Vue-CLI
一个官方出品的快速搭建Vue项目的脚手架工具!
安装
npm install @vue/cli -g
使用
vue create 项目名
然后一通选择...就OK了
可视化创建项目
vue ui
VueCLI3创建的Vue项目的目录
我们写的代码主要在src目录下
一个.vue
文件就是一个组件对象
main.js
是入口文件,项目运行从main.js开始的
router.js
是项目的路由配置,旧版本路由会存在router/idnex.js
里面
App.vue
是项目的根组件,渲染从这里开始。
扫描二维码关注公众号,回复:
6088616 查看本文章
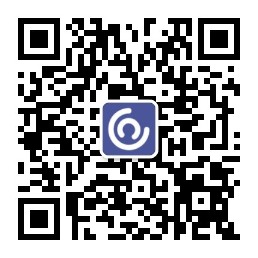
补充
拿到一个Vue项目,怎么运行起来?
先切换到项目目录下
安装项目依赖包
npm install
启动项目
npm run serve
项目开发完,要打包上线
npm run build
注意事项
scoped 参数 声明此 样式 只在 当前 组件中生效
<style scoped>
</style>
element-ui
element-ui 安装
cnpm i element-ui -S
完整引入
在 main.js 中写入以下内容:
import Vue from 'vue'; import ElementUI from 'element-ui'; import 'element-ui/lib/theme-chalk/index.css'; import App from './App.vue'; Vue.use(ElementUI); new Vue({ el: '#app', render: h => h(App) });
axios
发送
ajax
请求的工具!支持的 请求 快捷 方式
- axios.request(config)
- axios.get(url [,config])
- axios.delete(url [,config])
- axios.head(url [,config])
- axios.post(url [,data [,config]])
- axios.put(url [,data [,config]])
- axios.patch(url [,data [,config]])
注意 当使用别名方法时,不需要在config中指定url,method和data属性。
服务端发送的是 http请求
使用
cnpm install axios --save
安装 在项目目录下在mian.js 中配置 全局 使用
$axios
// 导入 Axios import Axios from 'axios' // axios默认URL前缀: 使用请求时 会自动拼接 Axios.defaults.baseURL = 'https://www.luffycity.com/api/v1'; // 设置 ajax token 请求头 Axios.defaults.headers = {'Authorization': '342c89461d8011e997186014b3cfd1b8', }; Vue.prototype.$axios = Axios;
使用:
在 vue-cli 中 发送
ajax
请求 需要 将 axios 改为this.$axios.get()
GET 请求
// 向具有指定ID的用户发出请求 axios.get('/user?ID=12345') .then(function (response) { console.log('请求成功的返回值'); }) .catch(function (error) { console.log('请求失败的返回值'); }); // 也可以通过 params 对象传递参数 后端从 request.query_params.get() 中获取 id axios.get('/user', {params: {id: 12345} }) .then(function (response) { console.log(response); }) .catch(function (error) { console.log(error); });
POST 请求:
// 发送数据应在 main.js 中设置 请求头 Axios.default.headers = {'Content-Type': 'application/json'}; // 第二个 参数 对象{} 为 post 请求数据 var dict = {name: 'zhangzhiyi', age: 18,} axios.post('/user', dict, // 数据 // 或者在 实例中设置请求头 {headers: {"Content-Type": "application/json; charset=utf-8"}} ) .then(function (response) { console.log(response); }) .catch(function (error) { console.log(error); });
发送 文件 分离项目
<!-- 使用 axios 上传文件时使用 --> <input ref="upp" type="file"> <button v-on:click="up">提交</button> // vue-cli 中 methods: { up() { let form_obj = new FormData(); // 获取DOM 并获取 文件对象 form_obj.append('file', this.$refs.upp.files[0]); console.log(this.$refs.upp.files[0]); this.$axios.post("http://127.0.0.1:80/uploading/", form_obj) .then(function (res) { console.log(res) }) } } // 使用form 表单提交时 <form action="http://127.0.0.1:80/uploading" method="post" enctype="multipart/form-data"> <input ref="upp" type="file"> <button v-on:click="up">提交</button> </form> methods: { up() { // 获取DOM 并获取 文件对象 console.log(this.$refs.upp.files[0]); } }
视图中:
class UploadingView(APIView): """ 文件上传 """ def post(self, request): # 使用 View 时使用 request.FILES 接受文件 APIView 也支持 request.FILES 参数 # file_obj = request.FILES.get('files') # print(file_obj) file_obj = request.data.get('file') print(file_obj) with open(file_obj.name, 'wb') as f: for chunk in file_obj.chunks(): # chunks() 以片段的形式获取 去每一个片段 f.write(chunk) # return HttpResponse("OK") return Response("OK")
并发请求
function getUserAccount() { return axios.get('/user/12345'); } function getUserPermissions() { return axios.get('/user/12345/permissions'); } axios.all([getUserAccount(), getUserPermissions()]) .then(axios.spread(function (acct, perms) { //两个请求现已完成 }));
自定义配置:
您可以使用自定义配置创建axios的新实例。 会与 实例的请求合并
axios.create([config])
var instance = axios.create({ baseURL: 'https://some-domain.com/api/', // 请求的主路径 会自定进行拼接 timeout: 1000, // 超时时间 headers: {'X-Custom-Header': 'foobar'} // 设置请求头 });
请求时的配置
{ // `url`是将用于请求的服务器URL url: '/user', // `method`是发出请求时使用的请求方法 method: 'get', // 默认 // `baseURL`将被添加到`url`前面,除非`url`是绝对的。 // 可以方便地为 axios 的实例设置`baseURL`,以便将相对 URL 传递给该实例的方法。 baseURL: 'https://some-domain.com/api/', // `transformRequest`允许在请求数据发送到服务器之前对其进行更改 // 这只适用于请求方法'PUT','POST'和'PATCH' // 数组中的最后一个函数必须返回一个字符串,一个 ArrayBuffer或一个 Stream transformRequest: [function (data) { // 做任何你想要的数据转换 return data; }], // `transformResponse`允许在 then / catch之前对响应数据进行更改 transformResponse: [function (data) { // Do whatever you want to transform the data return data; }], // `headers`是要发送的自定义 headers headers: {'X-Requested-With': 'XMLHttpRequest'}, // `params`是要与请求一起发送的URL参数 // 必须是纯对象或URLSearchParams对象 params: { ID: 12345 }, // `paramsSerializer`是一个可选的函数,负责序列化`params` // (e.g. https://www.npmjs.com/package/qs, http://api.jquery.com/jquery.param/) paramsSerializer: function(params) { return Qs.stringify(params, {arrayFormat: 'brackets'}) }, // `data`是要作为请求主体发送的数据 // 仅适用于请求方法“PUT”,“POST”和“PATCH” // 当没有设置`transformRequest`时,必须是以下类型之一: // - string, plain object, ArrayBuffer, ArrayBufferView, URLSearchParams // - Browser only: FormData, File, Blob // - Node only: Stream data: { firstName: 'Fred' }, // `timeout`指定请求超时之前的毫秒数。 // 如果请求的时间超过'timeout',请求将被中止。 timeout: 1000, // `withCredentials`指示是否跨站点访问控制请求 // should be made using credentials withCredentials: false, // default // `adapter'允许自定义处理请求,这使得测试更容易。 // 返回一个promise并提供一个有效的响应(参见[response docs](#response-api)) adapter: function (config) { /* ... */ }, // `auth'表示应该使用 HTTP 基本认证,并提供凭据。 // 这将设置一个`Authorization'头,覆盖任何现有的`Authorization'自定义头,使用`headers`设置。 auth: { username: 'janedoe', password: 's00pers3cret' }, // “responseType”表示服务器将响应的数据类型 // 包括 'arraybuffer', 'blob', 'document', 'json', 'text', 'stream' responseType: 'json', // default //`xsrfCookieName`是要用作 xsrf 令牌的值的cookie的名称 xsrfCookieName: 'XSRF-TOKEN', // default // `xsrfHeaderName`是携带xsrf令牌值的http头的名称 xsrfHeaderName: 'X-XSRF-TOKEN', // default // `onUploadProgress`允许处理上传的进度事件 onUploadProgress: function (progressEvent) { // 使用本地 progress 事件做任何你想要做的 }, // `onDownloadProgress`允许处理下载的进度事件 onDownloadProgress: function (progressEvent) { // Do whatever you want with the native progress event }, // `maxContentLength`定义允许的http响应内容的最大大小 maxContentLength: 2000, // `validateStatus`定义是否解析或拒绝给定的promise // HTTP响应状态码。如果`validateStatus`返回`true`(或被设置为`null` promise将被解析;否则,promise将被 // 拒绝。 validateStatus: function (status) { return status >= 200 && status < 300; // default }, // `maxRedirects`定义在node.js中要遵循的重定向的最大数量。 // 如果设置为0,则不会遵循重定向。 maxRedirects: 5, // 默认 // `httpAgent`和`httpsAgent`用于定义在node.js中分别执行http和https请求时使用的自定义代理。 // 允许配置类似`keepAlive`的选项, // 默认情况下不启用。 httpAgent: new http.Agent({ keepAlive: true }), httpsAgent: new https.Agent({ keepAlive: true }), // 'proxy'定义代理服务器的主机名和端口 // `auth`表示HTTP Basic auth应该用于连接到代理,并提供credentials。 // 这将设置一个`Proxy-Authorization` header,覆盖任何使用`headers`设置的现有的`Proxy-Authorization` 自定义 headers。 proxy: { host: '127.0.0.1', port: 9000, auth: : { username: 'mikeymike', password: 'rapunz3l' } }, // “cancelToken”指定可用于取消请求的取消令牌 // (see Cancellation section below for details) cancelToken: new CancelToken(function (cancel) { }) }
其他 http://www.cnblogs.com/libin-1/p/6607945.html