Directional Light:平行光源/方向性光源,用来模拟太阳光(角度只与旋转角度有关,与位置无关)
Point Light:点光源,用来模拟灯泡,向四周发散光源
Spotlight:锥光源/聚光灯,用来模拟手电筒,灯塔等光源,向一个方向扩散性发散光源
Area Light:区域光,用于烘焙光照贴图,上面这三种光源可以用于实时渲染,也可以用于烘焙光照贴图
通过代码控制光源旋转、颜色、强度,可以做出24h光线变幻,日月轮转
----Intensity光线强度,默认1,关闭0
----Shadow Type阴影类型,柔性阴影更耗资源
----Intensity Multiplier反射光
----Spot Angle锥光源的角度
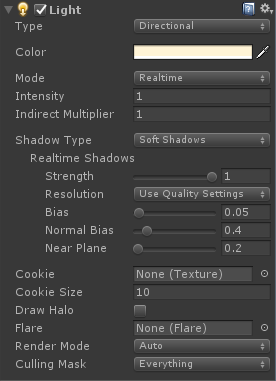
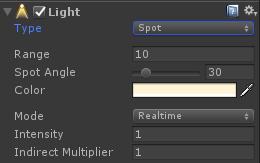
灯光控制
LightType是枚举类型
using System.Collections; using System.Collections.Generic; using UnityEngine; public class MyLight : MonoBehaviour { // Use this for initialization void Start() { Light light = GetComponent<Light>(); light.intensity = 1; //int[] array = new int[] { 1, 2, 3, 4, 5, 6 }; if (light != null) { Debug.Log(light.intensity); } } // Update is called once per frame void Update() { } }
父子物体:
在层级视图中,将一个游戏物体拖拽到另一个游戏物体下面,就变成了父子物体关系。
特点:子物体动,父物体不动;父物体动,子物体跟随移动。
作用:拼接游戏物体。
Center:父子物体的几何中心
Pivot:选中物体的几何中心

注意:当形成父子物体关系后,在检视面板中(在代码中不同)子物体的Transform下的属性是相对于父物体的值。比如position就是相对于父物体坐标为坐标原点的相对坐标。
当游戏物体没有父物体,世界就是父物体,这个时候坐标就是相对于世界的坐标位置。
在C#代码中所有物体都是世界坐标。
两个游戏物体的关联,本质上是把两个两个游戏物体Transform的关联在一起。
建立父子关系
API:Application Programer Interface //应用程序编程接口,拿到Unity来说,有一些需要用到的功能,unity已经帮你写好了,直接拿来用就可以得到想要的结果,不需要自己重新写,而且也不必须知道到底是怎么实现的。
查找游戏物体:
GameObject.Find(string name);//通过游戏物体的名字来查找游戏物体的引用。但是,正常开发过程中,尤其是在频繁的查找过程中,不建议使用Find。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Find_Parent : MonoBehaviour { public GameObject sphere; // Use this for initialization void Start() { //如何在整个场景中查找游戏物体 //查找写成静态方法,是工具,不是某一个对象的方法 sphere = GameObject.Find("Find_Parent/Sphere"); Debug.Log(sphere.name); //建立父子关系,需要关联transform gameObject.transform.parent = sphere.transform; } // Update is called once per frame void Update() { //不要在Update进行查找,GameObject.Find("Sphere"); //要建一个全局的sphere初始化 //移动父物体,子物体也会动 if (sphere) { sphere.transform.Translate(new Vector3(0, 0, 0.1f)); // //或者 // sphere.transform.Translate(Vector3.forward * 0.1f); } } }
GetComponent推荐使用泛型的重载
1、用代码获取组件
T:GetComponent<T>()//如果获取Transform,就可以直接写Transform,是Component类中的一个只读的成员属性,代表着当前这个组件对象所挂载的游戏物体身上的Transform组件的引用。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class GetComponent : MonoBehaviour { // Use this for initialization void Start () { Transform trans = GetComponent<Transform>(); //GetComponent获取组件的方法,加()表执行 //GetComponent获取不到组件,会返回空引用变量(空指针),在使用的时候,会报空引用异常的错误 Transform trans = null; Debug.Log(trans.position); if (trans != null) { Debug.Log(trans.position); } //transform transform.position = new Vector3(1, 2, 3);//不能只修改transform.position某个分量的值,需要对transform.position整体重新赋值,才会有返回值 Debug.Log(transform.position); } // Update is called once per frame void Update () { } }
2、用代码动态添加组件
gameObject可以直接在继承自component的类中使用,代表着当前组件所挂载的游戏物体的引用。
T:gameObject.AddComponent<T>() 当完成添加组件的任务之后,会返回这个组件的引用。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class AddComponent : MonoBehaviour { // Use this for initialization void Start () { Light light = gameObject.AddComponent<Light>(); light.intensity = 1; light.type = LightType.Spot; light.color = Color.green; Destroy(light, 5.0f); } // Update is called once per frame void Update () { } }
3、用代码动态移除组件
Destroy(Object obj);
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Destroy : MonoBehaviour { // Use this for initialization void Start () { AudioSource audio = GetComponent<AudioSource>(); if (audio)//audio是传进来的引用 { Destroy(audio); //5秒后销毁audio引用 Destroy(audio, 5); //5秒后销毁Lesson3脚本组件 Destroy(this, 5); } } // Update is called once per frame void Update () { } }
平移:transform.Translate(Vector3 translation);
transform.Translate (vector.forward) :移动的是自身坐标系的方向
transform.position += vector.forward:移动世界坐标系的方向
向量 * 标量,控制速度
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Move : MonoBehaviour { public float moveSpeed = 0.5f; // Use this for initialization void Start() { // transform.position = new Vector3(3.0f, 3.0f, 3.0f);//不要放在循环里用,调用一次就够了 } // Update is called once per frame void Update() { if (transform.position.z < 10.0f) { transform.Translate(Vector3.forward * moveSpeed * Time.deltaTime); } else { transform.position = new Vector3(3.0f, 3.0f, 3.0f); } //每帧z轴都移动0.1f,当前坐标加上新坐标,再返回给原坐标 transform.position += new Vector3(0, 0, 0.1f); //Translate平移 transform.Translate(new Vector3(0, 0, 0.1f)); //自定义myTranslate myTranslate(new Vector3(0, 0, 0.1f)); } void myTranslate(Vector3 translation) { transform.position += new Vector3(0, 0, 0.1f); } }
平移控制
using System.Collections; using System.Collections.Generic; using UnityEngine; public class MoveControl : MonoBehaviour { private float moveSpeed = 2; // Use this for initialization void Start() { } // Update is called once per frame void Update() { if (Input.GetKey(KeyCode.W)) { this.transform.Translate(Vector3.forward * Time.deltaTime * moveSpeed); } if (Input.GetKey(KeyCode.S)) { this.transform.Translate(Vector3.back * Time.deltaTime * moveSpeed); } if (Input.GetKey(KeyCode.A)) { this.transform.Translate(Vector3.left * Time.deltaTime * moveSpeed); } if (Input.GetKey(KeyCode.D)) { this.transform.Translate(Vector3.right * Time.deltaTime * moveSpeed); } } }
旋转:transform.Rotate(Vector3 eulerAngles);
transform.Rotate (vector.up) :绕着自身坐标系的方向旋转
transform.eulerAngles += vector.up:绕着世界坐标系的方向旋转
transform.eulerAngles = new Vector3(90,0,0);
using System.Collections; using System.Collections.Generic; using UnityEngine; public class position_eulerAngles : MonoBehaviour { // Use this for initialization void Start () { } // Update is called once per frame void Update () { transform.position += Vector3.back * 0.05f; transform.eulerAngles += Vector3.up; } }
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Rotate : MonoBehaviour { // Use this for initialization void Start() { } // Update is called once per frame void Update() { //旋转 //欧拉角 transform.eulerAngles += new Vector3(0, 0, 0.1f); transform.eulerAngles += Vector3.up; //旋转的API是Rotate transform.Rotate(Vector3.up); } }
为什么要继承MonoBehaviour
MonoBehaviour继承于Behaviour
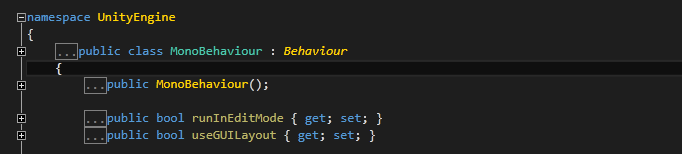
Behaviour继承于Component,Component是所有组件的基类,enabled:组件是否激活
Object是Component的基类,组件的本质是Object类
GetComponent获取不到组件,会返回空引用变量(空指针),在使用的时候,会报空引用异常的错误
Unity复习
cookie灯光遮罩效果(平行光源,点光源,椎光源)
jpg图片没有Alpha通道,需要从灰度生成Alpha通道,最好用png图片
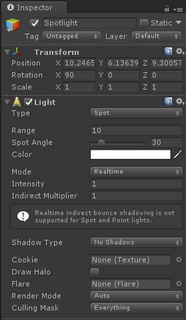
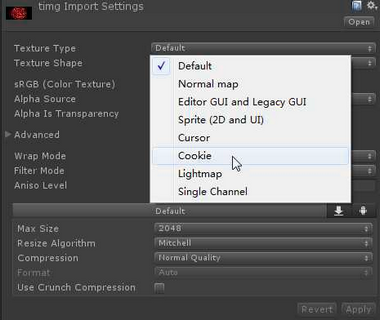
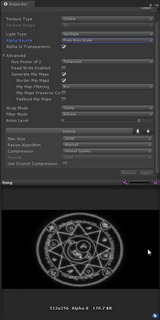
点光源,立方体贴图(六个面可以不一样),cookie
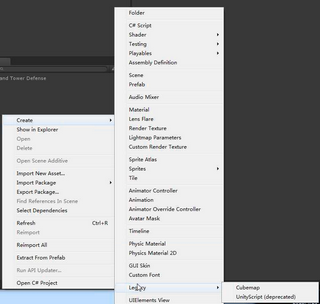
Halo光晕
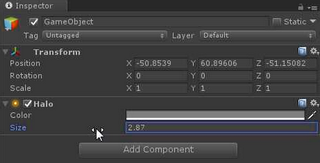
Layer:给游戏物体添加层
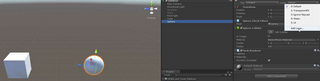
Culing Mask遮罩剔除:光源对那些层(游戏物体)起作用

子物体从父物体拖出,会叠加上父物体的Transform,Position和Rotation做加法,Scale做乘法

F12进入定义,a是alpha通道
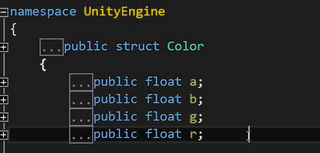
拖拽游戏物体到脚本组件,可以自动赋值
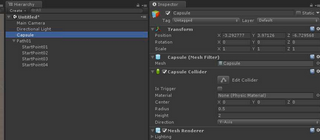
添加路径路点,“/”代表搜索路径,加上路径可以减少搜索次数
implicit:隐式转换
operator重载:if(exists != null) 可以简写为 if(exists)