This time, you are supposed to find A+B where A and B are two polynomials.
Input
Each input file contains one test case. Each case occupies 2 lines, and each line contains the information of a polynomial: K N1 aN1 N2 aN2 ... NK aNK, where K is the number of nonzero terms in the polynomial, Ni and aNi (i=1, 2, ..., K) are the exponents and coefficients, respectively. It is given that 1 <= K <= 10,0 <= NK < ... < N2 < N1 <=1000.
Output
For each test case you should output the sum of A and B in one line, with the same format as the input. Notice that there must be NO extra space at the end of each line. Please be accurate to 1 decimal place.
Sample Input
2 1 2.4 0 3.2
2 2 1.5 1 0.5
Sample Output
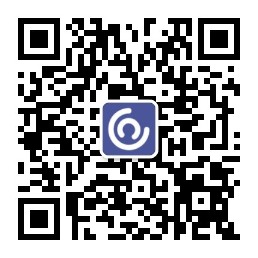
3 2 1.5 1 2.9 0 3.2
题目大意:给出两个多项式求二者的和
思路:用一个结构体数组来保存多项式的系数和指数,其中指数充当结构体数组的下标(由题意知,多项式每一项的指数不超过1000),即若指数为i,则{指数、系数}保存在下标为i的地方,初始所有指数成员均置为-1,(与多项式中真正包含的项数区分开),输入第二个多项式时之间将指数相同的项相加,其中注意若相加为0,则应将该项的指数置为-1(即最终结果暂时删除这一项),最后按指数从大到小方式输出结果多项式(只输出指数不为-1的)
code:
#include<iostream>
#include<stdio.h>
using namespace std;
struct Polynomials{
int exponents;//指数
double coefficients;//底数
};
struct Polynomials p1[1005];//保存第一个多项式,并与第二个多项式相加后保存结果
void Initial(){//对结构体数组进行初始化,将指数均标记为-1有助于之后的加法计算
int i;
for(i=0;i<1005;i++){
p1[i].exponents=-1;
p1[i].coefficients=0.0;
}
}
int main(){
int i,j,p1num,p2num,e;
double c;
int count=0;
Initial();
//读入两组多项式
cin>>p1num;
for(i=0;i<p1num;i++){
cin>>e>>c;
if(p1[e].exponents==-1){
count++;//统计个数
p1[e].exponents=e;
p1[e].coefficients=c;
}
else{
p1[e].coefficients+=c;
if(p1[e].coefficients==0){
count--;
p1[e].exponents=-1;
}
}
}
cin>>p2num;
for(i=0;i<p2num;i++){
cin>>e>>c;
if(p1[e].exponents==-1){
count++;//统计个数
p1[e].exponents=e;
p1[e].coefficients=c;
}
else{
p1[e].coefficients+=c;
if(p1[e].coefficients==0){
count--;
p1[e].exponents=-1;
}
}
}
cout<<count;
for(i=1004;i>=0;i--){
if(p1[i].exponents>=0){
printf(" %d %.1f",p1[i].exponents,p1[i].coefficients);
}
}
return 0;
}