Matplotlib 练习题
Exercise 11.1: Plotting a function
Plot the function
over the interval [0, 2]. Add proper axis labels, a title, etc.
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
sns.set_style("darkgrid")
x = np.linspace(0, 2, 100)
y = np.power(np.sin(x - 2), 2) * np.exp(-1 * np.power(x, 2))
plt.xlim((0, 2))
plt.ylim((0, 1))
plt.xlabel('x label')
plt.ylabel('y label')
plt.title('$f(x)=sin^2(x-2)e^{-x^2}$')
plt.plot(x, y)
plt.savefig("Figure_1.png")
plt.show()
Output:
Exercise 11.2: Data
Create a data matrix
with 20 observations of 10 variables. Generate a vector
with parameters Then generate the response vector
where
is a vector with standard normally distributed variables.
Now (by only using
and
), find an estimator for
, by solving
Plot the true parameters and estimated parameters . See Figure 1 for an example plot.
import numpy as np
import matplotlib.pyplot as plt
from scipy.optimize import leastsq
def error(b, X, y):
'''返回误差'''
result = np.ravel(X @ b - y)
return result
x = np.linspace(0, 9, 10)
X = np.random.random((20, 10))
b = np.random.random((10, 1))
z = np.random.normal(loc=0, scale=1, size=(20,1))
y = X @ b + z
b_ = np.random.random((10, 1))
b_ = leastsq(error, b_, args=(X,y))[0]
plt.plot(x, b, '.', label='True coefficients')
plt.plot(x, b_, 'x', label='Estimated coefficients')
plt.xlim((0, 9))
plt.ylim((-1.5, 1.5))
plt.xlabel('index')
plt.ylabel('value')
plt.legend()
plt.savefig("Figure_2.png")
plt.show()
Output:
Exercise 11.3: Histogram and density estimation
Generate a vector
of 10000 observations from your favorite exotic distribution. Then make a plot that shows a histogram of
(with 25 bins), along with an estimate for the density, using a Gaussian kernel density estimator (see scipy.stats). See Figure 2 for an example plot.
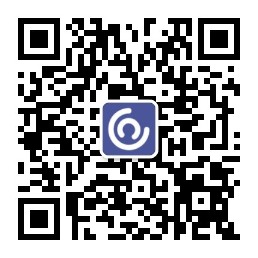
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import gaussian_kde
import seaborn as sns
sns.set_style("darkgrid")
z = np.random.random(size=10000)
kde = gaussian_kde(z)
x = np.linspace(0, 0.9999, 10000)
plt.xlim((0, 1))
plt.hist(z, bins=25, normed=True, color='b')
plt.plot(x, kde(x), 'r-')
plt.savefig('Figure_3.png')
plt.show()
Output: