题目:在一个长度为n的数组里的所有数字都在0到n-1的范围内。 数组中某些数字是重复的,但不知道有几个数字是重复的。也不知道每个数字重复几次。请找出数组中任意一个重复的数字。例如,如果输入长度为7的数组{2,3,1,0,2,5,3},那么对应的输出是重复的数字2或者3。
解法1:排序
将输入的数组排序,然后扫描排序后的数组即可。
排序算法时间复杂度:O(nlogn),空间复杂度:O(1)。
这里偷个懒,使用stl库的sort()函数来对数组排序,sort()函数的具体实现结合了快速排序,插入排序和堆排序。
#include <iostream> #include "stdlib.h" #include<algorithm> #include <vector> using namespace std; int main() { int A[] = { 2,3,1,0,2,5,3 }; int len = sizeof(A) / sizeof(A[0]); std::vector<int> vector(A, A + 7); //将数组复制到容器内 std::sort(vector.begin(), vector.end());//排序 //输出排序后的结果 /*std::cout << "vector contains:" << endl; for (auto i : vector) std::cout << i << endl;*/ //遍历容易,找出重复的数字 for (std::vector<int>::iterator it = vector.begin(); it != vector.end(); ++it) { //迭代器相对容器起始位置的偏移即相当于数组的下标 if ((it - vector.begin()) != *it) { std::cout << "重复数字为:" << *it << endl; break; } } system("pause"); return 0; }
解法2:哈希表
从头到尾扫描数组,每扫描到一个数字,判断该数字是否在哈希表中,如果该哈希表还没有这个数字,那么加入哈希表,如果已经存在,则返回该重复的数字;
算法时间复杂度:O(n),空间复杂度:O(n)。
unordered_map的内部实现方式为哈希表。
具体代码如下:
#include <iostream> #include "stdlib.h" #include <unordered_map> using namespace std; int main() { int A[] = { 2,3,1,0,2,5,3 }; int len = sizeof(A) / sizeof(A[0]); int flag = 0;//是否找到重复数字的标志位 std::unordered_map<int, int> hashmap = { }; for (int i = 0; i < len; i++) { if ( hashmap.empty() )//判断哈希表是否为空 { hashmap.insert({ A[i], A[i] }); } else { //遍历哈希表 for ( auto num : hashmap ) { if (num.first == A[i]) { std::cout << "重复数字为:" << A[i] << endl; flag = 1; break; } } if( flag == 1) break; else hashmap.insert({ A[i], A[i] }); } } system("pause"); return 0; }
解法3:交换
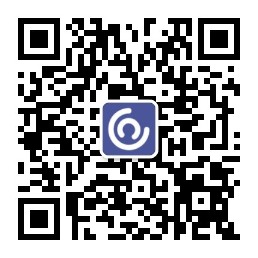
0~n-1正常的排序应该是A[i]=i;因此可以通过交换的方式,将它们都各自放回属于自己的位置;
从头到尾扫描数组A,当扫描到下标为i的数字m时,首先比较这个数字m是不是等于i,
如果是,则继续扫描下一个数字;
如果不是,则判断它和A[m]是否相等,如果是,则找到了第一个重复的数字(在下标为i和m的位置都出现了m);如果不是,则把A[i]和A[m]交换,即把m放回属于它的位置;
重复上述过程,直至找到一个重复的数字;
算法时间复杂度:O(n),空间复杂度:O(1)。
(将每个数字放到属于自己的位置最多交换两次)
具体代码如下:
#include <iostream> #include "stdlib.h" using namespace std; bool duplicate(int numbers[], int length, int& duplication) { //判断数组是否为空 if (numbers == nullptr || length <= 1) { return false; } //判断数组中的数字是否满足输入范围要求 for (auto i = 0; i < length; i++) { if (numbers[i] < 0 || numbers[i] > (length-1)) { return false; } } //遍历数组 for (int i = 0; i<length; i++) { while (numbers[i] != i) { if (numbers[i] == numbers[numbers[i]]) { duplication = numbers[i]; return true; } int tmp = numbers[i]; numbers[i] = numbers[tmp]; numbers[tmp] = tmp; } } return false; } int main() { int A[] = { 2,3,1,0,2,5,3 }; int len = sizeof(A) / sizeof(A[0]); int duplication; cout << duplicate(A, len, duplication) << endl; cout << duplication << endl; system("pause"); return 0; }
参考: