cc.Class({
extends: cc.Component,
properties: {
startBtn: {
default: null, // The default value will be used only when the component attachin // to a node for the first time
type: cc.Button, // optional, default is typeof default
visible: true, // optional, default is true
displayName: 'startBtn', // optional
},
wheelSp: {
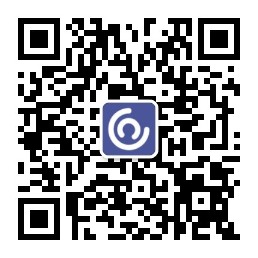
default: null,
type: cc.Sprite
},
maxSpeed: {
default: 5,
type: cc.Float,
max: 15,
min: 2,
},
duration: {
default: 3,
type: cc.Float,
max: 5,
min: 1,
tooltip: "减速前旋转时间"
},
acc: {
default: 0.1,
type: cc.Float,
max: 0.2,
min: 0.01,
tooltip: "加速度"
},
targetID: {
default: 0,
type: cc.Integer,
tooltip: "指定结束时的齿轮"
},
springback: {
default: false,
tooltip: "旋转结束是否回弹"
},
effectAudio: {
default: null,
url: cc.AudioClip
}
},
// use this for initialization
onLoad: function () {
cc.log("....onload");
this.wheelState = 0;
this.curSpeed = 0;
this.spinTime = 0; //减速前旋转时间
this.gearNum = 6;
this.defaultAngle = 360 / 150 / 2; //修正默认角度
this.gearAngle = 360 / this.gearNum; //每个齿轮的角度
this.wheelSp.node.rotation = this.defaultAngle;
this.finalAngle = 0; //最终结果指定的角度
this.effectFlag = 0; //用于音效播放
// if (!cc.sys.isBrowser) {
// cc.loader.loadRes('Sound/game_turntable', function (err, res) { if (err) { cc.log('...err:' + err); } });
// }
this.startBtn.node.on(cc.Node.EventType.TOUCH_END, function (event) {
// this.targetID = Math.floor(Math.random() * 6 + 1);
this.targetID = this.myRandom();
cc.log("begin spin");
if (this.wheelState !== 0) {
return;
}
this.decAngle = 2 * 360; // 减速旋转两圈
this.wheelState = 1;
this.curSpeed = 0;
this.spinTime = 0;
// var act = cc.rotateTo(10, 360*10);
// this.wheelSp.node.runAction(act.easing(cc.easeSineInOut()));
}.bind(this));
},
start: function () {
// cc.log('....start');
},
getRandom: function (start, end) {
var length = end - start;
var num = parseInt(Math.random() * (length) + start);
return num;
},
/**
* 谢谢参与25%,1张30%,2张30%,3张4%,5张4% 10张4%
*/
myRandom: function () {
var rand = Math.random();
if (rand < 0.3) return 2;//1张30%,2张30%
if (rand < 0.6) return 3;//1张30%,2张30%
if (rand < 0.88) return 1;//谢谢参与28%
if (rand < 0.92) return 4;//谢谢参与4%
if (rand < 0.96) return 5;//谢谢参与4%
return 6;//谢谢参与4%
},
caculateFinalAngle: function (targetID) {
this.finalAngle = 360 - this.targetID * this.gearAngle + this.defaultAngle;
if (this.springback) {
this.finalAngle += this.gearAngle;
}
},
// called every frame, uncomment this function to activate update callback
update: function (dt) {
if (this.wheelState === 0) {
return;
}
// 播放音效有可能卡
this.effectFlag += this.curSpeed;
if (!cc.sys.isBrowser && this.effectFlag >= this.gearAngle) {
// if (this.audioID) {
// cc.audioEngine.pauseEffect(this.audioID);
// }
// // this.audioID = cc.audioEngine.playEffect(this.effectAudio,false);
// this.audioID = cc.audioEngine.playEffect(cc.url.raw('resources/sound/game_XXX.mp3'));
this.effectFlag = 0;
}
if (this.wheelState == 1) {
// cc.log('....加速,speed:' + this.curSpeed);
this.spinTime += dt;
this.wheelSp.node.rotation = this.wheelSp.node.rotation + this.curSpeed;
if (this.curSpeed <= this.maxSpeed) {
this.curSpeed += this.acc;
}
else {
if (this.spinTime < this.duration) {
return;
}
// cc.log('....开始减速');
//设置目标角度
this.finalAngle = 360 - this.targetID * this.gearAngle + this.defaultAngle;
this.maxSpeed = this.curSpeed;
if (this.springback) {
this.finalAngle += this.gearAngle;
}
this.wheelSp.node.rotation = this.finalAngle;
this.wheelState = 2;
}
}
else if (this.wheelState == 2) {
// cc.log('......减速');
var curRo = this.wheelSp.node.rotation; //应该等于finalAngle
var hadRo = curRo - this.finalAngle;
this.curSpeed = this.maxSpeed * ((this.decAngle - hadRo) / this.decAngle) + 0.2;
this.wheelSp.node.rotation = curRo + this.curSpeed;
if ((this.decAngle - hadRo) <= 0) {
// cc.log('....停止');
this.wheelState = 0;
this.wheelSp.node.rotation = this.finalAngle;
if (this.springback) {
//倒转一个齿轮
// var act = new cc.rotateBy(0.6, -this.gearAngle);
var act = cc.rotateBy(0.6, -this.gearAngle);
var seq = cc.sequence(cc.delayTime(0.2), act, cc.callFunc(this.showRes, this));
this.wheelSp.node.runAction(seq);
}
else {
this.showRes();
}
}
}
},
showRes: function () {
// var Config = require("Config");
// if (cc.sys.isBrowser) {
// alert(data, 'You have got ' + this.targetID);
// }
//向后台发数据,刷新页面才能重新生成随机数,所以要把按钮禁用
var data = 0;
if (this.targetID == 1) {
var data = 0;
} else if (this.targetID == 2) {
var data = 1;
} else if (this.targetID == 3) {
var data = 2;
} else if (this.targetID == 4) {
var data = 3;
} else if (this.targetID == 5) {
var data = 5;
} else if (this.targetID == 6) {
var data = 10;
}
this.sendWheelData(data);
},
sendWheelData: function (data) {
var onEnter = function (ret) {
if (ret.errcode !== 0) {
cc.vv.wc.hide();
var msg = "获得奖励失败!";
if (ret.errcode == 1) {
msg = "获得奖励失败,小主可以联系群主需求帮助喔!";
}
else if (ret.errcode == -1) {
msg = "不好意思小主的房卡不足以扣除抽奖所需!";
}
cc.vv.shownotice.show(msg);
}
else {
if (data.gems == 0) {
var msg = "谢谢参与,小主需要继续加油!";
cc.vv.shownotice.show(msg);
} else {
var msg = "恭喜小主获得" + data.gems + "房卡奖励,继续加油!";
cc.vv.shownotice.show(msg);
}
}
};
var data = {
userid: cc.vv.userMgr.userId,
gems: data,
};
cc.vv.http.sendRequest("/send_wheel_data", data, onEnter);
}
});