import numpy as np
File Input and Output
NumPy is able to save and load data to and from disk either in text or binary format. In this section I only discuss NumPy's built-in binary format, since most users wil prefer pandas and other tools for loading text or tabular data.
np.save and np.load are the two workhorse functions(主要的函数) for efficiently saving and loading array data on disk. Arrays are saved by default in an uncompressed(未压缩的) raw binary format with file extension .npy:
arr = np.arange(10)
"保存数组"
np.save('../examples/some_arry', arr)
'保存数组'
If the file path does not already end in .npy, the extension will be appended. The array o disk can then be oaded with np.load.
np.load('../examples/some_array.npy')
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9], dtype=int64)
You save multiple arrays in an uncompressed(未解压的) archive using np.savez and passing the arrays as keyword arguments:
When loading an .npz file, you get back a dict-like object that loads the individual(个别的) arrays lazily.
np.savez("../examples/array_archive.npz", a=arr, b=arr)
arch = np.load('../examples/array_archive.npz')
'多个数组取出时, 是惰性加载的, 类似生成器'
arch['b']
'多个数组取出时, 是惰性加载的, 类似生成器'
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
if your data compresses well, you may wish to use numpy.savez_compressed instead:
np.savez_compressed('../examples/arrays_compressed.npz', a=arr, b=arr)
Linear Algebra
Linear algera, like matrix multiplication(乘法), decompositions(分解), determinats(秩), and other square matrix math, is an important part of any array library. Unlike some languages lile MATLIB, multiplying two, two-dimensional array with * is an element-wise product instead of a matrix dot product.(python 中的星号, 表示两个数组的对应元素乘, 而非矩阵乘哦) Thus, there is a function dot, both an array method and a function in the numpy namespace, for matrix multiplication.
"2x3"
x = np.array([[1,2,3], [4,5,6]])
"3x2"
y = np.array([[6,23], [-1,7], [8,9]])
x
y
'2x3'
'3x2'
array([[1, 2, 3],
[4, 5, 6]])
array([[ 6, 23],
[-1, 7],
[ 8, 9]])
"2x3 * 3x2 = 2x2, 对左边列向量的线性组合嘛"
x.dot(y)
"x.dot(y) is equivalent to np.dot(x,y)"
np.dot(x,y)
'2x3 * 3x2 = 2x2, 对左边列向量的线性组合嘛'
array([[ 28, 64],
[ 67, 181]])
'x.dot(y) is equivalent to np.dot(x,y)'
array([[ 28, 64],
[ 67, 181]])
A matrix product between a two-dimensional array and a suitably sized one-dimensional array results in a one-dimensional array:
np.dot(x, np.ones(3))
array([ 6., 15.])
The @ symbol also works as an infix operator(强制插入) that performs matrix multiplication:
x @ np.ones(3)
array([ 6., 15.])
numpy.linalg has a stardard set of matrix decompositions and things like inverse and determinant. These are implemented(被运行) under the hood via(通过) the same industry-standard linear algebra libraies used in other languages like MATLAB and R.. -> Python的这些矩阵的函数都是和像这样的语言用的同一个标准.
from numpy.linalg import inv, qr
x = np.random.randn(5,5)
"计算内积 $A^TA$"
mat = x.T.dot(x)
'矩阵求逆 inv'
inv(mat)
'计算内积 $A^TA$'
'矩阵求逆 inv'
array([[ 2.85462863, -0.08842397, -0.01719878, 0.28840731, 1.33531619],
[-0.08842397, 0.36385157, 0.05790547, 0.21733807, -0.04179607],
[-0.01719878, 0.05790547, 0.16845103, 0.03607368, -0.07364311],
[ 0.28840731, 0.21733807, 0.03607368, 0.33697629, 0.22115989],
[ 1.33531619, -0.04179607, -0.07364311, 0.22115989, 0.89288396]])
"矩阵与其逆的积-> 单位阵"
mat.dot(inv(mat))
'矩阵的QR分解, Q是正交矩阵, R是上三角矩阵'
q, r = qr(mat)
q
r
'矩阵与其逆的积-> 单位阵'
array([[ 1.00000000e+00, -1.34588695e-17, 1.20337000e-17,
1.43461087e-16, 1.19991758e-16],
[-1.44647137e-16, 1.00000000e+00, -2.44723192e-19,
-3.05504861e-16, 1.85480504e-17],
[-5.64972079e-16, 1.06290868e-17, 1.00000000e+00,
-1.24805205e-17, -1.52382375e-17],
[ 2.64575648e-17, -3.37897541e-18, -3.46206228e-17,
1.00000000e+00, -2.61606309e-16],
[-4.05280510e-16, -4.83836278e-17, 2.70272255e-17,
-3.85392887e-17, 1.00000000e+00]])
'矩阵的QR分解, Q是正交矩阵, R是上三角矩阵'
array([[-0.49090965, -0.10814586, -0.24518935, 0.10423654, 0.82239231],
[ 0.00323168, -0.7867822 , -0.00793056, -0.61663426, -0.02574129],
[ 0.32640742, 0.12703087, -0.92399902, -0.14659492, -0.04535519],
[-0.1390847 , 0.59414019, 0.14745407, -0.76639263, 0.13620759],
[ 0.79568267, -0.01178262, 0.25357916, -0.00701326, 0.54990788]])
array([[-2.59814895, 1.59367406, 4.62351953, -3.75681459, 6.16316304],
[ 0. , -7.15657133, 0.57430456, 7.77949798, -2.22774585],
[ 0. , 0. , -6.05158388, 1.46469814, -0.57791469],
[ 0. , 0. , 0. , -2.70965304, 0.66330379],
[ 0. , 0. , 0. , 0. , 0.61587833]])
The express x.T.dot(x) computes the dot product of x with its transpose x.T
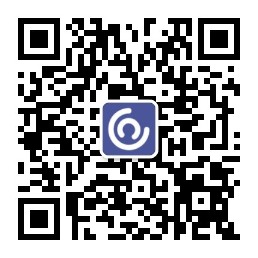
See Table 4-7 for a list of some of the most commonly used linear algbrea functions.
- diag 对角化
- dot 矩阵乘法
- trace 迹: Compute the sum of the diagonal elements
- det 行列式 Compute the matrix determinant
- inv 逆 Compute the inverse of a square matrix
- eig, qr, svd 矩阵的谱分解, QR分解, SVD 分解
- solve 线性方程的解 Solve the linear system Ax=b for x where A is a square matrix
- lstsq 最小二乘近似解 Compute the least-squares solution to Ax=b