tfrecords
由于有个5W张图片的训练集,如果是placeholder的方式来把数据喂给graph的话,那可真蠢。。所以不如用tensorflow官方推荐的tfrecords来进行IO。这种IO方式主要是两步:
1.先把数据dump成tfrecords文件
2.用队列来把数据喂给模型
写数据
tfrecords文件是一种二进制文件,虽然如果把这个二进制文件打开会发现完全没有可读性,但是tensorflow在读写这种二进制文件的时候底层用的是google推行的protocol buffer(协议内存),这就比较舒服了,完全可以把二进制文件当成黑盒子,代码层面逻辑正确的话就没问题。
刚刚说到tfrecords其实是protocol buffer,那不如捋一捋它的protocol。首先可以想象一下数据集是由很多条数据组成的,每条数据是由很多个字段(特征)组成的。所以tfrecords中用example来表示一条数据,用features来表示一条数据中的特征。
那么就可以顺藤摸瓜了,来看看example.proto和feature.proto长啥样。
example.proto
// Protocol messages for describing input data Examples for machine learning
// model training or inference.
syntax = "proto3";
import "tensorflow/core/example/feature.proto";
option cc_enable_arenas = true;
option java_outer_classname = "ExampleProtos";
option java_multiple_files = true;
option java_package = "org.tensorflow.example";
package tensorflow;
// An Example is a mostly-normalized data format for storing data for
// training and inference. It contains a key-value store (features); where
// each key (string) maps to a Feature message (which is oneof packed BytesList,
// FloatList, or Int64List). This flexible and compact format allows the
// storage of large amounts of typed data, but requires that the data shape
// and use be determined by the configuration files and parsers that are used to
// read and write this format. That is, the Example is mostly *not* a
// self-describing format. In TensorFlow, Examples are read in row-major
// format, so any configuration that describes data with rank-2 or above
// should keep this in mind. For example, to store an M x N matrix of Bytes,
// the BytesList must contain M*N bytes, with M rows of N contiguous values
// each. That is, the BytesList value must store the matrix as:
// .... row 0 .... .... row 1 .... // ........... // ... row M-1 ....
//
// An Example for a movie recommendation application:
// features {
// feature {
// key: "age"
// value { float_list {
// value: 29.0
// }}
// }
// feature {
// key: "movie"
// value { bytes_list {
// value: "The Shawshank Redemption"
// value: "Fight Club"
// }}
// }
// feature {
// key: "movie_ratings"
// value { float_list {
// value: 9.0
// value: 9.7
// }}
// }
// feature {
// key: "suggestion"
// value { bytes_list {
// value: "Inception"
// }}
// }
// # Note that this feature exists to be used as a label in training.
// # E.g., if training a logistic regression model to predict purchase
// # probability in our learning tool we would set the label feature to
// # "suggestion_purchased".
// feature {
// key: "suggestion_purchased"
// value { float_list {
// value: 1.0
// }}
// }
// # Similar to "suggestion_purchased" above this feature exists to be used
// # as a label in training.
// # E.g., if training a linear regression model to predict purchase
// # price in our learning tool we would set the label feature to
// # "purchase_price".
// feature {
// key: "purchase_price"
// value { float_list {
// value: 9.99
// }}
// }
// }
//
// A conformant Example data set obeys the following conventions:
// - If a Feature K exists in one example with data type T, it must be of
// type T in all other examples when present. It may be omitted.
// - The number of instances of Feature K list data may vary across examples,
// depending on the requirements of the model.
// - If a Feature K doesn't exist in an example, a K-specific default will be
// used, if configured.
// - If a Feature K exists in an example but contains no items, the intent
// is considered to be an empty tensor and no default will be used.
message Example {
Features features = 1;
};
// A SequenceExample is an Example representing one or more sequences, and
// some context. The context contains features which apply to the entire
// example. The feature_lists contain a key, value map where each key is
// associated with a repeated set of Features (a FeatureList).
// A FeatureList thus represents the values of a feature identified by its key
// over time / frames.
//
// Below is a SequenceExample for a movie recommendation application recording a
// sequence of ratings by a user. The time-independent features ("locale",
// "age", "favorites") describing the user are part of the context. The sequence
// of movies the user rated are part of the feature_lists. For each movie in the
// sequence we have information on its name and actors and the user's rating.
// This information is recorded in three separate feature_list(s).
// In the example below there are only two movies. All three feature_list(s),
// namely "movie_ratings", "movie_names", and "actors" have a feature value for
// both movies. Note, that "actors" is itself a bytes_list with multiple
// strings per movie.
//
// context: {
// feature: {
// key : "locale"
// value: {
// bytes_list: {
// value: [ "pt_BR" ]
// }
// }
// }
// feature: {
// key : "age"
// value: {
// float_list: {
// value: [ 19.0 ]
// }
// }
// }
// feature: {
// key : "favorites"
// value: {
// bytes_list: {
// value: [ "Majesty Rose", "Savannah Outen", "One Direction" ]
// }
// }
// }
// }
// feature_lists: {
// feature_list: {
// key : "movie_ratings"
// value: {
// feature: {
// float_list: {
// value: [ 4.5 ]
// }
// }
// feature: {
// float_list: {
// value: [ 5.0 ]
// }
// }
// }
// }
// feature_list: {
// key : "movie_names"
// value: {
// feature: {
// bytes_list: {
// value: [ "The Shawshank Redemption" ]
// }
// }
// feature: {
// bytes_list: {
// value: [ "Fight Club" ]
// }
// }
// }
// }
// feature_list: {
// key : "actors"
// value: {
// feature: {
// bytes_list: {
// value: [ "Tim Robbins", "Morgan Freeman" ]
// }
// }
// feature: {
// bytes_list: {
// value: [ "Brad Pitt", "Edward Norton", "Helena Bonham Carter" ]
// }
// }
// }
// }
// }
//
// A conformant SequenceExample data set obeys the following conventions:
//
// Context:
// - All conformant context features K must obey the same conventions as
// a conformant Example's features (see above).
// Feature lists:
// - A FeatureList L may be missing in an example; it is up to the
// parser configuration to determine if this is allowed or considered
// an empty list (zero length).
// - If a FeatureList L exists, it may be empty (zero length).
// - If a FeatureList L is non-empty, all features within the FeatureList
// must have the same data type T. Even across SequenceExamples, the type T
// of the FeatureList identified by the same key must be the same. An entry
// without any values may serve as an empty feature.
// - If a FeatureList L is non-empty, it is up to the parser configuration
// to determine if all features within the FeatureList must
// have the same size. The same holds for this FeatureList across multiple
// examples.
//
// Examples of conformant and non-conformant examples' FeatureLists:
//
// Conformant FeatureLists:
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.5 ] } }
// feature: { float_list: { value: [ 5.0 ] } } }
// } }
//
// Non-conformant FeatureLists (mismatched types):
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.5 ] } }
// feature: { int64_list: { value: [ 5 ] } } }
// } }
//
// Conditionally conformant FeatureLists, the parser configuration determines
// if the feature sizes must match:
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.5 ] } }
// feature: { float_list: { value: [ 5.0, 6.0 ] } } }
// } }
//
// Conformant pair of SequenceExample
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.5 ] } }
// feature: { float_list: { value: [ 5.0 ] } } }
// } }
// and:
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.5 ] } }
// feature: { float_list: { value: [ 5.0 ] } }
// feature: { float_list: { value: [ 2.0 ] } } }
// } }
//
// Conformant pair of SequenceExample
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.5 ] } }
// feature: { float_list: { value: [ 5.0 ] } } }
// } }
// and:
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { }
// } }
//
// Conditionally conformant pair of SequenceExample, the parser configuration
// determines if the second feature_lists is consistent (zero-length) or
// invalid (missing "movie_ratings"):
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.5 ] } }
// feature: { float_list: { value: [ 5.0 ] } } }
// } }
// and:
// feature_lists: { }
//
// Non-conformant pair of SequenceExample (mismatched types)
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.5 ] } }
// feature: { float_list: { value: [ 5.0 ] } } }
// } }
// and:
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { int64_list: { value: [ 4 ] } }
// feature: { int64_list: { value: [ 5 ] } }
// feature: { int64_list: { value: [ 2 ] } } }
// } }
//
// Conditionally conformant pair of SequenceExample; the parser configuration
// determines if the feature sizes must match:
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.5 ] } }
// feature: { float_list: { value: [ 5.0 ] } } }
// } }
// and:
// feature_lists: { feature_list: {
// key: "movie_ratings"
// value: { feature: { float_list: { value: [ 4.0 ] } }
// feature: { float_list: { value: [ 5.0, 3.0 ] } }
// } }
message SequenceExample {
Features context = 1;
FeatureLists feature_lists = 2;
};
feature.proto
syntax = "proto3";
option cc_enable_arenas = true;
option java_outer_classname = "FeatureProtos";
option java_multiple_files = true;
option java_package = "org.tensorflow.example";
package tensorflow;
// Containers to hold repeated fundamental values.
message BytesList {
repeated bytes value = 1;
}
message FloatList {
repeated float value = 1 [packed = true];
}
message Int64List {
repeated int64 value = 1 [packed = true];
}
// Containers for non-sequential data.
message Feature {
// Each feature can be exactly one kind.
oneof kind {
BytesList bytes_list = 1;
FloatList float_list = 2;
Int64List int64_list = 3;
}
};
message Features {
// Map from feature name to feature.
map<string, Feature> feature = 1;
};
// Containers for sequential data.
//
// A FeatureList contains lists of Features. These may hold zero or more
// Feature values.
//
// FeatureLists are organized into categories by name. The FeatureLists message
// contains the mapping from name to FeatureList.
//
message FeatureList {
repeated Feature feature = 1;
};
message FeatureLists {
// Map from feature name to feature list.
map<string, FeatureList> feature_list = 1;
};
看看这两个proto就能YY出他们的关系大概可以表示成example{features:{‘feature1’:xxx, ‘feature2’:yyy, …, ‘featuren’:23333}}。
捋清楚关系后,就可以想一个问题了。我的数据是一张张的图片,那最原始的特征就是像素,所以可以尝试把图的像素看成是字节快然后序列化成字符串来表示一张图。所以就有了下面的代码:
#转换成records
def conver_to_tfrecords(data_set, label_set, name):
#proto里提到的int64list
def _int64_feature(value):
return tf.train.Feature(int64_list=tf.train.Int64List(value=[value]))
#proto里提到的byteslist
def _bytes_feature(value):
return tf.train.Feature(bytes_list=tf.train.BytesList(value=[value]))
print('正在转换成tfrecords', name)
#实例化tfrecord的writer,说白了就是用来写文件的对象
writer = tf.python_io.TFRecordWriter(name)
num_examples = len(data_set)
for index in range(num_examples):
image = data_set[index]
height = image.shape[0]
width = image.shape[1]
#把像素转成字符串
image_raw = image.tostring()
label = label_set[index]
#把onehot后的label转成字符串
label_raw = label_to_one_hot(label).tostring()
#构建example,每个examp由图的高,宽,label, 像素组成
example = tf.train.Example(features=tf.train.Features(feature={
'height': _int64_feature(height),
'width': _int64_feature(width),
'label_raw': _bytes_feature(label_raw),
'image_raw': _bytes_feature(image_raw)}))
#把example序列化成字符串并写到名字为name的文件中
writer.write(example.SerializeToString())
writer.close()
print('转换完毕!')
这个时候,数据已经全部写到tfrecords文件中了。那接下来要干的事情就是用队列读数据。用队列读数据的好处是可以主线程用来训练模型,子线程用来从队列里拿数据,写过代码的都知道这样做有啥好处。。。
读数据
那要用队列,首先肯定是要创建个队列,那创建队列我这里用的tf.train.string_input_producer,因为我的图像和label都序列化成了字符串。
那有队列之后我肯定是要从队列里拿数据啦!!所以就会有下面的代码:
def read_and_decode(filename_queue, img_height=IMG_HEIGHT, img_width=IMG_WIDTH, chars_num=CHAR_NUM, classes_num=len(CHAR_SET)):
#实例化tfrecord的reader,用来读数据的对象
reader = tf.TFRecordReader()
#从创建好的字符串队列中读数据,现在还是二进制的字符串数据
_, serialized_example = reader.read(filename_queue)
#解析二进制数据,PS:我写tfrecords的时候图和label是string,所以我解析的时候也要是string
features = tf.parse_single_example(
serialized_example,
features={
'image_raw': tf.FixedLenFeature([], tf.string),
'label_raw': tf.FixedLenFeature([], tf.string),
})
#这里相当于把string转成float32,因为像素值不可能是字符嘛~~~
image = tf.decode_raw(features['image_raw'], tf.float32)
image.set_shape([img_height * img_width])
image = tf.cast(image, tf.float32) * (1.0 / 255)-0.5
reshape_image = tf.reshape(image, [img_height, img_width, 1])
label = tf.decode_raw(features['label_raw'], tf.float32)
label.set_shape([chars_num * classes_num])
reshape_label = tf.reshape(label, [chars_num, classes_num])
return tf.cast(reshape_image, tf.float32), tf.cast(reshape_label, tf.float32)
def inputs(train, batch_size, epoch):
filename = os.path.join('./', TRAIN_RECORDS_NAME if train else TRAIN_VALIDATE_NAME)
with tf.name_scope('input'):
filename_queue = tf.train.string_input_producer([filename], num_epochs=epoch)
image, label = read_and_decode(filename_queue)
#如果现在是训练,那我会打乱顺序再一个一个batch的拿数据,如果是验证那我就按顺序那batch
if train:
images, sparse_labels = tf.train.shuffle_batch([image, label], batch_size=batch_size, num_threads=6, capacity=2000 + 3 * batch_size, min_after_dequeue=2000)
else:
images, sparse_labels = tf.train.batch([image, label], batch_size=batch_size, num_threads=6, capacity=2000 + 3 * batch_size)
return images, sparse_labels
代码的意思已经写成注释了,不过要注意的是,tensorflow要执行graph需要session,所以你需要用session来run你的inputs才会真正的让队列里真正的有数据。至于怎么run起这个队列,后面再来哔哔。今天就到这里。下一篇估计会记录一下整个CNN怎么搭的。
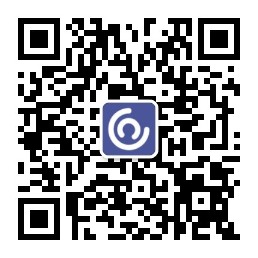