每个service都几乎需要以下方法:
1、 queryById
2、 queryAll
3、 queryOne
4、 queryListByWhere
5、 queryPageListByWhere
6、 save
7、 update
8、 deleteById
9、 deleteByIds
10、deleteByWhere
在封装BaseService的时候会遇到下面的问题:
所以我们可以通过下面这种方式:
package com.taotao.manage.service;
import java.util.Date;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import com.github.abel533.entity.Example;
import com.github.abel533.mapper.Mapper;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.taotao.manage.pojo.BasePojo;
public abstract class BaseService<T extends BasePojo> {
//@Autowired
//private Mapper<T> mapper;
public abstract Mapper<T> getMapper();
/**
* 根据id查询数据
*
* @param id
* @return
*/
public T queryById(Long id) {
return this.getMapper().selectByPrimaryKey(id);
}
/**
* 查询所有数据
*
* @return
*/
public List<T> queryAll() {
return this.getMapper().select(null);
}
/**
* 根据条件查询一条数据,如果有多条数据会抛出异常
*
* @param record
* @return
*/
public T queryOne(T record) {
return this.getMapper().selectOne(record);
}
/**
* 根据条件查询数据列表
*
* @param record
* @return
*/
public List<T> queryListByWhere(T record) {
return this.getMapper().select(record);
}
/**
* 分页查询
*
* @param page
* @param rows
* @param record
* @return
*/
public PageInfo<T> queryPageListByWhere(Integer page, Integer rows, T record) {
// 设置分页条件
PageHelper.startPage(page, rows);
List<T> list = this.queryListByWhere(record);
return new PageInfo<T>(list);
}
/**
* 新增数据,返回成功的条数
*
* @param record
* @return
*/
public Integer save(T record) {
record.setCreated(new Date());
record.setUpdated(record.getCreated());
return this.getMapper().insert(record);
}
/**
* 新增数据,使用不为null的字段,返回成功的条数
*
* @param record
* @return
*/
public Integer saveSelective(T record) {
record.setCreated(new Date());
record.setUpdated(record.getCreated());
return this.getMapper().insertSelective(record);
}
/**
* 修改数据,返回成功的条数
*
* @param record
* @return
*/
public Integer update(T record) {
return this.getMapper().updateByPrimaryKey(record);
}
/**
* 修改数据,使用不为null的字段,返回成功的条数
*
* @param record
* @return
*/
public Integer updateSelective(T record) {
record.setUpdated(new Date());
return this.getMapper().updateByPrimaryKeySelective(record);
}
/**
* 根据id删除数据
*
* @param id
* @return
*/
public Integer deleteById(Long id) {
return this.getMapper().deleteByPrimaryKey(id);
}
/**
* 批量删除
* @param clazz
* @param property
* @param values
* @return
*/
public Integer deleteByIds(Class<T> clazz, String property, List<Object> values) {
Example example = new Example(clazz);
example.createCriteria().andIn(property, values);
return this.getMapper().deleteByExample(example);
}
/**
* 根据条件做删除
*
* @param record
* @return
*/
public Integer deleteByWhere(T record) {
return this.getMapper().delete(record);
}
}
使用BaseService改造ItemCatService
package com.taotao.manage.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.github.abel533.mapper.Mapper;
import com.taotao.manage.mapper.ItemCatMapper;
import com.taotao.manage.pojo.ItemCat;
@Service
public class ItemCatService extends BaseService<ItemCat>{
@Autowired
private ItemCatMapper itemCatMapper;
// public List<ItemCat> queryItemCat(Long parentId) {
// ItemCat record = new ItemCat();
// record.setParentId(parentId);
// return this.itemCatMapper.select(record );
// }
@Override
public Mapper<ItemCat> getMapper() {
// TODO Auto-generated method stub
return this.itemCatMapper;
}
}
在Controller:
测试
Spring4的泛型注入
BaseService的优化
测试:
扫描二维码关注公众号,回复:
167733 查看本文章
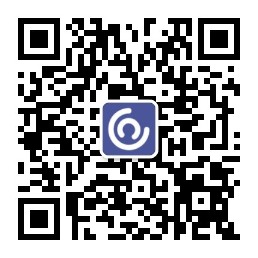
测试:运行时注入具体的通用Mapper的子接口的实现类:
问题
ItemCatMapper在编码时没有使用到,是否将其删除? – 不能。
原因:在Spring运行时会使用该对象,将其注入到BaseService中。