树存储方式能提高数据存储,读取的效率.
二叉树: 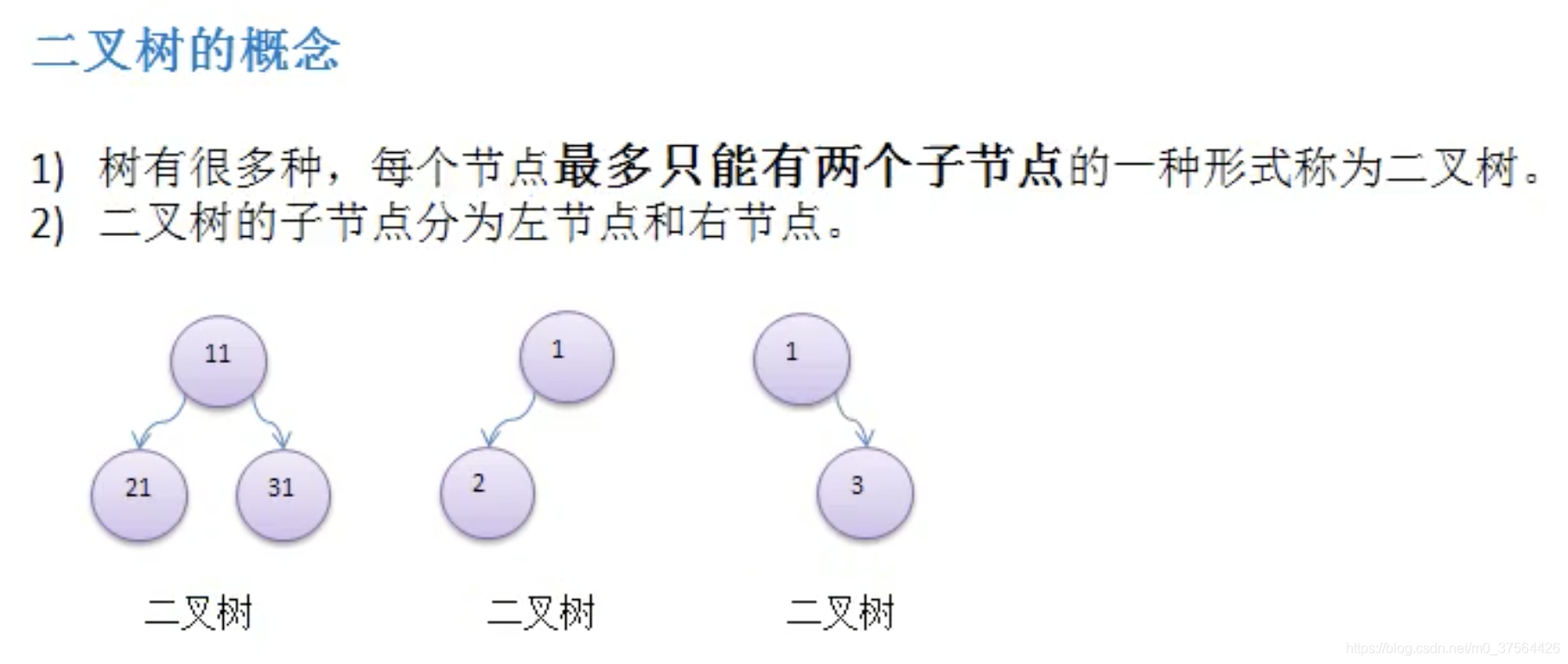
二叉树的遍历
前序遍历: 先输出父节点,再遍历左子树和右子树。
中序遍历: 先遍历左子树,再输出父节点, 再遍历右子树。
后序遍历: 先遍历左子树,再遍历右子树, 最后输出父节点。
public class BinaryTreeDemo {
public static void main(String[] args) {
// 先创建一棵二叉树
BinaryTree binaryTree = new BinaryTree();
// 创建需要的结点
HeroNode root = new HeroNode(1, "宋江");
HeroNode node2 = new HeroNode(2, "吴用");
HeroNode node3 = new HeroNode(3, "卢俊义");
HeroNode node4 = new HeroNode(4, "林冲");
HeroNode node5 = new HeroNode(5, "关胜");
binaryTree.setRoot(root);
root.setLeft(node2);
root.setRight(node3);
node3.setRight(node4);
node3.setLeft(node5);
// 前序遍历
System.out.println("前序遍历");
binaryTree.preOrder();
binaryTree.delNode(3);
System.out.println("删除后:");
binaryTree.preOrder();
//HeroNode nodeT = binaryTree.preOrderSearch(5);
//System.out.println(nodeT);
//BinaryTree.printCount();
HeroNode.count = 0;
// 中序遍历
//System.out.println("中序遍历");
//binaryTree.infixOrder();
//nodeT = binaryTree.infixOrderSearch(5);
//System.out.println(nodeT);
//BinaryTree.printCount();
HeroNode.count = 0;
// 后序遍历
//System.out.println("后序遍历");
//binaryTree.postOrder();
// nodeT = binaryTree.postOrderSearch(5);
// System.out.println(nodeT);
// BinaryTree.printCount();
}
}
class BinaryTree{
private HeroNode root;
public void setRoot(HeroNode root) {
this.root = root;
}
public void delNode(int no){
if(this.root == null){
System.out.println("二叉树为空");
}else if(this.root != null && this.root.getNo() == no){
this.root = null;
}else{
this.root.delNode(no);
}
}
// 前序遍历
public void preOrder(){
if(this.root != null){
this.root.preOrder();
}else{
System.out.println("二叉树为空,无法遍历");
}
}
// 中序遍历
public void infixOrder(){
if(this.root != null){
this.root.infixOrder();
}else{
System.out.println("二叉树为空,无法遍历");
}
}
// 后序遍历
public void postOrder(){
if(this.root != null){
this.root.postOrder();
}else{
System.out.println("二叉树为空,无法遍历");
}
}
// 前序遍历查找
public HeroNode preOrderSearch(int no){
if(this.root != null){
return this.root.preOrderSearch(no);
}else{
System.out.println("二叉树为空,无法遍历");
return null;
}
}
// 中序遍历查找
public HeroNode infixOrderSearch(int no){
if(this.root != null){
return this.root.infixOrderSearch(no);
}else{
System.out.println("二叉树为空,无法遍历");
return null;
}
}
// 后序遍历查找
public HeroNode postOrderSearch(int no){
if(this.root != null){
return this.root.postOrderSearch(no);
}else{
System.out.println("二叉树为空,无法遍历");
return null;
}
}
public static void printCount(){
HeroNode.printCount();
}
}
class HeroNode{
public static int count = 0;
private int no;
private String name;
private HeroNode left; // 默认为null
private HeroNode right; // 默认为null
public HeroNode(int no, String name) {
this.no = no;
this.name = name;
}
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public HeroNode getLeft() {
return left;
}
public void setLeft(HeroNode left) {
this.left = left;
}
public HeroNode getRight() {
return right;
}
public void setRight(HeroNode right) {
this.right = right;
}
@Override
public String toString() {
return "HeroNode{" +
"no=" + no +
", name='" + name + '\'' +
'}';
}
// 递归删除结点
public void delNode(int no){
if(this.left != null){
if(this.left.no == no){
this.left = null;
return;
}else{
this.left.delNode(no);
}
}
if(this.right != null){
if(this.right.no == no){
this.right = null;
return;
}else{
this.right.delNode(no);
}
}
}
// 前序遍历
public void preOrder(){
System.out.println(this); // 先输出父结点
// 递归左子树
if(null != this.left){
this.left.preOrder();
}
// 递归右子树
if(null != this.right){
this.right.preOrder();
}
}
// 中序遍历
public void infixOrder(){
// 先递归左子树
if(null != this.left){
this.left.infixOrder();
}
System.out.println(this); // 输出父结点
// 最后递归右子树
if(null != this.right){
this.right.infixOrder();
}
}
// 后序遍历
public void postOrder(){
// 先递归左子树
if(null != this.left){
this.left.postOrder();
}
// 再递归右子树
if(null != this.right){
this.right.postOrder();
}
System.out.println(this); // 最后输出父结点
}
// 前序遍历查找
public HeroNode preOrderSearch(int no){
count++;
HeroNode node = null;
if(this.no == no){
return this;
}
if(this.left != null){
node = this.left.preOrderSearch(no);
}
if(null != node){
return node;
}
if(this.right != null){
node = this.right.preOrderSearch(no);
}
return node;
}
// 中序遍历查找
public HeroNode infixOrderSearch(int no){
HeroNode node = null;
if(this.left != null){
node = this.left.infixOrderSearch(no);
}
if(null != node){
return node;
}
count++;
if(this.no == no){
return this;
}
if(this.right != null){
node = this.right.infixOrderSearch(no);
}
return node;
}
// 后序遍历查找
public HeroNode postOrderSearch(int no){
HeroNode node = null;
if(this.left != null){
node = this.left.postOrderSearch(no);
}
if(null != node){
return node;
}
if(this.right != null){
node = this.right.postOrderSearch(no);
}
if(null != node){
return node;
}
count++;
if(this.no == no){
return this;
}
return node;
}
public static void printCount(){
System.out.printf("遍历了%d次\n",count);
}
}