商品数据结构
表结构:
为什么价格要以最小单位分存储?
– 为了避免小数计算带来的问题。
商品描述表:
为什么要将商品描述和商品的基本数据分离?
1、 商品描述的数据量大,会导致数据文件变大,影响查询速度。
2、 后期会对商品描述数据的存储做改造,所以需要将描述数据分离
点击商品类目的叶子节点的事件
在item-add.jsp中定义:
新增页面中价格
KindEditor的使用
效果:
1、 导入js文件
2、 定义多行文本
3、 通过JS创建出富文本编辑器
Common.js:
提交事件
function submitForm(){
if(!$('#itemAddForm').form('validate')){
$.messager.alert('提示','表单还未填写完成!');
return ;
}
//处理商品的价格的单位,将元转化为分
$("#itemAddForm [name=price]").val(eval($("#itemAddForm [name=priceView]").val()) * 100);
//将编辑器中的内容同步到隐藏多行文本中
itemAddEditor.sync();
//输入的规格参数数据保存为json
var paramJson = [];
$("#itemAddForm .params li").each(function(i,e){
var trs = $(e).find("tr");
var group = trs.eq(0).text();
var ps = [];
for(var i = 1;i<trs.length;i++){
var tr = trs.eq(i);
ps.push({
"k" : $.trim(tr.find("td").eq(0).find("span").text()),
"v" : $.trim(tr.find("input").val())
});
}
paramJson.push({
"group" : group,
"params": ps
});
});
paramJson = JSON.stringify(paramJson);
$("#itemAddForm [name=itemParams]").val(paramJson);
/*
$.post("/rest/item/save",$("#itemAddForm").serialize(), function(data){
if(data.status == 200){
$.messager.alert('提示','新增商品成功!');
}
});
*/
//提交到后台的RESTful
$.ajax({
type: "POST",
url: "/rest/item",
data: $("#itemAddForm").serialize(), //表单序列化,将所有的输入内容转化成K/V数据格式
statusCode : {
201 : function(){
$.messager.alert('提示','新增商品成功!');
},
400 : function(){
$.messager.alert('提示','提交的参数不合法!');
},
500 : function(){
$.messager.alert('提示','新增商品失败!');
}
}
});
}
商品描述表中业务id
实现
POJO
package com.taotao.manage.pojo;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
@Table(name = "tb_item")
public class Item extends BasePojo {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
private String sellPoint;
private Long price;
private Integer num;
private String barcode;
private String image;
private Long cid;
private Integer status;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getSellPoint() {
return sellPoint;
}
public void setSellPoint(String sellPoint) {
this.sellPoint = sellPoint;
}
public Long getPrice() {
return price;
}
public void setPrice(Long price) {
this.price = price;
}
public Integer getNum() {
return num;
}
public void setNum(Integer num) {
this.num = num;
}
public String getBarcode() {
return barcode;
}
public void setBarcode(String barcode) {
this.barcode = barcode;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
public Long getCid() {
return cid;
}
public void setCid(Long cid) {
this.cid = cid;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
@Override
public String toString() {
return "Item [id=" + id + ", title=" + title + ", sellPoint=" + sellPoint + ", price=" + price
+ ", num=" + num + ", barcode=" + barcode + ", image=" + image + ", cid=" + cid + ", status="
+ status + "]";
}
}
package com.taotao.manage.pojo;
import javax.persistence.Id;
import javax.persistence.Table;
@Table(name = "tb_item_desc")
public class ItemDesc extends BasePojo{
@Id//对应tb_item中的id
private Long itemId;
private String itemDesc;
public Long getItemId() {
return itemId;
}
public void setItemId(Long itemId) {
this.itemId = itemId;
}
public String getItemDesc() {
return itemDesc;
}
public void setItemDesc(String itemDesc) {
this.itemDesc = itemDesc;
}
}
Mapper
package com.taotao.manage.mapper;
import com.github.abel533.mapper.Mapper;
import com.taotao.manage.pojo.Item;
public interface ItemMapper extends Mapper<Item>{
}
package com.taotao.manage.mapper;
import com.github.abel533.mapper.Mapper;
import com.taotao.manage.pojo.ItemDesc;
public interface ItemDescMapper extends Mapper<ItemDesc> {
}
Controller
扫描二维码关注公众号,回复:
167727 查看本文章
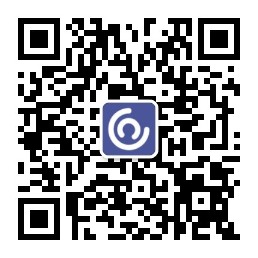
事务问题
解决:
将2次保存放到同一个Service的同一个方法中即可。
package com.taotao.manage.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.taotao.manage.pojo.Item;
import com.taotao.manage.pojo.ItemDesc;
@Service
public class ItemService extends BaseService<Item> {
@Autowired
private ItemDescService itemDescService;
public Boolean saveItem(Item item, String desc) {
// 初始值
item.setStatus(1);
item.setId(null); // 出于安全考虑,强制设置id为null,通过数据库自增长得到
Integer count1 = super.save(item);
// 保存商品描述数据
ItemDesc itemDesc = new ItemDesc();
itemDesc.setItemId(item.getId());
itemDesc.setItemDesc(desc);
Integer count2 = this.itemDescService.save(itemDesc);
return count1.intValue() == 1 && count2.intValue() == 1;
}
}
package com.taotao.manage.service;
import org.springframework.stereotype.Service;
import com.taotao.manage.pojo.ItemDesc;
@Service
public class ItemDescService extends BaseService<ItemDesc>{
}
Controller:
package com.taotao.manage.controller;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import com.taotao.manage.pojo.Item;
import com.taotao.manage.service.ItemService;
@RequestMapping("item")
@Controller
public class ItemController {
@Autowired
private ItemService itemService;
@RequestMapping(method = RequestMethod.POST)
public ResponseEntity<Void> saveItem(Item item, @RequestParam("desc") String desc) {
try {
if (StringUtils.isEmpty(item.getTitle())) { // TODO 未完成,待优化
// 参数有误, 400
return ResponseEntity.status(HttpStatus.BAD_REQUEST).build();
}
// 保存商品
Boolean bool = this.itemService.saveItem(item, desc);
if (!bool) {
// 保存失败
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
return ResponseEntity.status(HttpStatus.CREATED).build();
} catch (Exception e) {
e.printStackTrace();
}
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
测试运行看日志:
从日志可以看出是同属于一个事务。