{
int[] result = new int[11];
int[] price = { 0, 1, 5, 8, 9, 10, 17, 17, 20, 24, 30 };//索引为钢条长度
for (int i = 1; i <= 10; i++)
{
Console.WriteLine(UpDown(i, price,result));
}
}
public static int UpDown(int n, int[] p,int[] result) //返回值为最优解
{
if (n == 0) return 0;//不能分割时直接返回0
if (result[n] != 0)
{
return result[n];//最优解已经求出的时候不再重新求,直接取值
}
int temp = 0;
for (int i = 1; i <= n; i++)
{
int maxPrice = p[i] + UpDown(n - i, p,result);//递归调用,求出n-i的最优解
if (maxPrice > temp)
{
temp = maxPrice;
}
}
result[n] = temp;//保存最优解
return temp;
}
}
}
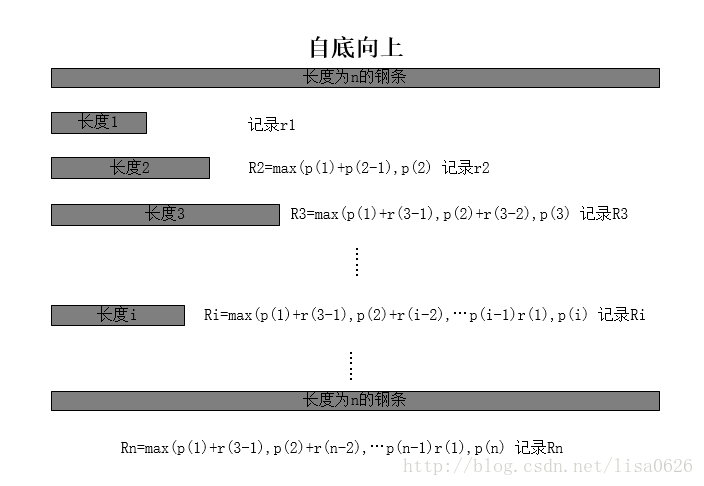
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 自底向上法
{
class Program
{
static void Main(string[] args)
{
int[] result = new int[11];
int[] price = { 0, 1, 5, 8, 9, 10, 17, 17, 20, 24, 30 };//索引为钢条长度
for (int i = 1; i <= 10; i++)
{
Console.WriteLine(BottomUp(i, price, result));
}
Console.ReadKey();
}
public static int BottomUp(int n, int[] p, int[] result)
{
for (int i = 1; i <= n; i++)//第一次循环 i=1 第二次循环 i=2
{
int temp = 0;
for (int j = 1; j <= i; j++)
{//第一次循环 j=1 第二次循环 j=1
int maxPrice = p[j] + result[i - j];//第一次循环 i-j=0 result[0]=0
if (maxPrice > temp) {
temp = maxPrice;//第一次循环 maxprice=1;
}
}
result[i] = temp;//第一次循环 result[1]=1
}
return result[n];
}
}
}