概述:在unity中利用顶点色简单实现面片从下到上的渐变
一、前期准备
绘制顶点色用来做什么?
通常用来分区域着色
1、模型绘制顶点色方法
- 在3D软件里面绘制,比如Maya、3Dmax、Blender等
- 在unity中使用插件
2、查看模型的顶点色
- 在3D软件中查看
- 使用shader中片元函数中输出
fixed4 frag (v2f i) : SV_Target{
return i.vertColor;
}
二、在shader中使用顶点色数据进行简单着色
1、新建shader和材质球,将创建的shader指定到材质球上,以及将材质球赋予到模型上。
2、shader属性
Properties
{
_MainColor("顶部颜色",Color) = (1.0,1.0,1.0,1.0)
_MainTex("顶部颜色贴图",2D) = "White"{
}
_SecondColor("底部颜色",Color) = (1.0,1.0,1.0,1.0)
_SecondTex("底部颜色贴图",2D) = "White"{
}
}
输入结构
struct appdata
{
float4 vertex : POSITION;
float2 uv : TEXCOORD0;
float4 vertexColor : COLOR; //输入顶点色数据
};
顶点函数
v2f vert (appdata v)
{
v2f o;
o.vertex = UnityObjectToClipPos(v.vertex);
o.uv = TRANSFORM_TEX(v.uv, _MainTex);
o.vertColor = v.vertexColor; //顶点色
return o;
}
输出结构
struct v2f
{
float2 uv : TEXCOORD0;
float4 vertColor : TEXCOORD1; //顶点色
float4 vertex : SV_POSITION;
};
声明定义
sampler2D _MainTex; float4 _MainTex_ST;
sampler2D _SecondTex; float4 _SecondTex_ST;
float4 _MainColor;
float4 _SecondColor;
片元函数
扫描二维码关注公众号,回复:
15072366 查看本文章
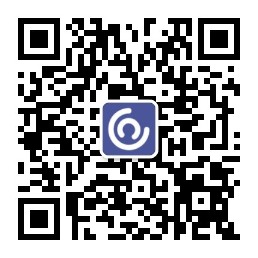
fixed4 frag (v2f i) : SV_Target
{
//用顶点色vertColor.r的r通道是因为刚才在查看模型顶点色的时候看到的渐变是用红色通道。所以就拿r通道来制作及渐变
fixed4 col = tex2D(_MainTex, i.uv) * _MainColor * i.vertColor.r + tex2D(_SecondTex, i.uv) * _SecondColor * (1.0 - i.vertColor.r );
return col;
}
完整代码
Shader "Unlit/vertexColor"
{
Properties
{
_MainColor("Main Color",Color) = (1.0,1.0,1.0,1.0)
_MainTex("MainTex",2D) = "White"{
}
_SecondColor("SecondColor",Color) = (1.0,1.0,1.0,1.0)
_SecondTex("SecondTex",2D) = "White"{
}
}
SubShader
{
Tags {
"RenderType"="Opaque" }
Pass
{
CGPROGRAM
#pragma vertex vert
#pragma fragment frag
// make fog work
#pragma multi_compile_fog
#include "UnityCG.cginc"
struct appdata
{
float4 vertex : POSITION;
float2 uv : TEXCOORD0;
float4 vertexColor : COLOR; //输入顶点色数据
};
struct v2f
{
float2 uv : TEXCOORD0;
float4 vertColor : TEXCOORD1; //顶点色
float4 vertex : SV_POSITION;
};
sampler2D _MainTex; float4 _MainTex_ST;
sampler2D _SecondTex; float4 _SecondTex_ST;
float4 _MainColor;
float4 _SecondColor;
v2f vert (appdata v)
{
v2f o;
o.vertex = UnityObjectToClipPos(v.vertex);
o.uv = TRANSFORM_TEX(v.uv, _MainTex);
o.vertColor = v.vertexColor; //顶点色
return o;
}
fixed4 frag (v2f i) : SV_Target
{
//着色
fixed4 col = tex2D(_MainTex, i.uv) * _MainColor * i.vertColor.r + tex2D(_SecondTex, i.uv) * _SecondColor * (1.0 - i.vertColor.r );
return col;
}
ENDCG
}
}
}