什么是点云连接
点云连接指的是将两个或多个点云数据合并在一起,形成一个更大的点云数据集。在这个过程中,点云数据根据它们的坐标位置被放置在一起。具体而言,点云连接操作将两个点云的点按顺序合并到一个新的点云对象中。最终,新的点云对象将包含两个点云的所有点,并且点的顺序保持不变。这样做可以将多个点云数据组合在一起,以形成大型、更复杂的点云场景。
pcl::concatenate
或pcl::concatenatePoints
函数用于将多个点云数据对象的点连接在一起,形成一个新的点云数据对象。这个函数实际上是点云数据对象的拼接或连接操作,它会改变点云的数量和位置。
什么是点云字段连接
pcl::concatenateFields
函数用于将多个点云数据对象的字段(属性)连接在一起,例如将 XYZ 坐标、RGB 颜色、法线等字段组合成一个新的点云数据对象。这个函数可以将不同点云数据对象中的不同字段合并成一个更大的点云数据对象,但不会改变点云的数量和位置。
点云连接代码
#include <pcl/point_cloud.h>
#include <pcl/point_types.h>
#include <pcl/visualization/pcl_visualizer.h>
void generateRandomPointCloud(pcl::PointCloud<pcl::PointXYZ>::Ptr cloud, int num_points){
srand(time(0));
cloud->resize(num_points);
for(int i=0; i < num_points; ++i){
cloud->points[i].x = 1024 * rand() / (RAND_MAX + 1.0f);
cloud->points[i].y = 1024 * rand() / (RAND_MAX + 1.0f);
cloud->points[i].z = 1024 * rand() / (RAND_MAX + 1.0f);
}
}
int main()
{
// 定义两个点云对象
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud1(new pcl::PointCloud<pcl::PointXYZ>());
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud2(new pcl::PointCloud<pcl::PointXYZ>());
// 生成对及点云数据
int num_points = 30;
generateRandomPointCloud(cloud1, num_points);
generateRandomPointCloud(cloud2, num_points);
// 进行点云连接
pcl::PointCloud<pcl::PointXYZ>::Ptr combined(new pcl::PointCloud<pcl::PointXYZ>()); // 用于存储连接结果
pcl::concatenate(*cloud1, *cloud2,*combined);
std::cout << "Combined Point Cloud size: " << combined->size() << std::endl;
// 设置点云可视化
pcl::visualization::PCLVisualizer viewer("Point Cloud Viewer");
viewer.setBackgroundColor(0,0,0);
viewer.addCoordinateSystem(1.0);
viewer.initCameraParameters();
/*设置点云大小*/
pcl::visualization::PointCloudColorHandlerCustom<pcl::PointXYZ> single_color(combined, 0, 255, 0); // 颜色
viewer.addPointCloud<pcl::PointXYZ>(combined, single_color,"combined_cloud");
viewer.setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 10, "combined_cloud"); // 大小
viewer.spin();
return 0;
}
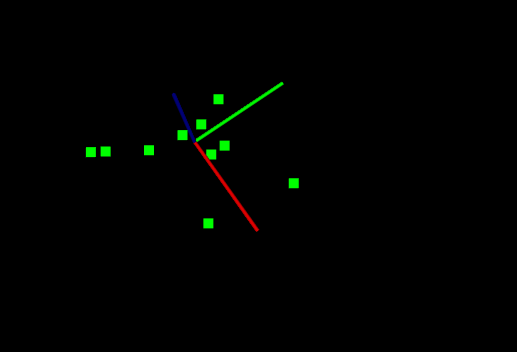
点云字段连接代码
#include <iostream>
#include <pcl/point_types.h>
#include <pcl/point_cloud.h>
#include <pcl/features/normal_3d.h>
#include <pcl/common/random.h>
#include <pcl/visualization/pcl_visualizer.h>
int main()
{
// ------------------------------
// 随机生成点云数据对象和法线数据对象
// -----------------------------
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>());//点云3D坐标
pcl::PointCloud<pcl::Normal>::Ptr normals(new pcl::PointCloud<pcl::Normal>);// 点云法线信息
pcl::PointCloud<pcl::PointNormal>::Ptr pointNormals(new pcl::PointCloud<pcl::PointNormal>);//点云3D坐标和法线信息
pcl::common::UniformGenerator<float> uniformGen(-1.0f, 1.0f); // 在范围(-1.0,1.0)内生成均匀分布的随机生成器
int numPoints = 5;
for (int i=0; i<numPoints; ++i){
pcl::PointXYZ point;
point.x = uniformGen.run();
point.y = uniformGen.run();
point.z = uniformGen.run();
cloud->push_back(point);
pcl::Normal normal;
normal._Normal::normal_x = uniformGen.run();
normal._Normal::normal_y = uniformGen.run();
normal._Normal::normal_z = uniformGen.run();
normals->push_back(normal);
}
// ------------------------------------
// 将XYZ 和 法线子段连接成一个新的点云数据对象
// -------------------------------------
pcl::concatenateFields(*cloud, *normals, *pointNormals);
// ------------------------------------
// 可视化点云和法线
// -------------------------------------
pcl::visualization::PCLVisualizer viewer("Point Cloud with Normals");
viewer.setBackgroundColor(0,0,0);
viewer.addCoordinateSystem(1.0);
viewer.cameraParamsSet();
// 添加点云和法向量到可视化对象
viewer.addPointCloud<pcl::PointNormal>(pointNormals, "cloud_normals");
viewer.setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 5, "cloud_normals");
viewer.setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_COLOR, 0.0, 1.0, 0.0, "cloud_normals");
// 显示法向量
viewer.addPointCloudNormals<pcl::PointNormal>(pointNormals,
1, // 每隔一个点进行法线可视化;为了方便可视化,避免过多的法线显示
0.1, // 表示法线的长度
"normals");
// 可视化循环
while(!viewer.wasStopped()){
viewer.spinOnce();
}
return 0;
}
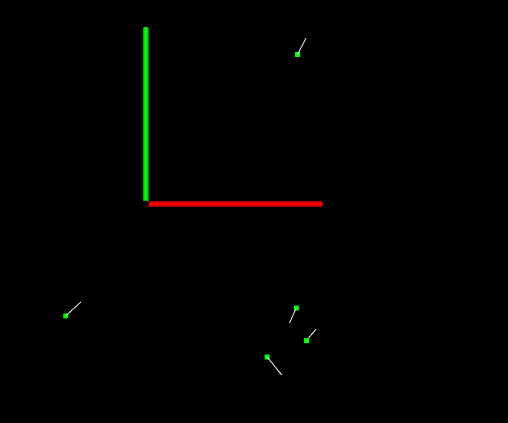