对外的接口服务
我们把上一篇:【SSM分布式架构电商项目-14】后台CMS内容管理系统管理前台首页广告里面的ContentController拷贝到api包下,对外提供接口服务:
package com.taotao.manage.controller.api;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import com.taotao.common.bean.EasyUIResult;
import com.taotao.manage.service.ContentService;
@RequestMapping("api/content")
@Controller
public class ApiContentController {
@Autowired
private ContentService contentService;
/**
* 根据内容分类id查询分类列表
*
* @param categoryId
* @param page
* @param rows
* @return
*/
@RequestMapping(method = RequestMethod.GET)
public ResponseEntity<EasyUIResult> queryListByCategoryId(@RequestParam("categoryId") Long categoryId,
@RequestParam(value = "page", defaultValue = "1") Integer page,
@RequestParam(value = "rows", defaultValue = "10") Integer rows) {
try {
EasyUIResult easyUIResult = this.contentService.queryListByCategoryId(categoryId, page, rows);
return ResponseEntity.ok(easyUIResult);
} catch (Exception e) {
e.printStackTrace();
}
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(null);
}
}
测试:
访问接口服务的方式
方式有2种:
1、 js访问
a) 有跨域 – jsonp解决
b) 无跨域 – ajax解决
2、 Java代码访问
a) Httpclient
Httpclient
导入依赖
DoGET
package cn.itcast.httpclient;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class DoGET {
public static void main(String[] args) throws Exception {
// 创建Httpclient对象
CloseableHttpClient httpclient = HttpClients.createDefault();
// 创建http GET请求
HttpGet httpGet = new HttpGet("http://www.baidu.com/s?wd=java");
CloseableHttpResponse response = null;
try {
// 执行请求
response = httpclient.execute(httpGet);
// 判断返回状态是否为200
if (response.getStatusLine().getStatusCode() == 200) {
String content = EntityUtils.toString(response.getEntity(), "UTF-8");
System.out.println("内容:"+content);
}
} finally {
if (response != null) {
response.close();
}
httpclient.close();
}
}
}
带有参数的GET请求
package cn.itcast.httpclient;
import java.net.URI;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class DoGETParam {
public static void main(String[] args) throws Exception {
// 创建Httpclient对象
CloseableHttpClient httpclient = HttpClients.createDefault();
// 定义请求的参数
URI uri = new URIBuilder("http://manage.taotao.com/rest/api/content").setParameter("categoryId", "33")
.setParameter("page", "1").setParameter("rows", "1").build();
System.out.println(uri);
// 创建http GET请求
HttpGet httpGet = new HttpGet(uri);
CloseableHttpResponse response = null;
try {
// 执行请求
response = httpclient.execute(httpGet);
// 判断返回状态是否为200
if (response.getStatusLine().getStatusCode() == 200) {
String content = EntityUtils.toString(response.getEntity(), "UTF-8");
System.out.println(content);
}
} finally {
if (response != null) {
response.close();
}
httpclient.close();
}
}
}
DoPOST
package cn.itcast.httpclient;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.NameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
public class DoPOSTParam {
public static void main(String[] args) throws Exception {
// 创建Httpclient对象
CloseableHttpClient httpclient = HttpClients.createDefault();
// 创建http POST请求
HttpPost httpPost = new HttpPost("http://www.oschina.net/search");
// 伪装成浏览器
httpPost.setHeader(
"User-Agent",
"Mozilla/5.0 (Windows NT 6.3; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/50.0.2661.94 Safari/537.36");
// 设置2个post参数,一个是scope、一个是q
List<NameValuePair> parameters = new ArrayList<NameValuePair>(0);
parameters.add(new BasicNameValuePair("scope", "project"));
parameters.add(new BasicNameValuePair("q", "java"));
parameters.add(new BasicNameValuePair("fromerr", "7nXH76r7"));
// 构造一个form表单式的实体
UrlEncodedFormEntity formEntity = new UrlEncodedFormEntity(parameters);
// 将请求实体设置到httpPost对象中
httpPost.setEntity(formEntity);
CloseableHttpResponse response = null;
try {
// 执行请求
response = httpclient.execute(httpPost);
// 判断返回状态是否为200
if (response.getStatusLine().getStatusCode() == 200) {
String content = EntityUtils.toString(response.getEntity(), "UTF-8");
System.out.println(content);
}
} finally {
if (response != null) {
response.close();
}
httpclient.close();
}
}
}
带有参数的POST请求
public static void main(String[] args) throws Exception {
// 创建Httpclient对象
CloseableHttpClient httpclient = HttpClients.createDefault();
// 创建http POST请求
HttpPost httpPost = new HttpPost("http://www.oschina.net/search");
// 伪装成浏览器
httpPost.setHeader("User-Agent", "Mozilla/5.0 (Windows NT 6.3; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/50.0.2661.94 Safari/537.36");
// 设置2个post参数,一个是scope、一个是q
List<NameValuePair> parameters = new ArrayList<NameValuePair>(0);
parameters.add(new BasicNameValuePair("scope", "project"));
parameters.add(new BasicNameValuePair("q", "java"));
parameters.add(new BasicNameValuePair("fromerr", "7nXH76r7"));
// 构造一个form表单式的实体
UrlEncodedFormEntity formEntity = new UrlEncodedFormEntity(parameters);
// 将请求实体设置到httpPost对象中
httpPost.setEntity(formEntity);
CloseableHttpResponse response = null;
try {
// 执行请求
response = httpclient.execute(httpPost);
// 判断返回状态是否为200
if (response.getStatusLine().getStatusCode() == 200) {
String content = EntityUtils.toString(response.getEntity(), "UTF-8");
System.out.println(content);
}
} finally {
if (response != null) {
response.close();
}
httpclient.close();
}
}
连接管理器
注意:
package cn.itcast.httpclient;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.conn.HttpClientConnectionManager;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.impl.conn.PoolingHttpClientConnectionManager;
import org.apache.http.util.EntityUtils;
public class HttpConnectManager {
public static void main(String[] args) throws Exception {
PoolingHttpClientConnectionManager cm = new PoolingHttpClientConnectionManager();
// 设置最大连接数
cm.setMaxTotal(200);
// 设置每个主机地址的并发数
cm.setDefaultMaxPerRoute(20);
doGet(cm);
doGet(cm);
}
public static void doGet(HttpClientConnectionManager cm) throws Exception {
CloseableHttpClient httpClient = HttpClients.custom().setConnectionManager(cm).build();
// 创建http GET请求
HttpGet httpGet = new HttpGet("http://www.baidu.com/");
CloseableHttpResponse response = null;
try {
// 执行请求
response = httpClient.execute(httpGet);
// 判断返回状态是否为200
if (response.getStatusLine().getStatusCode() == 200) {
String content = EntityUtils.toString(response.getEntity(), "UTF-8");
System.out.println("内容长度:" + content.length());
}
} finally {
if (response != null) {
response.close();
}
// 此处不能关闭httpClient,如果关闭httpClient,连接池也会销毁
// httpClient.close();
}
}
}
定期关闭无效连接
public class ClientEvictExpiredConnections {
public static void main(String[] args) throws Exception {
PoolingHttpClientConnectionManager cm = new PoolingHttpClientConnectionManager();
// 设置最大连接数
cm.setMaxTotal(200);
// 设置每个主机地址的并发数
cm.setDefaultMaxPerRoute(20);
new IdleConnectionEvictor(cm).start();
}
public static class IdleConnectionEvictor extends Thread {
private final HttpClientConnectionManager connMgr;
private volatile boolean shutdown;
public IdleConnectionEvictor(HttpClientConnectionManager connMgr) {
this.connMgr = connMgr;
}
@Override
public void run() {
try {
while (!shutdown) {
synchronized (this) {
wait(5000);
// 关闭失效的连接
connMgr.closeExpiredConnections();
}
}
} catch (InterruptedException ex) {
// 结束
}
}
public void shutdown() {
shutdown = true;
synchronized (this) {
notifyAll();
}
}
}
}
设置请求参数
Httpclient和Spring的整合
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context" xmlns:p="http://www.springframework.org/schema/p"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.0.xsd">
<bean id="connectionManager"
class="org.apache.http.impl.conn.PoolingHttpClientConnectionManager">
<!-- 最大连接数 -->
<property name="maxTotal" value="${http.maxTotal}" />
<!-- 设置每个主机地址的并发数 -->
<property name="defaultMaxPerRoute" value="${http.defaultMaxPerRoute}" />
</bean>
<!-- Httpclient对象的构建器 -->
<bean id="httpClientBuilder" class="org.apache.http.impl.client.HttpClientBuilder">
<property name="connectionManager" ref="connectionManager" />
</bean>
<!-- Httpclient对象 -->
<!-- 注意:该对象为多例 -->
<bean class="org.apache.http.impl.client.CloseableHttpClient"
factory-bean="httpClientBuilder" factory-method="build" scope="prototype">
</bean>
<!-- 请求配置的构建器 -->
<bean id="requestConfigBuilder" class="org.apache.http.client.config.RequestConfig.Builder">
<!-- 创建连接的最长时间 -->
<property name="connectTimeout" value="${http.connectTimeout}" />
<!-- 从连接池中获取到连接的最长时间 -->
<property name="connectionRequestTimeout" value="${http.connectionRequestTimeout}" />
<!-- 数据传输的最长时间 -->
<property name="socketTimeout" value="${http.socketTimeout}" />
<!-- 提交请求前测试连接是否可用 -->
<property name="staleConnectionCheckEnabled" value="${http.staleConnectionCheckEnabled}" />
</bean>
<!-- 请求配置对象 -->
<bean class="org.apache.http.client.config.RequestConfig"
factory-bean="requestConfigBuilder" factory-method="build" />
<!-- 定期清理无效连接 -->
<bean class="com.taotao.common.httpclient.IdleConnectionEvictor">
<constructor-arg index="0" ref="connectionManager"/>
</bean>
</beans>
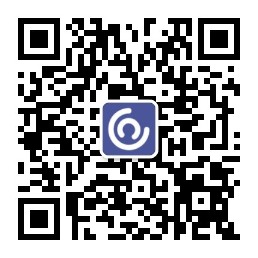
分析:
我们先配置连接管理器:PoolingHttpClientConnectionManager
之后我们需要拿到CloseableHttpClient:
CloseableHttpClient httpClient = HttpClients.custom().setConnectionManager(cm).build();
这里分两步,先是HttpClients.custom()
,然后并设置setConnectionManager(cm)。
第二步使用build()方法生成CloseableHttpClient 对象。
第一步:
我们可以看到在第一步HttpClients.custom().setConnectionManager(cm)
最后返回的是HttpClientBuilder对象,所以我们先来配置HttpClientBuilder的bean
第二步:使用build()方法:
接下来我们要配置请求参数:
// 构建请求配置信息
RequestConfig config = RequestConfig.custom().setConnectTimeout(1000) // 创建连接的最长时间
.setConnectionRequestTimeout(500) // 从连接池中获取到连接的最长时间
.setSocketTimeout(10 * 1000) // 数据传输的最长时间
.setStaleConnectionCheckEnabled(true) // 提交请求前测试连接是否可用
.build();
这里我们也分两步:第一步先是RequestConfig.custom()得到org.apache.http.client.config.RequestConfig.Builder,并且设置其属性,第二步通过build()方法得到RequestConfig 对象。
所以我们先配置 org.apache.http.client.config.RequestConfig.Builder
之后我们在给它设置属性:
第二步:通过build方法得到RequestConfig
接下来我们还要配置定期清理无用连接,我们在common中加入:
package com.taotao.common.httpclient;
import org.apache.http.conn.HttpClientConnectionManager;
public class IdleConnectionEvictor extends Thread {
private final HttpClientConnectionManager connMgr;
private volatile boolean shutdown;
public IdleConnectionEvictor(HttpClientConnectionManager connMgr) {
this.connMgr = connMgr;
// 启动当前线程
this.start();
}
@Override
public void run() {
try {
while (!shutdown) {
synchronized (this) {
wait(5000);
// 关闭失效的连接
connMgr.closeExpiredConnections();
}
}
} catch (InterruptedException ex) {
// 结束
}
}
public void shutdown() {
shutdown = true;
synchronized (this) {
notifyAll();
}
}
}
然后我们在配置文件中配置:
然后我们要加入外部配置文件:
在Spring配置中,加载外部配置文件:
封装ApiService
我们来封装使用HttpClient的通用Service,我们把它放在common中。
package com.taotao.common.service;
import java.io.IOException;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.BeanFactory;
import org.springframework.beans.factory.BeanFactoryAware;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.taotao.common.httpclient.HttpResult;
@Service
public class ApiService implements BeanFactoryAware {
@Autowired(required = false)
private RequestConfig requestConfig;
private BeanFactory beanFactory;
/**
* GET请求地址,响应200,返回响应的内容,响应为404、500返回null
*
* @param url
* @return
* @throws ClientProtocolException
* @throws IOException
*/
public String doGet(String url) throws ClientProtocolException, IOException {
// 创建http GET请求
HttpGet httpGet = new HttpGet(url);
httpGet.setConfig(this.requestConfig);
CloseableHttpResponse response = null;
try {
// 执行请求
response = getHttpclient().execute(httpGet);
// 判断返回状态是否为200
if (response.getStatusLine().getStatusCode() == 200) {
return EntityUtils.toString(response.getEntity(), "UTF-8");
}
} finally {
if (response != null) {
response.close();
}
}
return null;
}
/**
* 带有参数的GET请求
*
* @param url
* @param params
* @return
* @throws ClientProtocolException
* @throws IOException
* @throws URISyntaxException
*/
public String doGet(String url, Map<String, String> params) throws ClientProtocolException, IOException,
URISyntaxException {
// 定义请求的参数
URIBuilder builder = new URIBuilder(url);
for (Map.Entry<String, String> entry : params.entrySet()) {
builder.setParameter(entry.getKey(), entry.getValue());
}
return this.doGet(builder.build().toString());
}
/**
* 带有参数的POST请求
*
* @param url
* @param params
* @return
* @throws ClientProtocolException
* @throws IOException
*/
public HttpResult doPost(String url, Map<String, String> params) throws ClientProtocolException,
IOException {
// 创建http POST请求
HttpPost httpPost = new HttpPost(url);
httpPost.setConfig(this.requestConfig);
if (null != params) {
List<NameValuePair> parameters = new ArrayList<NameValuePair>(0);
for (Map.Entry<String, String> entry : params.entrySet()) {
parameters.add(new BasicNameValuePair(entry.getKey(), entry.getValue()));
}
// 构造一个form表单式的实体
UrlEncodedFormEntity formEntity = new UrlEncodedFormEntity(parameters);
// 将请求实体设置到httpPost对象中
httpPost.setEntity(formEntity);
}
CloseableHttpResponse response = null;
try {
// 执行请求
response = getHttpclient().execute(httpPost);
HttpEntity entity = response.getEntity();
if (null == entity) {
return new HttpResult(response.getStatusLine().getStatusCode(), null);
}
return new HttpResult(response.getStatusLine().getStatusCode(), EntityUtils.toString(
response.getEntity(), "UTF-8"));
} finally {
if (response != null) {
response.close();
}
}
}
/**
* 带有json参数的POST请求
*
* @param url
* @param params
* @return
* @throws ClientProtocolException
* @throws IOException
*/
public HttpResult doPostJson(String url, String json) throws ClientProtocolException,
IOException {
// 创建http POST请求
HttpPost httpPost = new HttpPost(url);
httpPost.setConfig(this.requestConfig);
if (null != json) {
StringEntity stringEntity = new StringEntity(json, ContentType.APPLICATION_JSON);
// 将请求实体设置到httpPost对象中
httpPost.setEntity(stringEntity);
}
CloseableHttpResponse response = null;
try {
// 执行请求
response = getHttpclient().execute(httpPost);
HttpEntity entity = response.getEntity();
if (null == entity) {
return new HttpResult(response.getStatusLine().getStatusCode(), null);
}
return new HttpResult(response.getStatusLine().getStatusCode(), EntityUtils.toString(
response.getEntity(), "UTF-8"));
} finally {
if (response != null) {
response.close();
}
}
}
/**
* 没有参数的POST请求
*
* @param url
* @return
* @throws ClientProtocolException
* @throws IOException
*/
public HttpResult doPost(String url) throws ClientProtocolException, IOException {
return this.doPost(url, null);
}
private CloseableHttpClient getHttpclient() {
return this.beanFactory.getBean(CloseableHttpClient.class);
}
@Override
public void setBeanFactory(BeanFactory beanFactory) throws BeansException {
// 该方法是在Spring容器初始化时会调用该方法,传入beanFactory
this.beanFactory = beanFactory;
}
}
异常处理:
get返回值是根据状态码为200则返回数据,状态码不为200则返回null。post的返回值必须返回响应状态码和内容,比如返回状态码为201的时候同时也会返回内容,所以我们构造一个对象来返回:
package com.taotao.common.httpclient;
public class HttpResult {
private Integer code;
private String body;
public HttpResult() {
}
public HttpResult(Integer code, String body) {
this.code = code;
this.body = body;
}
public Integer getCode() {
return code;
}
public void setCode(Integer code) {
this.code = code;
}
public String getBody() {
return body;
}
public void setBody(String body) {
this.body = body;
}
}
然后我们再来看一个单例对象中如何使用多例的对象的问题:
原本我们应该这样写:
然后我们通过注入的httpclient使用httpclient.execute(httpGet);
得到CloseableHttpResponse对象。但是因为我们注解@Service的ApiService是单例的,只会实例化一次,实例化一次的时候只会注入一次httpclient,那么我们的httpclient就变成单例的了。然而我们的httpclient必须是个多例对象。那我们可能会想把ApiService变成多例的,加@Scope(“prototype”)就可以了。那么如果有其他的Service或者Controller要调用这个ApiService是不是也是同样的情况,也是在单例对象中使用多例对象。所以这个方法不可行。那么我们如何在单例对象中使用多例对象?
解决方法就是:httpclient这个对象不能注入,而是每次使用的时候,通过容器里面去获取,因为在容器里面指定httpclient是多例的。
所以主要的代码截图下:
通过以上步骤就可以达到在单例对象中使用多例对象。