版权声明:本文为博主原创文章,未经博主允许不得转载,不得用于商业用途。 https://blog.csdn.net/WDAJSNHC/article/details/82392002
codeup1779 堆排序
时空限制 1000ms/128MB
题目描述
堆排序是一种利用堆结构进行排序的方法,它只需要一个记录大小的辅助空间,每个待排序的记录仅需要占用一个存储空间。
首先建立小根堆或大根堆,然后通过利用堆的性质即堆顶的元素是最小或最大值,从而依次得出每一个元素的位置。
堆排序的算法可以描述如下:
在本题中,读入一串整数,将其使用以上描述的堆排序的方法从小到大排序,并输出。
输入
输入的第一行包含1个正整数n,表示共有n个整数需要参与排序。其中n不超过100000。
第二行包含n个用空格隔开的正整数,表示n个需要排序的整数。
输出
只有1行,包含n个整数,表示从小到大排序完毕的所有整数。
请在每个整数后输出一个空格,并请注意行尾输出换行。
样例输入
10
2 8 4 6 1 10 7 3 5 9
样例输出
1 2 3 4 5 6 7 8 9 10
提示
在本题中,需要按照题目描述中的算法完成堆排序的算法。
扫描二维码关注公众号,回复:
3084059 查看本文章
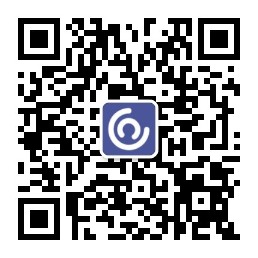
堆排序对于元素数较多的情况是非常有效的。通过对算法的分析,不难发现在建立含有n个元素的堆时,总共进行的关键字比较次数不会超过4n,且n个节点的堆深度是log2n数量级的。因此,堆排序在最坏情况下的时间复杂度是O(nlog2n),相对于快速排序,堆排序具有同样的时间复杂度级别,但是其不会退化。堆排序较快速排序的劣势是其常数相对较大。
代码
法一:二叉排序树
#include<iostream>
using namespace std;
struct node{
int data;
node *lch,*rch;
};
int n,cnt=0;
void insert(node *&root,int x){
if (root==NULL){
root = new node;
root->data = x;
root->lch = root->rch = NULL;
return;
}
if (x<root->data) insert(root->lch,x); //不超过父节点,插入左子树
else insert(root->rch,x); //否则,插入右子树
}
void create(node *&root){
cin>>n;
for (int i=1,x; i<=n; i++){
cin>>x;
insert(root,x);
}
}
void midord(node *root){ //中序遍历
if (root){
midord(root->lch);
if (++cnt<n) cout<<root->data<<" ";
else cout<<root->data<<endl;
midord(root->rch);
}
}
int main(){
ios::sync_with_stdio(false);
node *root(NULL);
create(root);
midord(root);
return 0;
}
法二:STL优先队列
#include<iostream>
#include<queue>
using namespace std;
priority_queue<int,vector<int>,greater<int> > pq; //小顶堆
int main(){
ios::sync_with_stdio(false);
int n;
cin>>n;
for (int i=1,x; i<=n; i++){
cin>>x;
pq.push(x);
}
for (int i=1; i<n; i++){
cout<<pq.top()<<" ";
pq.pop();
}
cout<<pq.top()<<endl;
return 0;
}
法三:手动堆
#include<iostream>
#include<algorithm>
using namespace std;
const int N = 100005;
int n,a[N];
void adjust(int i,int m){ //调整为大顶堆
for (int j=2*i; j<=m; ){
if (j<m && a[j+1]>a[j]) j++; //存在右孩子且值更大
if (a[i]<a[j]){ //a[i]父亲节点 a[j]孩子节点
swap(a[i],a[j]);
i = j;
j = 2*i;
}
else break;
}
}
void heapsort(int n){
for (int i=n/2; i>=1; i--) adjust(i,n); //调整内部节点
for (int i=n; i>1; i--){
swap(a[1],a[i]);
adjust(1,i-1);
}
}
int main(){
ios::sync_with_stdio(false);
cin>>n;
for (int i=1; i<=n; i++) cin>>a[i];
heapsort(n);
for (int i=1; i<=n; i++)
i<n ? cout<<a[i]<<" " : cout<<a[i]<<endl;
return 0;
}