题目 HDU--1394
The inversion number of a given number sequence a1, a2, ..., an is the number of pairs (ai, aj) that satisfy i < j and ai > aj.
For a given sequence of numbers a1, a2, ..., an, if we move the first m >= 0 numbers to the end of the seqence, we will obtain another sequence. There are totally n such sequences as the following:
a1, a2, ..., an-1, an (where m = 0 - the initial seqence)
a2, a3, ..., an, a1 (where m = 1)
a3, a4, ..., an, a1, a2 (where m = 2)
...
an, a1, a2, ..., an-1 (where m = n-1)
You are asked to write a program to find the minimum inversion number out of the above sequences.
Input
The input consists of a number of test cases. Each case consists of two lines: the first line contains a positive integer n (n <= 5000); the next line contains a permutation of the n integers from 0 to n-1.
Output
For each case, output the minimum inversion number on a single line.
Sample Input
10 1 3 6 9 0 8 5 7 4 2
Sample Output
16
题意
多组输入。给定一个数字n,然后第二行输入n个在0--n-1之间的数。在给定的序列中,每次把第一个数移到最后一个位置,求所有序列的逆序数的最小值。
分析
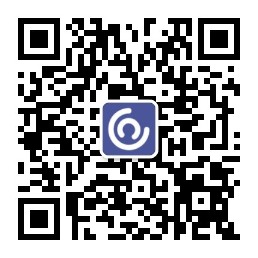
利用线段树求a[i]+1到n-1之间的大于a[i]的个数,统计完初始序列的逆序对数之后,就开始移动。每次把a[i]移动到最后一个位置之前,a[i]后面比a[i]大的有n-a[i]-1个,a[i]后面比a[i]小的有a[i]个,移动后,大于a[i]的全部成了逆序,小于a[i]的全部成了顺序,所以,在原来逆序数的基础上加上n-a[i]-1,再减去a[i],最后跟原来比较。
代码
#include<iostream>
#include<cstdio>
#include<string.h>
using namespace std;
const int N=5005;
int a[N];
struct node{
int left,right;
int sum;
}tree[N<<2];
void pushup(int m)
{
tree[m].sum=tree[m<<1].sum+tree[m<<1|1].sum;
}
void build(int m,int l,int r)
{
tree[m].sum=0;
tree[m].left=l;
tree[m].right=r;
if(l==r)
return ;
int mid=(l+r)>>1;
build(m<<1,l,mid);
build(m<<1|1,mid+1,r);
}
void updata(int m,int id)
{
if(tree[m].left==id&&tree[m].right==id)
{
tree[m].sum=1;//出现过,标为1
return ;
}
int mid=(tree[m].left+tree[m].right)>>1;
if(id<=mid)
updata(m<<1,id);
else
updata(m<<1|1,id);
pushup(m);
}
int query(int m,int l,int r)
{
if(tree[m].left>=l&&tree[m].right<=r)//
{
return tree[m].sum;
}
int mid=(tree[m].left+tree[m].right)>>1;
int sum1=0,sum2=0;
if(l<=mid)
sum1=query(m<<1,l,r);
if(r>mid)
sum2=query(m<<1|1,l,r);
return sum1+sum2;
}
int main()
{
int n,sum;
while(~scanf("%d",&n))
{
if(n==0)
break;
build(1,0,n-1);
sum=0;
for(int i=0;i<n;i++)
{
scanf("%d",&a[i]);
sum += query(1,a[i]+1,n-1);//比a[i]大的数肯定在a[i]+1到n-1之间
updata(1,a[i]);
}
int ans=sum;
for(int i=0;i<n;i++)
{
sum=sum+n-2*a[i]-1;//把a[i]移到最后面,a[i]后面大于a[i]的数有n-a[i]-1个,
ans=min(ans,sum);//a[i]后面比a[i]小的有a[i]个,要加上大的,减去小的
}
printf("%d\n",ans);
}
return 0;
}