springboot官方web开发文档:https://docs.spring.io/spring-boot/docs/2.0.6.RELEASE/reference/htmlsingle/#boot-features-spring-mvc
Web开发支持
Spring Boot 为 Web 开发提供了 spring-boot-starter-web 启动器作为基本支持,为我们提供了嵌入的Tomcat 以及 Spring MVC 的依赖支持。(参考:pom.xml)
也提供了很多不同场景的自动配置类,让我们只需要在配置文件中指定少量的配置即可启动项目。自动配置类存储在 spring-boot-autoconfigure.jar 的 org.springframework.boot.autoconfigure 包下。
自动配置场景 SpringBoot 帮我们配置了什么?是否修改?能修改哪些配置?是否可以扩展?……
从文件名可以看出:
xxxxAutoConfiguration :向容器中添加自动配置组件
xxxxProperties :使用自动配置类 来封装 配置文件的内容
SpringMVC配置 : WebMvcAutoConfiguration 和 WebMvcProperties
内嵌 Servlet 容器 : ServletWebServerFactoryAutoConfiguration 和 ServerProperties
上传文件的属性 :MultipartAutoConfiguration 和 MultipartProperties
JDBC : DataSourceAutoConfiguration 和 DataSourceProperties
。。。。。。
也提供了很多不同场景的自动配置类,让我们只需要在配置文件中指定少量的配置即可启动项目。自动配置类存储在 spring-boot-autoconfigure.jar 的 org.springframework.boot.autoconfigure 包下。
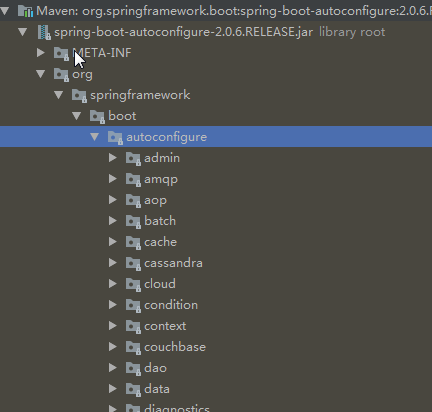
自动配置场景 SpringBoot 帮我们配置了什么?是否修改?能修改哪些配置?是否可以扩展?……
从文件名可以看出:
xxxxAutoConfiguration :向容器中添加自动配置组件
xxxxProperties :使用自动配置类 来封装 配置文件的内容
SpringMVC配置 : WebMvcAutoConfiguration 和 WebMvcProperties
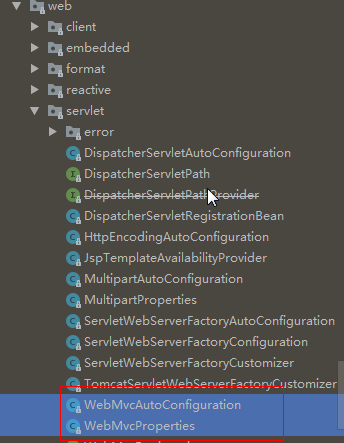
内嵌 Servlet 容器 : ServletWebServerFactoryAutoConfiguration 和 ServerProperties
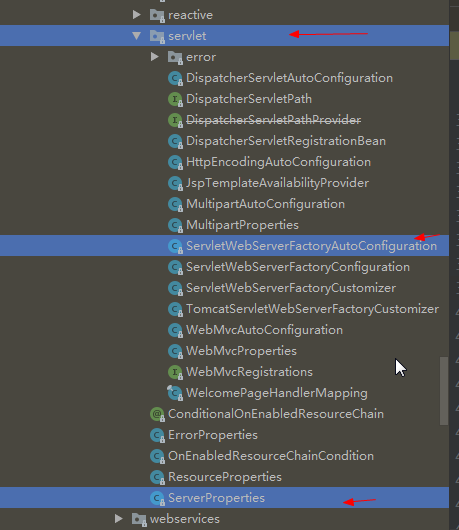
上传文件的属性 :MultipartAutoConfiguration 和 MultipartProperties
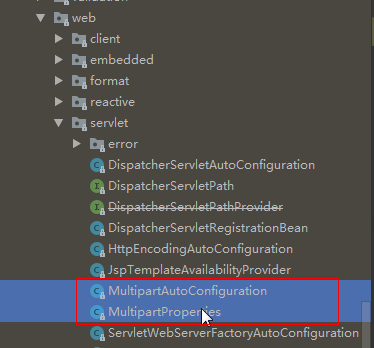
JDBC : DataSourceAutoConfiguration 和 DataSourceProperties
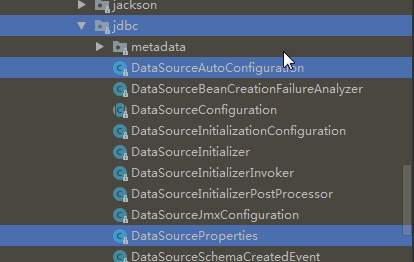
。。。。。。
静态资源的映射规则
对静态资源的映射规则, 可通过分析 WebMvcAutoConfiguration 自动配置类得到
webjars资源映射
在 WebMvcAuotConfiguration.addResourceHandlers() 分析webjars 资源映射
1. 所有 /webjars/** 请求,都去 classpath:/META-INF/resources/webjars/ 目录找对应资源文件
2. webjars:以jar包的方式引入静态资源
webjars官网: https://www.webjars.org/
3. 在官网打开资源文件的依赖配置信息,然后粘贴到 pom.xml 中
4. 访问 localhost:8080/webjars/jquery/3.3.1/jquery.js 会在下面路径 中查找
1. 所有 /webjars/** 请求,都去 classpath:/META-INF/resources/webjars/ 目录找对应资源文件
2. webjars:以jar包的方式引入静态资源
webjars官网: https://www.webjars.org/
3. 在官网打开资源文件的依赖配置信息,然后粘贴到 pom.xml 中
4. 访问 localhost:8080/webjars/jquery/3.3.1/jquery.js 会在下面路径 中查找
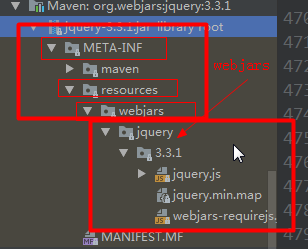
其他静态资源映射
在 WebMvcAuotConfiguration.addResourceHandlers() 分析 访问其他资源映射
staticPathPattern 处理其他访问的静态路径, 从 WebMVCProperties 构造器中获取到 /**
ResourceProperties 根据请求查找资源文件, 从以下 四个路径 中 查找( 静态资源目录 )
当接受到 /** 请求访问资源时, 会被映射到下面4个 类路径下的静态资源目录中 查找
访问 localhost:8080/style.css 会在上面四个静态资源路径 中查找文件
staticPathPattern 处理其他访问的静态路径, 从 WebMVCProperties 构造器中获取到 /**
ResourceProperties 根据请求查找资源文件, 从以下 四个路径 中 查找( 静态资源目录 )
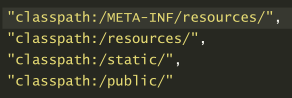
当接受到 /** 请求访问资源时, 会被映射到下面4个 类路径下的静态资源目录中 查找
访问 localhost:8080/style.css 会在上面四个静态资源路径 中查找文件
欢迎页映射
在 WebMvcAuotConfiguration.welcomePageHandlerMapping() 分析 欢迎页映射
getWelcomePage() 方法获取 欢迎页面 可存储路径
分析后, 会从 4个静态资源目录 + 根路径 / 中 查找 index.html 页面
会在 静态资源目录下 与 根路径查找 (按该顺序) index.html页面; 收到 "/**" 请求映射
访问 localhost:8080/ 会在上面5个目录中查找 index.html 页面(因为/也属于 /** )
getWelcomePage() 方法获取 欢迎页面 可存储路径
分析后, 会从 4个静态资源目录 + 根路径 / 中 查找 index.html 页面
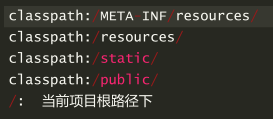
会在 静态资源目录下 与 根路径查找 (按该顺序) index.html页面; 收到 "/**" 请求映射
访问 localhost:8080/ 会在上面5个目录中查找 index.html 页面(因为/也属于 /** )
图标映射
Spring Boot 会在静态资源目录下 与 根路径(按该顺序) 查找 faicon.ico 页面;
如果存在这样的文件,Spring Boot 会自动将其设置为应用图标。
如果存在这样的文件,Spring Boot 会自动将其设置为应用图标。
classpath:/META-INF/resources/
classpath:/resources/
classpath:/static/
classpath:/public/
/: 当前项目根路径下
Thymeleaf模板引擎
Spring Boot 官方不推荐使用JSP,因为内嵌的 Tomcat 、Jetty 容器不支持以 jar 形式运行 JSP。Spring Boot
中提供了大量模板引擎,包含 Freemarker、Mastache、Thymeleaf 等。 而 Spring Boot 官方推荐使用
Thymeleaf 作为模板引擎, 因为 Thymeleaf 提供了完美的 SpringMVC 的支持。
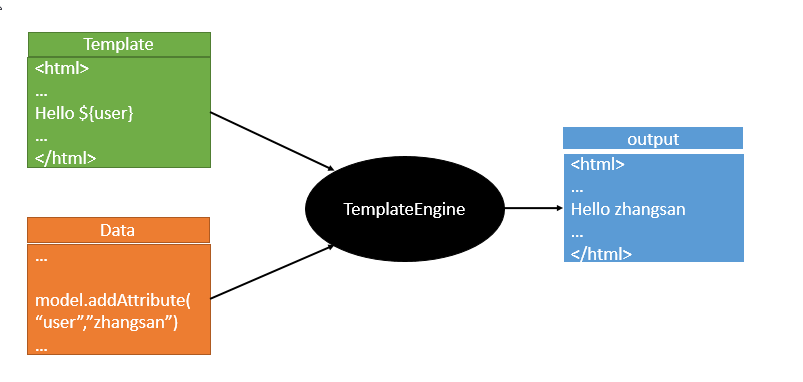
引入 Thymeleaf
pom.xml 加入 Thymeleaf 启动器
<!-- thymeleaf 模板启动器 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
使用Thymeleaf

通过分析发现, 将 HTML 页面放到 classpath:/templates/ 目录下, Thymeleaf 就能自动渲染
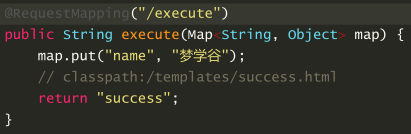
发送 http://localhost:8080/execute 后, 通过上面代码转到 classpath:/templates/success.html
导入 Thymeleaf 的名称空间:在 html 页面加上以下名称空间, 使用 Thymeleaf 时就有语法提示。
<html xmlns:th="http://www.thymeleaf.org">
Thymeleaf简单语法
常用属性:
表达式语法:
优先级 | 属性名 | 作用 |
1 | th:insert th:replace |
引入片段,与th:fragment声明组合使用; 类似于 jsp:include |
2 | th:each | 遍历 , 类似于 c:forEach |
3 | th:if th:unless th:switch th:case |
条件判断, 类似于 c:if |
4 | th:object th:with |
声明变量, 类似于 c:set |
5 | th:attr th:attrprepend th:attrappend |
修改任意属性, prepend前面追加, append后面追加 |
6 | th:value th:href th:src ... |
修改任意html原生属性值 |
7 | th:text th:utext |
修改标签体中的内容, th:text 转义特殊字符, 即 h1标签以文本显示出来 th:utext 是不转义特殊字符, 即 h1 标签展现出本来效果 |
8 | th:fragment | 声明片段 |
9 | th:remove | 移除片段 |
表达式语法:
一、Simple expressions(表达式语法)
1. Variable Expressions(变量表达式): ${...} (参考: 4.2 Variables)
1)、获取变量值;使用OGNL表达式;
2)、获取对象的属性, 调用方法
3)、使用内置的基本对象:
#ctx : the context object.(当前上下文对象)
#vars: the context variables.(当前上下文里的变量)
#locale : the context locale. (当前上下文里的区域信息)
下面是Web环境下的隐式对象
#request : (only in Web Contexts) the HttpServletRequest object.
#response : (only in Web Contexts) the HttpServletResponse object.
#session : (only in Web Contexts) the HttpSession object.
#servletContext : (only in Web Contexts) the ServletContext object.
示例: ${session.foo} (用法参考: 18 Appendix A: Expression Basic Objects)
4)、使用内置的工具对象:(用法参考: 19 Appendix B: Expression Utility Objects)
#execInfo : information about the template being processed.
#messages : methods for obtaining externalized messages inside variables
expressions, in the same way as they would be obtained using #{…} syntax.
#uris : methods for escaping parts of URLs/URIs
#conversions : methods for executing the configured conversion service
(if any).
#dates : methods for java.util.Date objects: formatting, component
extraction, etc.
#calendars : analogous to #dates , but for java.util.Calendar
objects.
#numbers : methods for formatting numeric objects.
#strings : methods for String objects: contains, startsWith,
prepending/appending, etc.
#objects : methods for objects in general.
#bools : methods for boolean evaluation.
#arrays : methods for arrays.
#lists : methods for lists.
#sets : methods for sets.
#maps : methods for maps.
#aggregates : methods for creating aggregates on arrays or collections.
#ids : methods for dealing with id attributes that might be repeated
(for example, as a result of an iteration).
2. Selection Variable Expressions(选择表达式): *{...}
(参考:4.3 Expressions on selections)
1)、和${}在功能上是一样, 额外新增:配合 th:object 使用
<div th:object="${session.user}">
省得每次写${session.user.firstName}, 直接取出对象,然后写对象名即可
<p>Name: <span th:text="*{firstName}">Sebastian</span> </p>
<p>Email: <span th:text="*{email}">Saturn</span> </p>
</div>
3. Message Expressions(获取国际化内容): #{...} (参考:4.1 Messages)
4. Link URL Expressions(定义URL): @{...} (参考:4.4 Link URLs)
5. Fragment Expressions(片段引用表达式): ~{...} (参考:4.5 Fragments)
<div th:insert="~{commons :: main}">...</div>
二、Literals(字面量) (参考: 4.6 Literals)
1. Text literals: 'one text' , 'Another one!' ,…
2. Number literals: 0 , 34 , 3.0 , 12.3 ,…
3. Boolean literals: true , false
4. Null literal: null
5. Literal tokens: one , sometext , main ,…
三、Text operations(文本操作) (参考: 4.7 Appending texts)
1. String concatenation: +
2. Literal substitutions: |The name is ${name}|
四、Arithmetic operations(数学运算) (参考: 4.9 Arithmetic operations)
1. Binary operators: + , - , * , / , %
2. Minus sign (unary operator): -
五、Boolean operations(布尔运算)
1. Binary operators: and , or
2. Boolean negation (unary operator): ! , not
五、Comparisons and equality(比较运算) (参考: 4.10 Comparators and Equality)
1. Comparators: > , < , >= , <= ( gt , lt , ge , le )
2. Equality operators: == , != ( eq , ne )
六、Conditional operators(条件表达式;三元运算符) (参考: 4.11 Conditional expressions)
1. If-then: (if) ? (then)
2. If-then-else: (if) ? (then) : (else)
3. Default: (value) ?: (defaultvalue)
七、Special tokens(特殊操作) (参考: 4.13 The No-Operation token)
1. No-Operation: _
SpringBoot热部署
默认情况下, 在开发中我们修改一个项目文件后,想看到效果不得不重启应用,这会导致浪费大量时间 ,我们希望不重启应用的情况下,程序可以自动部署(热部署)。
在 Spring Boot 开发环境下禁用模板缓存:
在 Spring Boot 开发环境下禁用模板缓存:
#开发环境下关闭thymeleaf模板缓存,thymeleaf默认是开启状态
spring.thymeleaf.cache=false
添加 Spring Boot Devtools 热部署依赖:
<!--热部署-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
</dependency>
Intellij IEDA和Eclipse不同,Intellij IDEA必须做一些小调整:
- 在 Eclipse 中,修改文件后要手动进行保存,它就会自动编译,就触发热部署现象。
- 在Intellij IEDA 中,修改文件后都是自动保存,默认不会自动编译文件,需要手动编译按 Ctrl + F9 (推荐使用)或 Build -> Build Project ;或者进行以下设置才会自动编译(效果不明显)
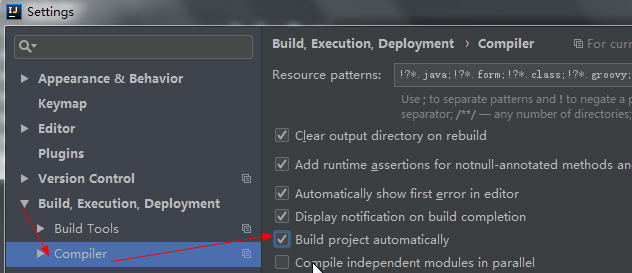