原文详解:spring boot项目集成swagger2管理
1.使用原因与好处
在开发中,避免不了的就是为接口编写文档,占用相当大的一部分时间,
同时也需要版本更新以及维护。
在数据对象中当接口字段产生变化时,我们需要更新字段并立即告知前端,来进行修改。
现在我们用swagger2来完成这样的在线生成接口文档。
2.使用方法
编写测试代码用以举例说明如下:
2.1继承swagger2,在pom文件中添加依赖
<!--swagger接口组件-->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.6.1</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.6.1</version>
</dependency>
2.2添加swagger2的配置文件
package com.wahct.amll.config.swagger;
import io.swagger.annotations.ApiOperation;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.ApiInfoBuilder;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
/**
* 类描述:swagger配置文件
* Created by 李泽阳 on 2019/3/12
*/
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
//.pathMapping("/develop/")//对请求的路径增加develop前缀
//.globalOperationParameters(setHeaderToken())//获取token方法
.select()
.apis(RequestHandlerSelectors.withMethodAnnotation(ApiOperation.class)) //只过滤包含有ApiOperation注解的方法
.paths(PathSelectors.any()) //对所有的路径进行监控
.build();
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("手表商城系统API接口")
//.description("项目工程描述")
.termsOfServiceUrl("http://licocom.com/")
.version("1.0.0")
.build();
}
}
2.3在启动项上开启扫描类
在启动项上添加扫描
package com.wahct.amll;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
import java.util.logging.Logger;
@SpringBootApplication
@EnableSwagger2//开启Swagger
public class AmllApplication {
private static Logger logger = Logger.getLogger(String.valueOf(AmllApplication.class));
public static void main(String[] args) {
SpringApplication.run(AmllApplication.class, args);
//logger.info("================开始启动===============");
//logger.info("================启动成功===============");
}
}
3.为接口编写说明
3.1编写controller文档说明
package com.wahct.amll.controller;
import com.wahct.amll.common.Response.ResponseMessage;
import com.wahct.amll.common.Response.Result;
import com.wahct.amll.service.ProductServiceI;
import com.wahct.amll.vo.ProductUpdateVo;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
/**
* 类描述:产品对外接口
* Created by 李泽阳 on 2019/3/12
*/
@RestController
@Api(tags = "ProductInterfaces", description = "产品接口")
@RequestMapping("/product")
public class ProductController {
@Autowired
private ProductServiceI productServiceI;
//通过Id查询产品详情
@RequestMapping(value = "getById/{Id}", method = RequestMethod.POST)
@ResponseBody
@ApiOperation(value = "产品查询ById", notes = "根据Id查询对应产品详情", httpMethod = "POST", produces = "application/json")
public ResponseMessage<?> getById(@ApiParam(required = true, name = "id", value = "40283a0e696fe38e01696fe413d20005") @PathVariable("Id") String Id) {
try {
List list = productServiceI.queryByIdPro(Id);
return Result.success(list.get(0));
} catch (Exception e) {
e.printStackTrace();
return Result.error("数据查询失败!");
}
}
//通过Id更新数据
@RequestMapping(value = "updateById", method = RequestMethod.POST)
@ResponseBody
@ApiOperation(value = "产品更新ById", notes = "根据Id更新产品详细信息", httpMethod = "POST", produces = "application/json")
public ResponseMessage<?> updateById(@RequestBody ProductUpdateVo productUpdateVo) {
try {
productServiceI.updateProduct(productUpdateVo);
return Result.success("数据更新成功!");
} catch (Exception e) {
e.printStackTrace();
return Result.error("数据更新失败!");
}
}
}
在方法上编写说明,如果采用键值对采用@ApiParam,类方法采用@Api注解
3.2为vo模型注解文档
package com.wahct.amll.vo;
import io.swagger.annotations.ApiModelProperty;
import lombok.Getter;
import lombok.Setter;
/**
* 类描述:产品新增接口数据对象
* Created by 李泽阳 on 2019/3/12
*/
@Getter
@Setter
public class ProductVo {
/*产品名称*/
@ApiModelProperty(value = "产品名称", required = true, example = "Armani阿玛尼")
private String productName;
/*产品数量*/
@ApiModelProperty(value = "产品数量", required = true, example = "100")
private Integer productNumber;
/*备注说明*/
@ApiModelProperty(value = "产品备注说明", required = true, example = "阿玛尼( Armani) 乔治·阿玛尼(Giorgio Armani)创立于意大利米兰。")
private String notepad;
}
4.生成在线接口文档
http://localhost:8080/swagger-ui.html 注意:添加对应访问后缀和修改项目端口。
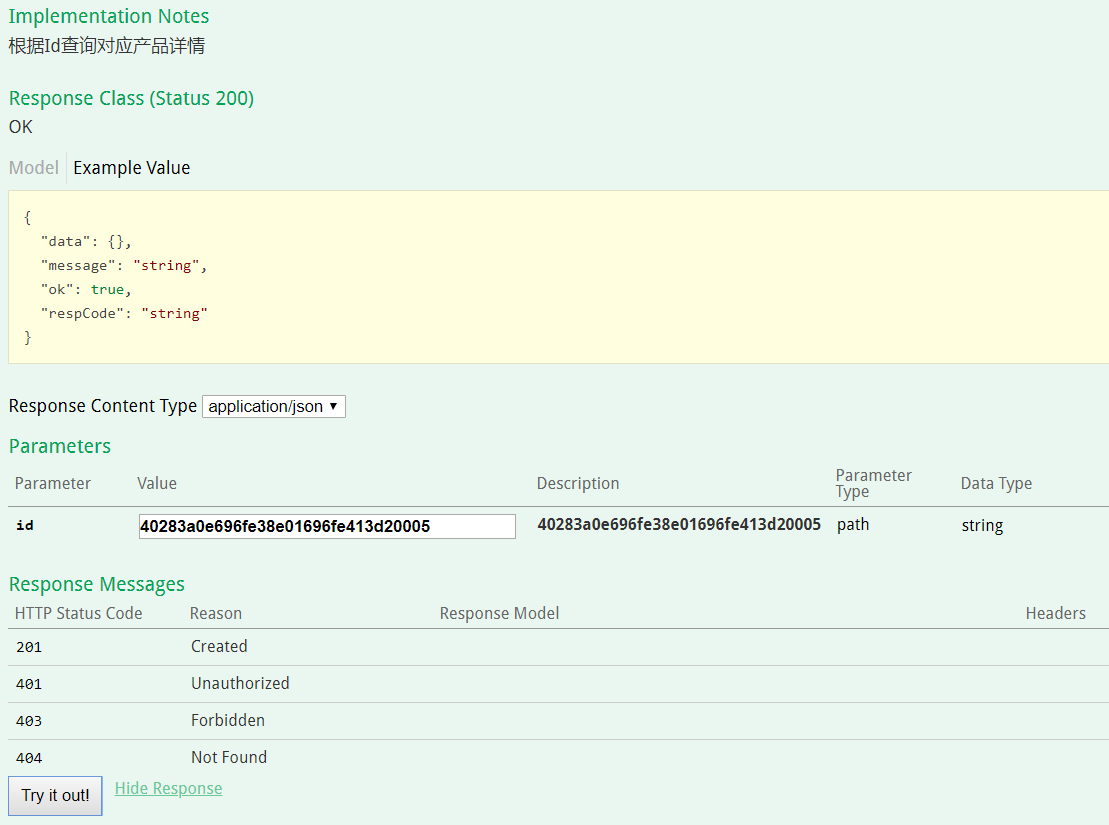
响应体
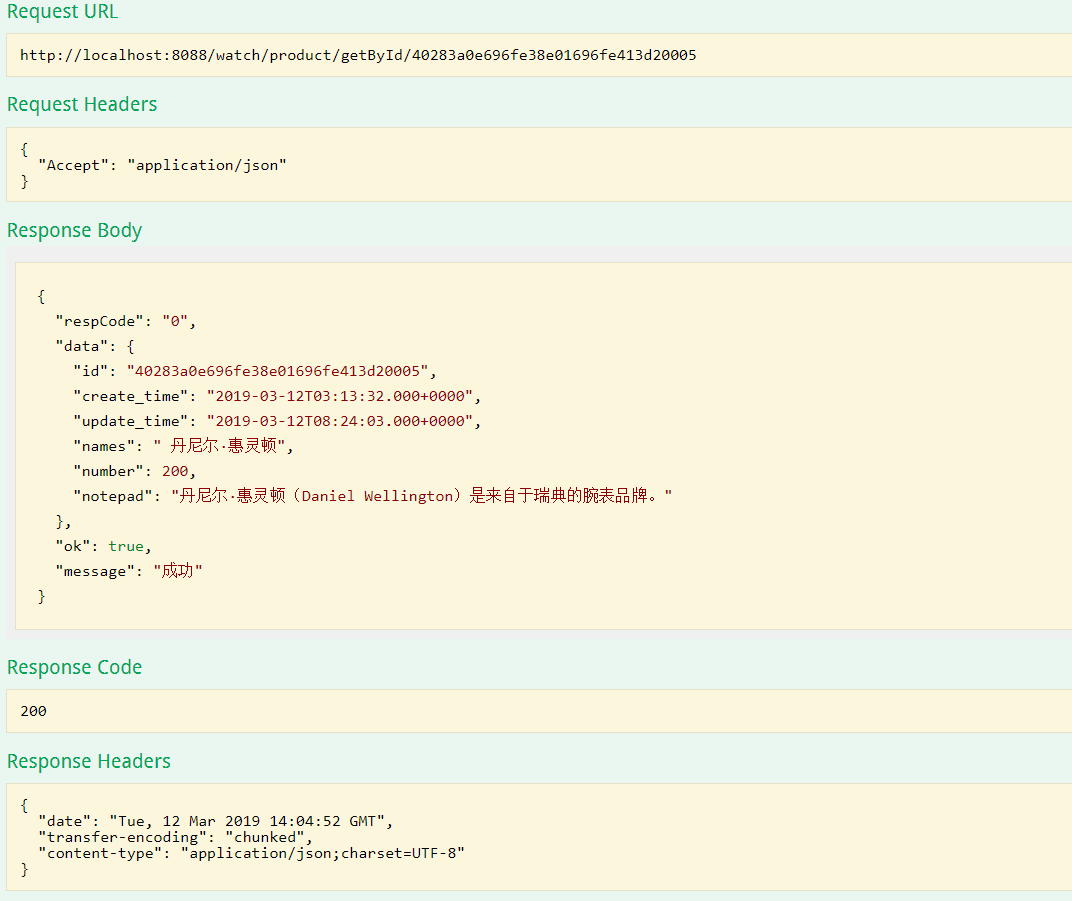
5.各注解用法说明
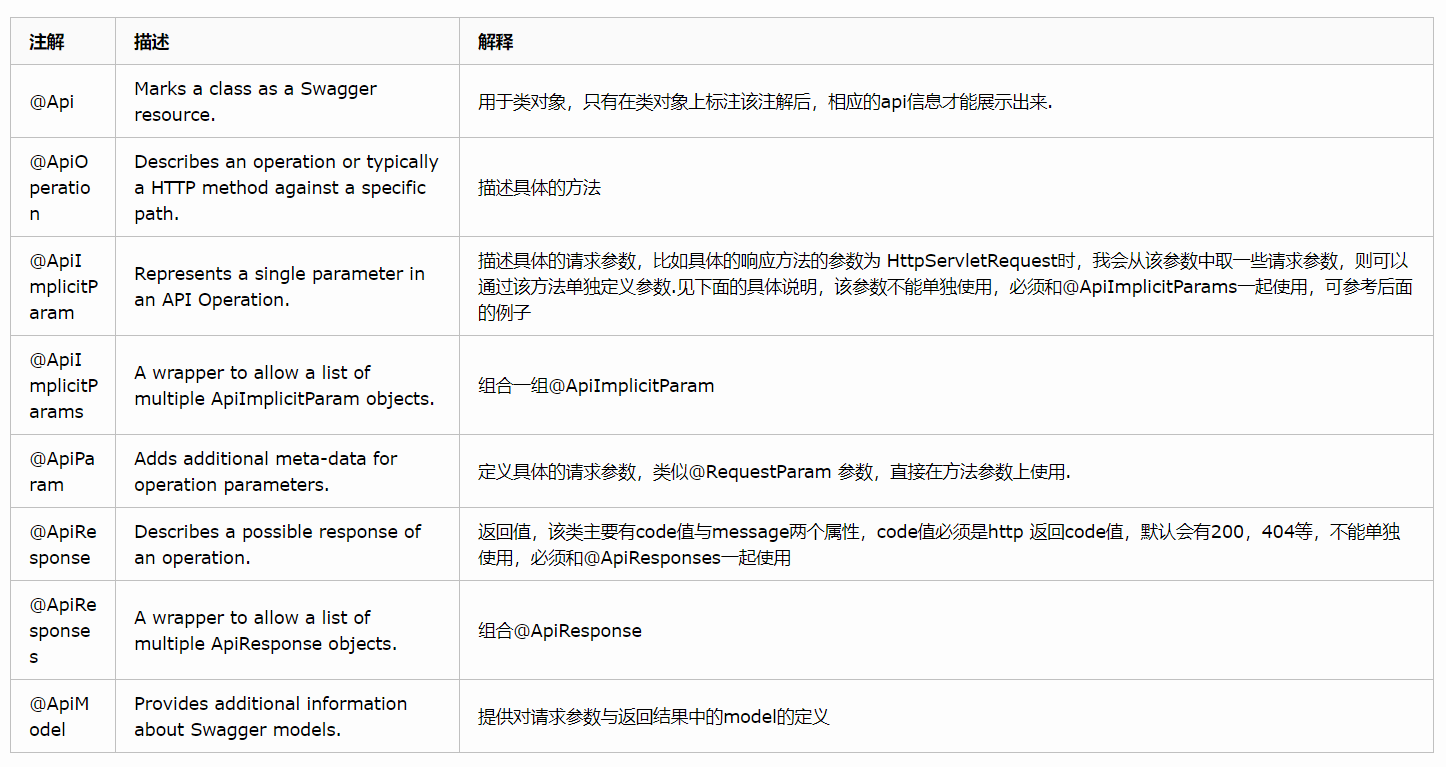
6.注意事点/以及不足
1.线上环境建议关闭接口文档。
2. tags标题有中文bug,中文时不可展开接口列表,需要英文。
3.使用springboot1.5.5 对应swagger2.6.1。
面向开发过程,记录学习之路