Problem Description
度度熊有一张纸条和一把剪刀。
纸条上依次写着 N 个数字,数字只可能是 0 或者 1。
度度熊想在纸条上剪 K 刀(每一刀只能剪在数字和数字之间),这样就形成了 K+1 段。
他再把这 K+1 段按一定的顺序重新拼起来。
不同的剪和接的方案,可能会得到不同的结果。
度度熊好奇的是,前缀 1 的数量最多能是多少。
Input
有多组数据,读到EOF结束。
对于每一组数据,第一行读入两个数 N 和 K 。
第二行有一个长度为 N 的字符串,依次表示初始时纸条上的 N 个数。
0≤K<N≤10000
所有数据 N 的总和不超过100000
Output
对于每一组数据,输出一个数,表示可能的最大前缀 1 的数量。
扫描二维码关注公众号,回复:
2818839 查看本文章
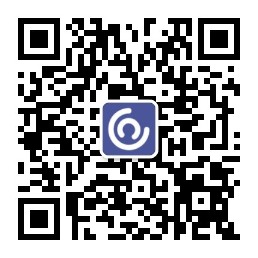
Sample Input
5 1 11010 5 2 11010
Sample Output
2 3
Source
思路:
思路就是记录扫一遍,记录每个含有1的小段(需要记录1的数量,和需要砍的刀数,起点坐标,终点坐标),然后依次枚举谁做第一段(只用1刀)+特判就行。
代码:
#include <iostream>
#include <stdio.h>
#include <string.h>
#include <algorithm>
#include <queue>
#include <map>
#include <vector>
#include <set>
#include <string>
#include <math.h>
#include <stack>
typedef long long ll;
#define INF 0x3f3f3f3f
const int maxn=1e5+10;
const int MAXN=1e3+10;
using namespace std;
int n,k;
char s[maxn];
struct Point
{
int l,r;
int num;
int cut;
}p[maxn];
bool cmp(Point a,Point b)
{
if(a.num==b.num)
{
if(a.cut==b.cut)
{
return a.l<b.l;
}
return a.cut<b.cut;
}
return a.num>b.num;
}
int main(int argc, char const *argv[])
{
#ifndef ONLINE_JUDGE
freopen("in.txt","r",stdin);
freopen("out.txt","w",stdout);
#endif
while(scanf("%d%d",&n,&k)!=EOF)
{
if(n==0)
{
printf("0\n");
continue;
}
scanf("%s",s);
int n=strlen(s);
int cnt=0;
int flag=0;
for(int i=0;i<n;i++)
{
if(s[i]=='1')
{
p[cnt].l=i;
while(s[i]=='1')
{
i++;
}
p[cnt].r=i-1;
p[cnt].num=i-p[cnt].l;
if(p[cnt].l==0)
{
p[cnt].cut=0;
}
else if(p[cnt].r==n-1&&p[cnt].num<n)
{
p[cnt].cut=1;
}
else if(p[cnt].r==n-1&&p[cnt].l==0)
{
p[cnt].cut=0;
}
else
{
p[cnt].cut=2;
}
cnt++;
}
}
sort(p,p+cnt,cmp);
if(k==0)
{
int ans3=0;
for(int i=0;i<cnt;i++)
{
if(p[i].l==0)
{
ans3=p[i].num;
break;
}
}
printf("%d\n",ans3);
continue;
}
int ans=0;
int g=0;
for(int i=0;i<cnt;i++)
{
if(k>=g+p[i].cut)
{
g+=p[i].cut;
ans+=p[i].num;
}
}
int ans2=0,g2=0;
int ans3=0;
if(p[0].l!=0)
{
for(int i=0;i<cnt;i++)
{
ans2=0,g2=0;
int tmp=k;
if(p[i].r==n-1)
{
continue;
}
ans2+=p[i].num;
if(p[i].l!=0) flag=1;
tmp-=1;
for(int j=0;j<cnt;j++)
{
if(j==i) continue;
if(flag==1)
{
if(p[j].l==0)
{
p[j].cut=1;
}
}
if(tmp>=g2+p[j].cut)
{
g2+=p[j].cut;
ans2+=p[j].num;
}
}
ans3=max(ans3,ans2);
}
}
printf("%d\n",max(ans,ans3));
}
return 0;
}