贪心算法是解决最优化问题的一种方法,解决过程中总是做出当下(局部)最好的选择,因为具有最优子结构的特点,局部最优解可以得到全局最优解;
最优子结构
最优子结构反映了分解问题的方式,我们在解决问题的时候,都会尝试将问题分解成子问题,进而会产生局部解(总体解的子结构)。通常局部解不止一个,当局部解是局部最优解,并且该局部最优解是全局最优解的一部分时,我们称子问题的最优解为 最优子结构。
求解步骤
- 将问题分解为一系列子问题,同时定义子问题的最优解结构;
- 应用贪心原则确定每个子问题的局部最优解,用子问题的局部最优解堆叠出全局最优解。
Assign Cookies
Example 1:
Input: [1,2,3], [1,1]
Output: 1
Explanation: You have 3 children and 2 cookies. The greed factors of 3 children are 1, 2, 3.
And even though you have 2 cookies, since their size is both 1, you could only make the child whose greed factor is 1 content.
You need to output 1.
首先对两个数组进行排序,我们先拿最小的cookie给胃口最小的小朋友。如果能满足当前小朋友,再拿下一个cookie去满足下一位小朋友;如果不能满足,那么我们就用下一块稍大一点的cookie去尝试满足当前的小朋友。
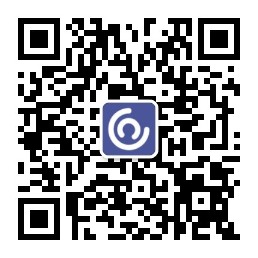
class Solution(object):
def findContentChildren(self, g, s):
"""
:type g: List[int]
:type s: List[int]
:rtype: int
"""
g.sort()
s.sort()
i = 0
for j in range(len(s)):
if i == len(g):
break
if s[j] >= g[i]:
i += 1
return i
Remove K Digits
Given a non-negative integer num represented as a string, remove k digits from the number so that the new number is the smallest possible.
Input: num = "1432219", k = 3
Output: "1219"
遍历给定数字num的每一位,对于当前遍历到的数字d,进行如下while循环:如果还没删够,result不为空,且result的最后一位大于d,那么我们将result的最后一位删掉,k自减1。
class Solution(object):
def removeKdigits(self, num, k):
"""
:type num: str
:type k: int
:rtype: str
"""
result = []
for d in num:
while k and result and result[-1] > d:
result.pop()
k -= 1
result.append(d)
# 删掉剩余的k位,如果删光则返回0
return ''.join(result).lstrip('0')[:-k or None] or '0'
Gas Station
There are N gas stations along a circular route, where the amount of gas at station i is gas[i].
You have a car with an unlimited gas tank and it costs cost[i] of gas to travel from station i to its next station (i+1). You begin the journey with an empty tank at one of the gas stations.
Return the starting gas station's index if you can travel around the circuit once, otherwise return -1.
从0开始出发,如果当前的gas值大于cost值,则前进到下一个站点,剩余的gas加上当前的gas再减去cost,看是否大于0,若大于0,则继续前进。若这个值小于0,则说明从起点到这个点中间的任何一个点都不能作为起点,则把起点设为下一个点,继续遍历。当遍历完整个环时,如果总油量大于总消耗则当前保存的起点即为所求。
class Solution(object):
def canCompleteCircuit(self, gas, cost):
"""
:type gas: List[int]
:type cost: List[int]
:rtype: int
"""
start, cur_sum, total_sum = 0, 0 ,0
for i in range(len(gas)):
diff = gas[i] - cost[i]
cur_sum += diff
total_sum += diff
if cur_sum < 0:
start = i + 1
cur_sum = 0
if total_sum >= 0:
return start
else:
return -1
Candy
There are N children standing in a line. Each child is assigned a rating value.
Each child must have at least one candy.
Children with a higher rating get more candies than their neighbors.
What is the minimum candies you must give?
ratings是由一系列递增,递减或等高子序列组成。等高子序列即平级,每人分一个即可;递增/递减子序列从局部最低点分隔成子域,那么每个子域中的最高点的发放个数应该从序列较长一侧开始计算。
class Solution(object):
def candy(self, ratings):
"""
:type ratings: List[int]
:rtype: int
"""
def count_candy(s1, s2):
# inc,dec中较大的从1开始计算,自然可以保证较小的符合条件
if s1 < s2:
s1, s2 = s2, s1
count = 0
count += ((1 + (s1 + 1)) * (s1 + 1)) / 2
count += ((1 + s2) * s2) / 2
return count
if len(ratings) < 2:
return len(ratings)
inc, dec, count, extra = 0, 0, 0, 0
for i in range(len(ratings) - 1):
if ratings[i] <= ratings[i + 1]:
if dec != 0 or ratings[i] == ratings[i + 1]:
count += count_candy(inc, dec)
inc, dec = 0, 0
if ratings[i] != ratings[i+1]:
inc = 1
# 转折点重复计算了一次
extra += 1
else:
inc += 1
else:
dec += 1
count += count_candy(inc, dec) - extra
return count