Threejs之MeshBasicMaterial(基本几何体)
import * as THREE from "three";
import {
OrbitControls } from "three/examples/jsm/controls/OrbitControls";
import gsap from "gsap";
import * as dat from "dat.gui";
import webglGlobalOptions from "./config/webgl";
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
camera.position.set(0, 0, 10);
scene.add(camera);
const geometry = new THREE.BoxGeometry(1, 1, 1);
const materialColor = {
color: 0x00ff00,
}
const material = new THREE.MeshBasicMaterial({
color: materialColor.color
});
const cube = new THREE.Mesh(geometry, material);
const gui = new dat.GUI();
gui
.add(cube.position, "x")
.min(0)
.max(5)
.step(0.01)
.name("移动物体的x轴")
.onChange((value) => {
console.log("x轴大小变化:", value);
}).onFinishChange(value => {
console.log("完成操作的x轴大小:", value);
});
gui.addColor(materialColor, "color").onChange(color=>{
cube.material.color.set(color)
}).name("物体填充色")
gui.add(cube, "visible").name("是否显示")
const cubeAnimateBtn = {
fn: () => {
gsap.to(cube.position, {
x: 5, duration: 5, yoyo: true});
}
}
gui.add(cubeAnimateBtn,"fn").name("点击物体运动")
const folder = gui.addFolder("物体配置项")
folder.add(cube.material, "wireframe").name("是否显示物体线框")
scene.add(cube);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
const controls = new OrbitControls(camera, renderer.domElement);
controls.enableDamping = true;
const axesHelper = new THREE.AxesHelper(
webglGlobalOptions.WEBGL_COORDINATE_AXIS_LENGTH
);
scene.add(axesHelper);
const clock = new THREE.Clock();
function render() {
controls.update();
renderer.render(scene, camera);
requestAnimationFrame(render);
}
render();
window.addEventListener("resize", () => {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
renderer.setPixelRatio(window.devicePixelRatio);
});
window.addEventListener("dblclick", () => {
const isExistFullScreenElement = document.fullscreenElement;
if (!isExistFullScreenElement) {
renderer.domElement.requestFullscreen();
}
document.exitFullscreen();
});
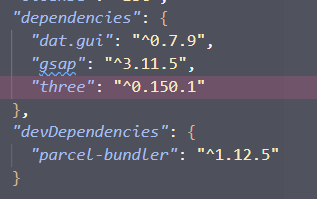