版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/u011310942/article/details/88137848
本文同步发表于我的微信公众号,扫一扫文章底部的二维码或在微信搜索 极客导航 即可关注,每个工作日都有文章更新。
一、概况
网络请求可能是每门语言比较重要的一部分了,在Python语言中,虽然有urllib
这样的自带原生网络请求库,但是它的一些API对待开发者好像不怎么友好。So,Requests
的革命开始了,不仅API人性化,更重要的是它支持urllib
所有特性。
Requests
支持HTTP连接保持和连接池,支持使用cookie保持会话,支持文件上传,支持自动确定响应内容的编码,支持国际化的URL
和 POST
数据自动编码。好处不多说了,我们快来体验一下吧。
好像忘记说了一句,Requests的库再牛逼,底层也是urllib
。
二、准备
- 安装requests库
pip3 install requests
三、使用
1、Get请求:
response = requests.get("http://www.baidu.com/")
看到没,对,你没看错,发起GET请求就短短的一行代码。
返回的response
有很多属性,我们来看看:
response.text 返回解码后的字符串,
respones.content 以字节形式(二进制)返回。
response.status_code 响应状态码
response.request.headers 请求的请求头
response.headers 响应头
response.encoding = 'utf-8' 可以设置编码类型
response.encoding 获取当前的编码
response.json() 内置的JSON解码器,以json形式返回,前提返回的内容确保是json格式的,不然解析出错会抛异常
- 添加参数和请求头
import requests
#参数
kw = {'wd':'美女'}
#请求头
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/54.0.2840.99 Safari/537.36"
}
# params 接收一个字典或者字符串的查询参数,
# 字典类型自动转换为url编码,不需要urlencode()
response = requests.get(
"http://www.baidu.com/s?",
params = kw,
headers = headers
)
# 查看响应内容,response.text 返回的是Unicode格式的数据
print (response.text)
# 查看响应内容,response.content返回的字节流数据
print (respones.content)
# 查看完整url地址
print (response.url)
# 查看响应头部字符编码
print (response.encoding)
# 查看响应码
print (response.status_code)
返回的内容如下:
......内容太多,不宜展示
......内容太多,不宜展示
'http://www.baidu.com/s?wd=%E9%95%BF%E5%9F%8E'
'utf-8'
200
注意
- 使用
response.text
时,Requests
会基于 HTTP 响应的文本编码自动解码响应内容,大多数 Unicode 字符集都能被无缝地解码。 - 使用
response.content
时,返回的是服务器响应数据的原始二进制字节流,可以用来保存图片等二进制文件。
比如:
import requests
response = requests.get("http://www.sina.com")
print(response.request.headers)
print(response.content.decode('utf-8'))
结果:
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>新浪首页</title>
<meta name="keywords" content="新浪,新浪网,SINA,sina,sina.com.cn,新浪首页,门户,资讯" />
...
如果用response.text
可能会出现乱码情况,比如:
import requests
response = requests.get("http://www.sina.com")
print(response.text)
结果:
扫描二维码关注公众号,回复:
5950951 查看本文章
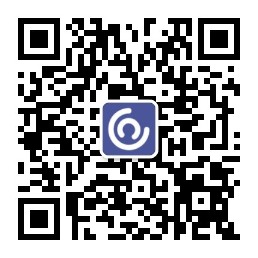
<!DOCTYPE html>
<!-- [ published at 2017-06-09 15:18:10 ] -->
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>新浪首页</title>
<meta name="keywords" content="新浪,新浪网,SINA,sina,sina.com.cn,新浪首页,门户,资讯" />
<meta name="description" content="新浪网为全çƒç”¨æˆ·24å°æ—¶æä¾›å…¨é¢åŠæ—¶çš„䏿–‡èµ„讯,内容覆盖国内外çªå‘新闻事件ã€ä½“å›èµ›äº‹ã€å¨±ä¹æ—¶å°šã€äº§ä¸šèµ„讯ã€å®žç”¨ä¿¡æ¯ç‰ï¼Œè®¾æœ‰æ–°é—»ã€ä½“育ã€å¨±ä¹ã€è´¢ç»ã€ç§‘æŠ€ã€æˆ¿äº§ã€æ±½è½¦ç‰30多个内容频é“ï¼ŒåŒæ—¶å¼€è®¾åšå®¢ã€è§†é¢‘ã€è®ºå›ç‰è‡ªç”±äº’动交æµç©ºé—´ã€‚" />
<link rel="mask-icon" sizes="any" href="//www.sina.com.cn/favicon.svg" color="red">
- 原因
当收到一个响应时,Requests
会猜测响应的编码方式,用于在你调用response.text
方法时对响应进行解码。Requests
首先在 HTTP 头部检测是否存在指定的编码方式,如果不存在,则会使用 chardet.detect
来尝试猜测编码方式(存在误差),所以更推荐 response.content.deocde()
2、Post请求:
网站登录和注册等功能一般都是通过post请求方式做的,学会用Requests
发起post请求也是爬取需要登录网站的第一步。
import requests
#测试请求地址
req_url = "https://httpbin.org/post"
#表单数据
formdata = {
'username': 'test',
'password': '123456',
}
#添加请求头
req_header = {
'User-Agent':'Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/67.0.3396.99 Safari/537.36',
}
#发起请求
response = requests.post(
req_url,
data = formdata,
headers = req_header
)
print (response.text)
# 如果是json文件可以直接显示
#print (response.json())
结果:
{
"args": {},
"data": "",
"files": {},
"form": {
"password": "123456",
"username": "test"
},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip, deflate",
"Content-Length": "29",
"Content-Type": "application/x-www-form-urlencoded",
"Host": "httpbin.org",
"User-Agent": "Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/67.0.3396.99 Safari/537.36"
},
"json": null,
"origin": "223.72.76.90, 223.72.76.90",
"url": "https://httpbin.org/post"
}
- 上传文件
url = 'https://httpbin.org/post'
files = {'file': open('image.png', 'rb')}
response = requests.post(url, files=files)
print(response.text)
结果:
{
"args": {},
"data": "",
"files": {
"file": "....内容太多,不宜展示"
},
"form": {},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip, deflate",
"Content-Length": "27597",
"Content-Type": "multipart/form-data; boundary=16afeeccbaec949570677ca271dc2d0c",
"Host": "httpbin.org",
"User-Agent": "python-requests/2.21.0"
},
"json": null,
"origin": "223.72.76.90, 223.72.76.90",
"url": "https://httpbin.org/post"
}
四、总结
以上是Requests
最基本的Get请求和Post请求,语法比较简单,这也是学会爬虫的第一步。加油吧!骚年。
欢迎关注我的公众号,我们一起学习。