If we represent a date in the format YYYY-MM-DD (for example, 2017-04-09), do you know how many 9s will appear in all the dates between Y1-M1-D1 and Y2-M2-D2 (both inclusive)?
Note that you should take leap years into consideration. A leap year is a year which can be divided by 400 or can be divided by 4 but can't be divided by 100.
Input
The first line of the input is an integer T (1 ≤ T ≤ 105), indicating the number of test cases. Then T test cases follow. For each test case:
The first and only line contains six integers Y1, M1, D1, Y2, M2, D2, their meanings are described above.
It's guaranteed that Y1-M1-D1 is not larger than Y2-M2-D2. Both Y1-M1-D1 and Y2-M2-D2 are between 2000-01-01 and 9999-12-31, and both dates are valid.
We kindly remind you that this problem contains large I/O file, so it's recommended to use a faster I/O method. For example, you can use scanf/printf instead of cin/cout in C++.
Output
For each test case, you should output one line containing one integer, indicating the answer of this test case.
Sample Input
4 2017 04 09 2017 05 09 2100 02 01 2100 03 01 9996 02 01 9996 03 01 2000 01 01 9999 12 31
Sample Output
4 2 93 1763534
Hint
For the first test case, four 9s appear in all the dates between 2017-04-09 and 2017-05-09. They are: 2017-04-09 (one 9), 2017-04-19 (one 9), 2017-04-29 (one 9), and 2017-05-09 (one 9).
For the second test case, as year 2100 is not a leap year, only two 9s appear in all the dates between 2100-02-01 and 2100-03-01. They are: 2017-02-09 (one 9) and 2017-02-19 (one 9).
For the third test case, at least three 9s appear in each date between 9996-02-01 and 9996-03-01. Also, there are three additional nines, namely 9996-02-09 (one 9), 9996-02-19 (one 9) and 9996-02-29 (one 9). So the answer is 3 × 30 + 3 = 93.
思路是分年份相同与否两种情况,前者直接暴力计算,后者取时间段两头的时间和中间的时间分开计算。比赛的时候卡了两次。第一次是因为计算年份不同时中间年份里的9而tle,选择采取了预处理。第二次是tle之后的wa……是因为自己失误少算了月份里的9。还是一道比较复杂的水题,因为wa得很多也懒得把代码改美观了,又臭又长。
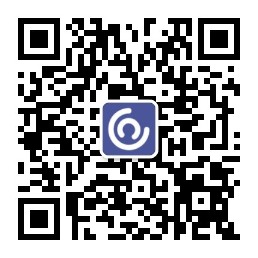
#include <iostream> #include <string> #include <cstdio> #include <map> #include <vector> #include <set> #include <algorithm> using namespace std; int day[2][12] = {{3,2,3,3,3,3,3,3,33,3,3,3}, {3,3,3,3,3,3,3,3,33,3,3,3}}; int sumday[2][12] = {{31,28,31,30,31,30,31,31,30,31,30,31}, {31,29,31,30,31,30,31,31,30,31,30,31}}; int aaa[10011], summ=0; bool check(int x) { if (x % 400 == 0 || (x % 100 != 0 && x % 4 == 0)) return true; else return false; } int nine(int a) { int num[4], ans = 0; num[0] = a / 1000, num[1] = (a / 100) % 10, num[2] = (a / 10) % 10, num[3] = a % 10; if (num[0] == 9) ans++; if (num[1] == 9) ans++; if (num[2] == 9) ans++; if (num[3] == 9) ans++; return ans; } void init(){ int i; for (i = 2000; i <= 10011; i++) { if (check(i)) { summ += 66; summ += nine(i) * 366; } else { summ += 65; summ += nine(i) * 365; } aaa[i]=summ; } return; } int main() { int t; init(); scanf("%d", &t); while (t--) { int y, yy, m, mm, d, dd; scanf("%d%d%d%d%d%d", &y, &m, &d, &yy, &mm, &dd); int sum = 0; if (y == yy) { if (m == mm) { for (int i = d; i <= dd; i++) { if (i == 9 || i == 19 || i == 29) sum++; sum += nine(y) + nine(m); } } else { for (int i = d; i <= (check(y) ? sumday[1][m - 1] : sumday[0][m - 1]); i++) { if (i == 9 || i == 19 || i == 29) sum++; sum += nine(y) + nine(m); } for (int i = m + 1; i < mm; i++) { if (check(y)) { sum += day[1][i - 1]; sum += nine(y) * sumday[1][i - 1]; } else { sum += day[0][i - 1]; sum += nine(y) * sumday[0][i - 1]; } } for (int i = 1; i <= dd; i++) { if (i == 9 || i == 19 || i == 29) sum++; sum += nine(y) + nine(mm); } } } else { for (int i = d; i <= (check(y) ? sumday[1][m - 1] : sumday[0][m - 1]); i++) { if (i == 9 || i == 19 || i == 29) sum++; sum += nine (y) + nine(m); } for (int i = m + 1; i <= 12; i++) { if (check(y)) { sum += day[1][i - 1]; sum += nine(y) * sumday[1][i - 1]; } else { sum += day[0][i - 1]; sum += nine(y) * sumday[0][i - 1]; } } sum += aaa[yy-1] - aaa[y]; for (int i = 1; i < mm; i++) { if (check(yy)) { sum += day[1][i - 1]; sum += nine(yy) * sumday[1][i - 1]; } else { sum += day[0][i - 1]; sum += nine(yy) * sumday[0][i - 1]; } } for (int i = 1; i <= dd; i++) { if (i == 9 || i == 19 || i == 29) sum++; sum += nine(yy) + nine(mm); } } printf("%d\n", sum); } return 0; }