什么是迁移学习
迁移学习,就是将一个问题上训练好的模型通过简单的调整使其适用于一个新的问题。本文将介绍如何利用在ImageNet数据集上训练的Inception-v3模型来解决一个新的图像分类问题。
迁移学习的其中一种思路是保留训练好的Inception-v3模型中的所有卷积层的参数,只是替换最后一层全连接层。那么我们只需要训练最后的这一层全连接成就好了。
那么如何训练这最后一层全连接层呢?
首先声明一个概念:
在Inception-v3最后一层全连接层之前的网络称之为瓶颈层(bottleneck)
我们是先将一张图片输入到瓶颈层,瓶颈层输出一个特征向量,我们就是用这个特征向量来训练新的全连接层。
也就是说实际上我们只训练了一个一层的神经网络,在训练完成后,与原来的inception-v3的一部分拼凑起来,形成一个新的网络。
下面图是原先的inception-v3网络结构
重新设计后的inception-v3网络:
没错,网络结构没有发生变化,迁移学习并不会改变网络的结构,迁移学习仅仅只是让网络的参数发生变化,使得网络更适合解决新的图片分类问题。
与训练模型和数据集准备
数据集
wget http://download.tensorflow.org/example_images/flower_photos.tgz
tar xzf flower_photos.tgz
该数据集解压后的文件夹包含5个子文件夹,每一个子文件夹的名称为一种花的名名称。平均每一种话有734张图片,每一张图片都是RGB色彩模式的,大小也不相同。
预训练模型
wget http://storage.googleapis.com/download.tensorflow.org/models/inception_dec_2015.zip
unzip inception_dec_2015.zip
这个模型是经过ImageNet数据集训练过的inception-v3模型,迁移学习实际上就是在别人训练好的模型上进行微调(fine-tuning),但不是所有训练集训练出来的模型都适合对你要解决的问题进行迁移学习的,那么什么样的训练集训练出来的模型适合对你要解决的问题进行fine-tuning呢?
训练集合之间的问题相似即可。
ImageNet数据集是为了促进计算机图像识别技术的发展而设立的一个大型图像数据集。其图片数量最多,分辨率最高,含有的类别更多,有上千个图像类别。经过该集合训练出来的网络本身已近对1000种物体具有很好的分类效果了,也就是说能很好的区分1000种物体的特征,那么用它在进行一个5分类的迁移学习是在适合不过的了。
如何重新训练网络
这里有两种思路:
第一种是网络结构不变,将最后一层(1000个节点)改为5个节点,重新训练整个网络,包括瓶颈层的参数。
第二种是保留瓶颈层的网络参数不变,只训练瓶颈成到最后5个节点的全连接层的参数。
在不同的情况使用不同的迁移学习策略,本文主要讲第二种。
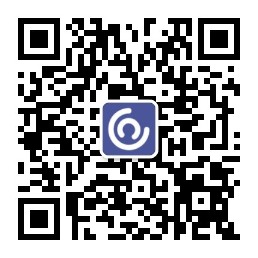
整体实现代码:
import tensorflow as tf
import glob
import os.path
import random
import numpy as np
import tensorflow as tf
from tensorflow.python.platform import gfile
# Inception-v3 模型瓶颈层的节点个数
BOTTLENECK_TENSOR_SIZE = 2048
# 模型张量所对应的名称
BOTTLENECK_TENSOR_NAME = 'pool_3/_reshape:0' # 瓶颈层
JPEG_DATA_TENSOR_NAME = 'DecodeJpeg/contents:0' # 输入层
MODEL_DIR = '/path/to/model' # 下载好的模型存放目录
MODEL_FILE = 'classify_image_graph_def.pb' # 模型文件
CACHE_DIR = '/tmp/bottleneck' # 图片经过瓶颈层之后输出的特征向量保存于文件之后的目录地址
INPUT_DATA = '/path/to/flower_data' # 训练图片文件夹
VALIDATION_PERCENTAGE = 10 # 数据百分之10用于验证
TEST_PERCENTAGE = 10 # 数据百分之10用于测试, 剩下的百分之80用于训练
LEARNING_RATE = 0.01 # 学习率
STEPS = 4000 # 训练迭代次数
BATCH = 100
def create_image_lists(testing_precentage, validation_percentage) :
result = {}
sub_dirs = [x[0] for x in os.walk(INPUT_DATA)] # 获取训练数据目录下的所有子文件夹
is_root_dir = True
for sub_dir in sub_dirs:
if is_root_dir: # 得到的第一个目录是当前目录,不需要考虑
is_root_dir = False
continue
extensions = ['jpg', 'jpge', 'JPG', 'JPGE']
file_list = []
dir_name = os.path.basename(sub_dir) # 返回最后一个'/'的目录名
for extension in extensions:
file_glob = os.path.join(INPUT_DATA, dir_name, '*.' + extension) # 文件路径拼接
file_list.extend(glob.glob(file_glob)) # 把所有符合要求的文件加入到file_lsit列表
if not file_list : continue
label_name = dir_name.lower() # 大写转小写,花名
training_images = []
testing_images = []
validation_images =[]
for file_name in file_list:
base_name = os.path.basename(file_name) # 图片名称
# 随机将数据分到训练集, 测试集和验证集
chance = np.random.randint(100)
if chance < validation_percentage:
validation_images.append(base_name)
if chance < (testing_precentage + validation_percentage):
testing_images.append(base_name)
else:
training_images.append(base_name)
# 当前类别的数据放入结果字典
result[label_name] = {
'dir' : dir_name,
'training' : training_images,
'testing' : testing_images,
'validation' : validation_images
}
# 返回整理好的数据
return result
# 这个函数通过类别名称, 所属数据集和图片编码获取一张图片特征向量的地址
def get_image_path(image_lists, image_dir, label_name, index, category) :
label_lists = image_lists[label_name]
category_list = label_lists[category] # category表示的是3种数据集的其中之一 training,testing,validation
mod_index = index % len(category_list)
base_name = category_list[mod_index]
sub_dir = label_lists['dir']
full_path = os.path.join(image_dir, sub_dir, base_name)
return full_path
# 这个函数通过类别名称,所属数据集和图片编号获取经过Inception-v3模型处理之后的特征向量文件地址
def get_bottleneck_path(image_lists, lable_name, index, category):
return get_image_path(image_lists, CACHE_DIR, lable_name, index, category) + '.txt' # 特征向量文件是.txt结尾的
# 这个函数使用加载训练好的Incepyion-v3 模型处理一张图片,得到这个图片的特征向量
def run_bottleneck_on_image(sess, image_data, image_data_tensor, bottleneck_tensor):
bottleneck_values = sess.run(bottleneck_tensor, {image_data_tensor: image_data})
bottleneck_values = np.squeeze(bottleneck_values) # 压缩成一维的向量
return bottleneck_values
# 这个函数获取一张图片经过Inception-v3模型处理之后的特征向量。这个函数会先试图寻找已经计算并且保存下来的特征向量,如果找不到则先计算这个特张向量,然后保存到文件
def get_or_create_bottleneck(sess, image_lists, label_name, index, category, jpeg_data_tensor, bottleneck_tensor):
label_list = image_lists[label_name]
sub_dir = label_list['dir']
sub_dir_path = os.path.join(CACHE_DIR, sub_dir)
if not os.path.exists(sub_dir_path):
os.makedirs(sub_dir_path)
bottleneck_path = get_bottleneck_path(image_lists, label_name, index, category)
# 特征向量文件不存在,则通过Inceptin-v3模型来计算特征向量,将计算的结果存入文件
if not os.path.exists(bottleneck_path):
image_path = get_image_path(image_lists, INPUT_DATA, label_name, index, category)
image_data = gfile.FastGFile(image_path, 'rb').read() # 读取图片,准备输入到InceptionV3网络(没有改变图像大小)
# 通过Inception-v3模型计算特征向量
bottleneck_values = run_bottleneck_on_image(sess, image_data, jpeg_data_tensor, bottleneck_tensor)
bottleneck_string = ','.join(str(x) for x in bottleneck_values)
with open(bottleneck_path, 'w') as bottleneck_file:
bottleneck_file.write(bottleneck_string)
# 否则直接从文件中获取图片相应的特征向量
else:
with open(bottleneck_path, 'r') as bottleneck_file:
bottleneck_string = bottleneck_file.read()
bottleneck_values = [float(x) for x in bottleneck_string.split(',')]
return bottleneck_values
# 这个函数随机获取一个batch的图片作为训练数据
def get_random_cached_bottlenecks(sess, n_classes, image_lists, how_many, category, jpeg_data_tensor, bottleneck_tensor):
bottlenecks = []
ground_truths = []
for _ in range(how_many):
lable_index = random.randrange(n_classes)
lable_name = list(image_lists.keys(0))[lable_index]
image_index = random.randrange(65536)
bottleneck = get_or_create_bottleneck(sess, image_lists, lable_name, image_index, category, jpeg_data_tensor, bottleneck_tensor)
ground_truth = np.zeros(n_classes, dtype=np.float32)
ground_truth[lable_index] = 1.0
bottlenecks.append(bottleneck)
ground_truths.append(ground_truth)
return bottlenecks, ground_truths
# 这个函数获取全部的测试数据,在最终测试的时候需要在所有的测试数据上计算正确率(不是随机获取一个batch,而是全部数据)
def get_test_bottlenecks(sess, image_lists, n_classes, jpeg_data_tensor, bottleneck_tensor):
bottlenecks = []
ground_truths = []
label_name_list = list(image_lists.keys())
# 枚举所有的类别和每个类别的测试图片
for label_index, label_name in enumerate(image_lists):
category = 'testing'
for index, unused_base_name in enumerate(image_lists[label_name][category]):
bottleneck = get_or_create_bottleneck(sess, image_lists, label_name, index, category, jpeg_data_tensor, bottleneck_tensor)
ground_truth = np.zeros(n_classes, dtype=np.float32)
ground_truth[label_index] = 1.0
bottlenecks.append(bottleneck)
ground_truth.append(ground_truth)
return bottlenecks, ground_truths
def main():
# 读取所有图片
image_lists = create_image_lists(TEST_PERCENTAGE, VALIDATION_PERCENTAGE)
n_classes =len(image_lists.keys()) # 5种花,该值为5
# 载入已有的模型
with gfile.FastGFile(os.path.join(MODEL_DIR, MODEL_FILE), 'rb') as f:
graph_def = tf.GraphDef()
graph_def.ParseFromString(f.read())
bottleneck_tensor, jpeg_data_tensor = tf.import_graph_def(graph_def, return_elements=[BOTTLENECK_TENSOR_NAME, JPEG_DATA_TENSOR_NAME])
bottleneck_input = tf.placeholder(tf.float32, [None, BOTTLENECK_TENSOR_SIZE], name='BottleneckInputPlaceholder') # 特征化过后的欲训练数据
ground_truth_input = tf.placeholder(tf.float32, [None, n_classes], name='GroundTruthInput') # 对应的真实结果
# 定义正向传播
with tf.name_scope('final_training_ops'):
weights = tf.Variable(tf.truncated_normal([BOTTLENECK_TENSOR_SIZE, n_classes], stddev=0.001))
biases = tf.Variable(tf.zeros(n_classes))
logits = tf.matmul(bottleneck_input, weights) + biases
final_tensor = tf.nn.softmax(logits)
# 定义损失函数
cross_entropy = tf.nn.softmax_cross_entropy_with_logits(logits, ground_truth_input)
cross_entropy_mean = tf.reduce_mean(cross_entropy)
# 定义反向传播
train_step = tf.train.GradientDescentOptimizer(LEARNING_RATE).minimize(cross_entropy_mean)
# 计算正确率
with tf.name_scope('evaluation'):
correct_prediction = tf.equal(tf.argmax(final_tensor, 1), tf.argmax(ground_truth_input, 1))
evaluation_step = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
# 图定义结束
with tf.Session() as sess:
init = tf.initialize_all_variables()
sess.run(init)
for i in range(STEPS):
# 每次获取一个batch的训练数据
train_bottlenecks, train_ground_truth = get_random_cached_bottlenecks(sess, n_classes, image_lists, BATCH, 'training', jpeg_data_tensor, bottleneck_tensor)
sess.run(train_step, feed_dict={bottleneck_input:train_bottlenecks, ground_truth_input: train_ground_truth})
# 在验证数据上测试正确率
if i%100 == 0 or i+1 == STEPS:
validation_bottlenecks, validation_ground_truth = get_random_cached_bottlenecks(sess, n_classes, image_lists, BATCH, 'validation', jpeg_data_tensor, bottleneck_tensor)
validation_accuracy = sess.run(train_step, feed_dict={bottleneck_input:validation_bottlenecks, ground_truth_input:validation_ground_truth})
print(validation_accuracy * 100)
# 在最后的测试数据上测试正确率
test_bottlenecks, test_ground_truth = get_test_bottlenecks(sess, image_lists, n_classes, jpeg_data_tensor, bottleneck_tensor)
test_accuracy = sess.run(evaluation_step, feed_dict={bottleneck_input:test_bottlenecks, ground_truth_input:test_ground_truth})
print(test_accuracy)
这里,直接用了原始图片输入到瓶颈层,然后输出特征向量,读者可以对其进行优化,将图像进行预处理,这样能很好的提高准确度。
代码中,对训练数据的管理比较混乱,可读性不高,且难横向扩展,可以用TFRecord进行数据管理。
关键代码:
这里的关键代码就只有两部分,第一部分是新的全连接层的前向传播,第二部分是如何将图片输入到瓶颈层然后获取特征输出向量。
- 新的全连接层的前向传播
# 定义正向传播
with tf.name_scope('final_training_ops'):
weights = tf.Variable(tf.truncated_normal([BOTTLENECK_TENSOR_SIZE, n_classes], stddev=0.001))
biases = tf.Variable(tf.zeros(n_classes))
logits = tf.matmul(bottleneck_input, weights) + biases
final_tensor = tf.nn.softmax(logits)
- 如何将图片输入到瓶颈层然后获取特征输出向量
先从下载好的模型中获取到瓶颈层的输入和输出张量:
bottleneck_tensor, jpeg_data_tensor = tf.import_graph_def(graph_def, return_elements=[BOTTLENECK_TENSOR_NAME, JPEG_DATA_TENSOR_NAME])
将数据从输入层输入,获取瓶颈层输出:
bottleneck_values = sess.run(bottleneck_tensor, {image_data_tensor: image_data})
bottleneck_values = np.squeeze(bottleneck_values) # 压缩成一维的向量
可以看到关键代码就那么一点,其他的大部分都是训练数据的管理和调用,上面代码数据的管理可读性很差,读者可以改为用TFRecord进行优化。