nodejs环境
nodejs是当下前端工程化开发必不可少的环境, 使用 nodejs的 npm功能来管理依赖包
查看node 和 npm的版本
node -v #查看node版本
npm -v #查看npm版本
git版本控制
git版本控制工具是目前最为流行的分布式版本管理工具,代码的**提交, 检出, 日志**, 都需要通过git完成
git --version #查看git安装版本
npm淘宝镜像
npm是非常重要的npm管理工具,由于npm的服务器位于国外, 所以一般建议 将 npm设置成国内的淘宝镜像
npm config set registry https://registry.npm.taobao.org/ #设置淘宝镜像地址
npm config get registry #查看镜像地址
vscode编辑器
vscode编辑器插件 + vetur + eslint
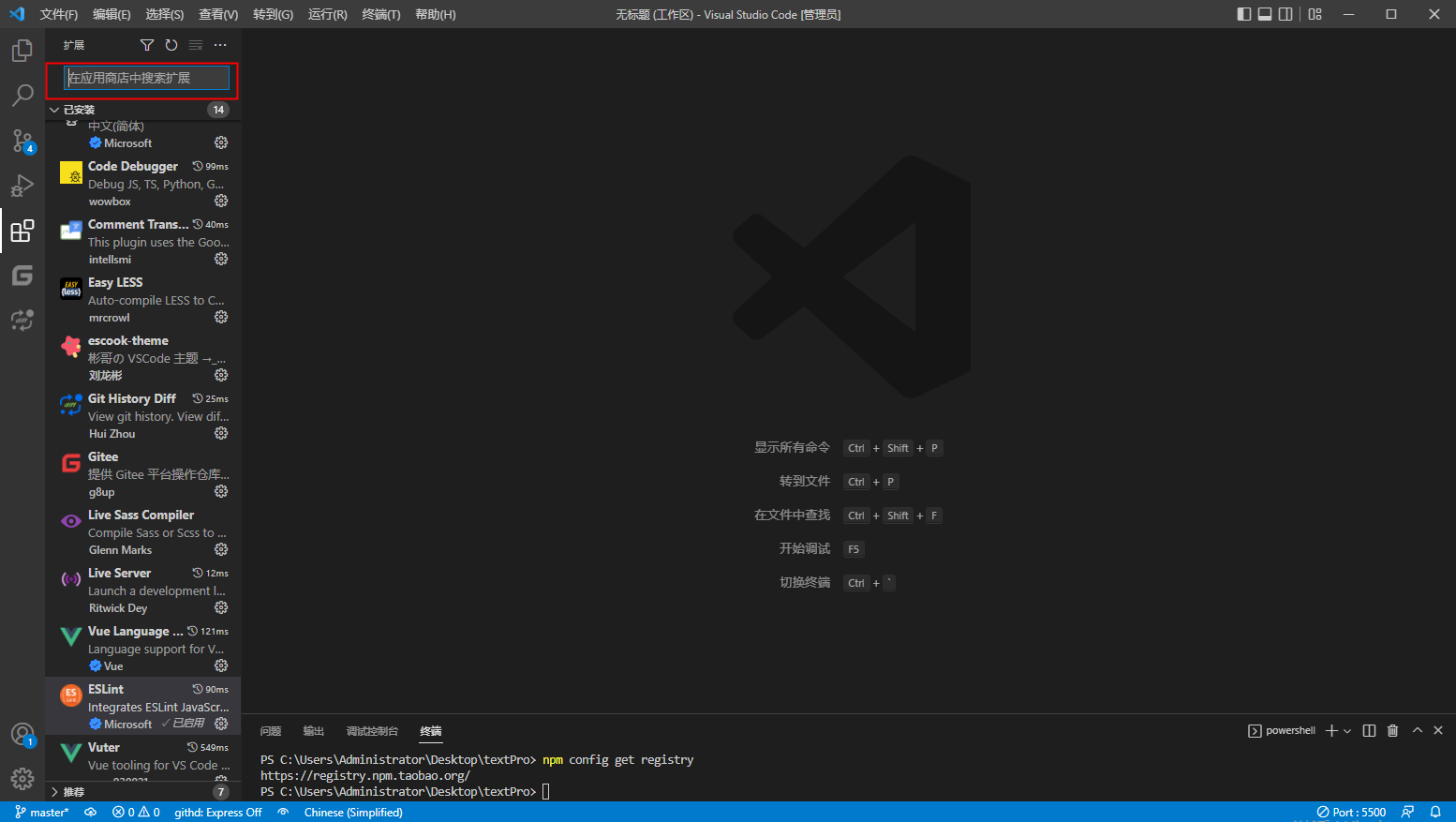
-
除此之外, eslint需要在vscode中进行一些参数的配置
{
"eslint.enable": true,
"eslint.run": "onType",
"eslint.options": {
"extensions": [
".js",
".vue",
".jsx",
".tsx"
]
},
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true
}
}
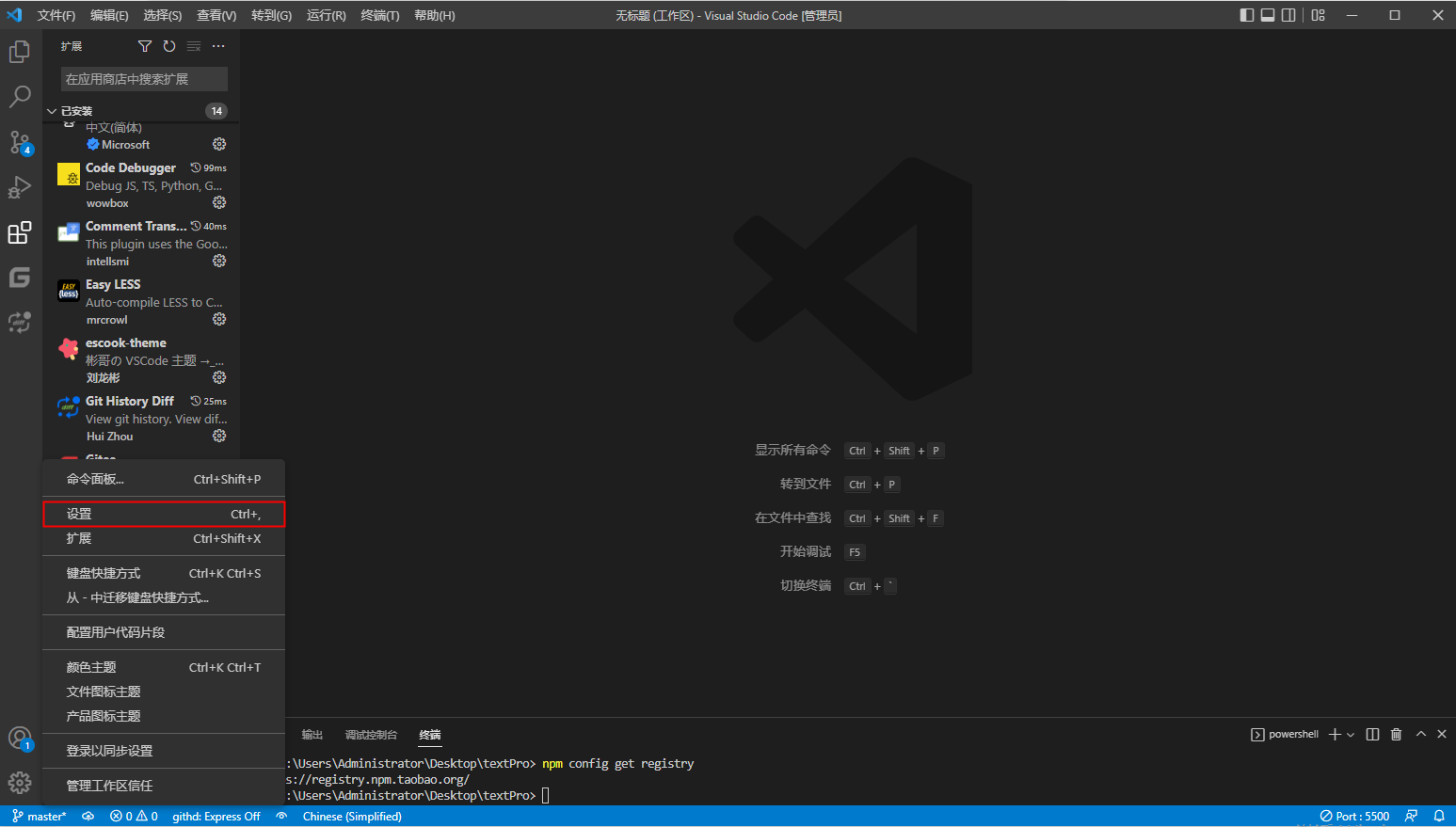
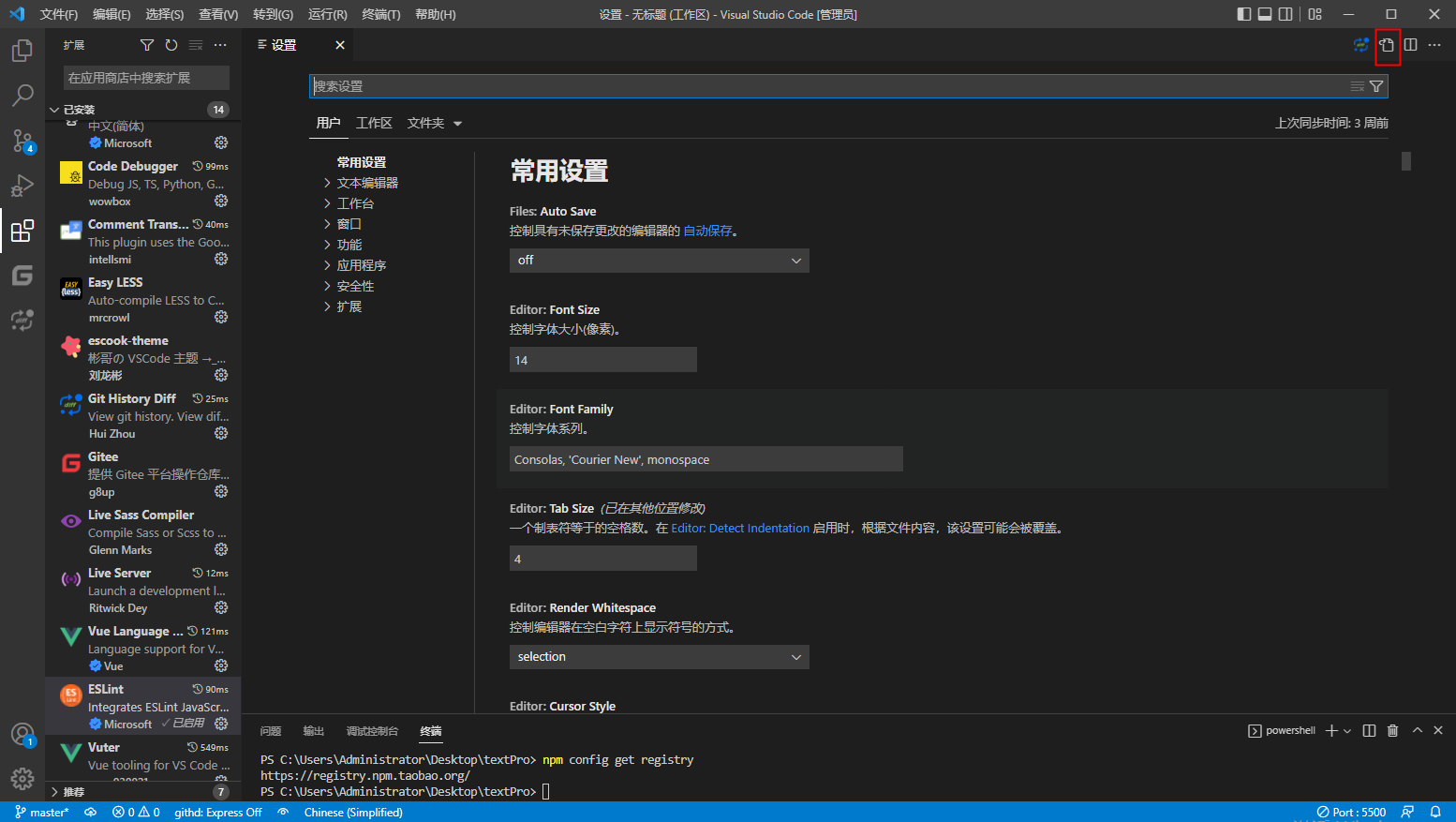
重置样式(normalize.css)
一般浏览器必须将一些最低样式应用于元素,也就是说每个浏览器对初级元素,都有自己设置的初始化样式,而normalize就是可以对不同浏览器的样式进行规范化,删除了浏览器的不一致性,保留了一组优化后 可以依赖的基本样式,在默认的HTML元素样式上提供了跨浏览器的高度一致性
执行 npm i normalize.css 安装重置样式的包
在 main.js
导入 normalize.css
即可
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
import store from './store'
+import 'normalize.css'
createApp(App).use(store).use(router).mount('#app')
公用样式(common.less)
// 重置样式
* {
box-sizing: border-box;
}
html {
height: 100%;
font-size: 14px;
}
body {
height: 100%;
color: #333;
min-width: 1240px;
font: 1em/1.4 'Microsoft Yahei', 'PingFang SC', 'Avenir', 'Segoe UI', 'Hiragino Sans GB', 'STHeiti', 'Microsoft Sans Serif', 'WenQuanYi Micro Hei', sans-serif
}
ul,
h1,
h3,
h4,
p,
dl,
dd {
padding: 0;
margin: 0;
}
a {
text-decoration: none;
color: #333;
outline: none;
}
i {
font-style: normal;
}
input[type="text"],
input[type="search"],
input[type="password"],
input[type="checkbox"]{
padding: 0;
outline: none;
border: none;
-webkit-appearance: none;
&::placeholder{
color: #ccc;
}
}
img {
max-width: 100%;
max-height: 100%;
vertical-align: middle;
}
ul {
list-style: none;
}
#app {
background: #f5f5f5;
user-select: none;
}
.container {
width: 1240px;
margin: 0 auto;
position: relative;
}
.ellipsis {
white-space: nowrap;
text-overflow: ellipsis;
overflow: hidden;
}
.ellipsis-2 {
word-break: break-all;
text-overflow: ellipsis;
display: -webkit-box;
-webkit-box-orient: vertical;
-webkit-line-clamp: 2;
overflow: hidden;
}
.fl {
float: left;
}
.fr {
float: right;
}
.clearfix:after {
content: ".";
display: block;
visibility: hidden;
height: 0;
line-height: 0;
clear: both;
}
在main.js中引用就行
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
import store from './store'
import 'normalize.css'
+import '@/assets/styles/common.less'
createApp(App).use(store).use(router).mount('#app')