https://code.google.com/codejam/contest/4384486/dashboard#s=p0
A
题意
给定一个无向图,其中存在着唯一的一个环,求每个点到这个环的最短距离。
数据范围
≤ T ≤ 100.
1 ≤ xi ≤ N, for all i.
1 ≤ yi ≤ N, for all i.
xi ≠ yi, for all i.
(xi, yi) ≠ (xj, yj), for all i ≠ j.
The graph in which planets are nodes and tubes are edges is connected and has exactly one cycle.
small
3 ≤ N ≤ 30.
large
3 ≤ N ≤ 1000.
题解
显然关键在于找出这个环上的点。找出之后即使是大数据集也可以暴力BFS遍历每个点到这个换的最短距离(O(n^2)),或者可以反过来从环上bfs到所有点(O(n))。
比较暴力的找环方法是直接DFS遍历,然后list存储走过的点,当点的所有child dfs完之后将该点删掉。这样遇到重复的点时这之间的所有点就是该环。
#include<bits/stdc++.h>
using namespace std;
using ll = long long;
vector<int> g[1005];
vector<int> path;
vector<int> circle;
bool visited[1005];
void dfs(int cur, int pa) {
if (visited[cur] == true) {
auto it = find(path.begin(), path.end(), cur);
if (it != path.end()) {
while (it != path.end()) {
circle.push_back(*it);
it++;
}
}
return;
}
visited[cur] = true;
path.push_back(cur);
for (int &child : g[cur]) {
if (child != pa) {
dfs(child, cur);
}
}
path.pop_back();
}
void solve() {
int n;
cin >> n;
int x, y;
for (int i = 1; i <= n; i++) {
g[i].clear();
}
path.clear();
circle.clear();
memset(visited, false, sizeof(visited));
for (int i = 1; i <= n; i++) {
cin >> x >> y;
g[x].push_back(y);
g[y].push_back(x);
}
dfs(1, -1);
vector<int> dis(n+1, n+1);
queue<int> q;
int temp = circle.size();
for (int i = 0; i < temp; i++) {
//cout << circle[i] << "\n";
dis[circle[i]] = 0;
q.push(circle[i]);
}
int cur = 1;
while (!q.empty()) {
int sz = q.size();
for (int i = 0; i < sz; i++) {
int top = q.front();
q.pop();
for (int &child : g[top]) {
if (dis[child] > cur) {
dis[child] = cur;
q.push(child);
}
}
}
cur++;
}
printf("%d",dis[1]);
for (int i = 2; i <= n; i++) {
printf(" %d", dis[i]);
}
printf("\n");
}
int main() {
freopen("A-large.in", "r", stdin);
freopen("A-large.out", "w", stdout);
int t;
cin >> t;
for (int tt = 1; tt <= t; tt++) {
printf("Case #%d: ", tt);
solve();
}
return 0;
}
B
题意
求从一个无向图中拆出的边能组成的凸边形个数。其中拆出的便不能有相同的端点。
数据范围
0 ≤ Li, j ≤ 1000 for all i, j.
Li, i = 0, for all i.
Li, j = Lj, i, for all i, j.
small
N = 6
large
6<=N<=15
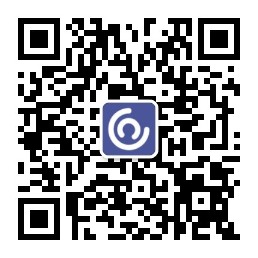
题解
对小数据集,显然最多只能拆出三条边组成三角形,暴力即可。
大数据集不会,待补。
C
题意
给一个数组,对数组中的所有连续子数组按以下公式求k次和,然后求和最后所有的结果(modulo 1000000007)。
对连续子数组Aj, Aj+1, ..., Ak,第i次求和公式为 Aj × 1i + Aj+1 × 2i + Aj+2 × 3i + ... + Ak × (k-j+1)i.
样例:
In Sample Case #1, the Parameter Array is [3, 2]. All the contiguous subarrays are [3], [2], [3, 2].
For i = 1:
1-st Exponential-power of [3] = 3 × 11 = 3
1-st Exponential-power of [2] = 2 × 11 = 2
1-st Exponential-power of [3, 2] = 3 + 2 × 21 = 7
So POWER1 is 12.
For i = 2:2-nd Exponential-power of [3] = 3 × 12 = 3
2-nd Exponential-power of [2] = 2 × 12 = 2
2-nd Exponential-power of [3, 2] = 3 + 2 × 22 = 11
So POWER2 is 16.
For i = 3:3-rd Exponential-power of [3] = 3 × 13 = 3
3-rd Exponential-power of [2] = 2 × 13 = 2
3-rd Exponential-power of [3, 2] = 3 + 2 × 23 = 19
So POWER3 is 24.So answer is 24 + 12 + 16 = 53.
数据范围
1 ≤ T ≤ 100.
1 ≤ x1 ≤ 1e5.
1 ≤ y1 ≤ 1e5
1 ≤ C ≤ 1e5.
1 ≤ D ≤ 1e5.
1 ≤ E1 ≤ 1e5.
1 ≤ E2 ≤ 1e5.
1 ≤ F ≤ 1e5.
small
1 ≤ N ≤ 100.
1 ≤ K ≤ 20.
large
1 ≤ N ≤ 1e6.
1 ≤ K ≤ 1e4
题解
首先根据xycdef生成数组A。
对于小数据集暴力即可。O(k*n^3)
对于大数据集,可以考虑每个数对最后结果的贡献,大力推推就可以出来了。
考虑第i次计算,则数组A中第j位计算的值为:A[j] * (N+1-j) * {1^i + 2^i + .... + j^i} ( j从1开始) 。直接枚举的话复杂度O(k * n^2lgk) ,当然第j^i求和可以累加,能降到O(knlgk) 。
另一种思路是调换一下求和顺序,则ans=sum_{i=1..j} {A[j] (N+1-j) * sum_{i=1..k}{1^i + 2^i + .... + j^i}}。其中m^i求和可以用等比数列公式,再通过累加可以降到O(k*lgk)。(lgk为求幂时的复杂度)
#include<bits/stdc++.h>
using namespace std;
using ll = long long;
const ll mod = 1e9 + 7;
ll pw(ll a, ll b) {
ll ans = 1;
while (b) {
if (b&1) ans = ans * a % mod;
b /= 2;
a = a * a % mod;
//cout << ans << " " << a << "\n";
}
return ans;
}
ll calc(ll a, ll b) {
//cout << pw(a, b) << "\n";
ll ans = a * ((pw(a, b) - 1 + mod) % mod) % mod * pw(a-1, mod-2) % mod;
//cout << ans <<"\n";
}
ll solve() {
ll n, k;
cin >> n >>k;
vector<ll> nums(n+1);
ll x, y;
cin >> x >> y;
ll c,d,e1,e2,f;
cin >> c >> d >> e1 >> e2 >> f;
nums[1] = (x+y) % f;
for (int i = 2; i <= n; i++) {
ll tx = x, ty = y;
x = (c*tx+d*ty+e1) %f;
y = (d*tx+c*ty+e2) %f;
nums[i] = (x+y) % f;
}
ll ans = nums[1]*n%mod*k%mod;
ll sum = k;
//cout << ans <<"\n";
for (int i = 2; i <= n; i++) {
sum = (sum + calc(i, k)) % mod;
//cout << sum << "\n";
ans = (ans + nums[i] * (n+1-i) % mod * sum % mod) % mod;
//cout << i << " " << ans << "\n";
}
return ans;
}
int main() {
freopen("C-large-practice.in", "r", stdin);
freopen("C-large.out", "w", stdout);
int t;
cin >> t;
for (int tt = 1; tt <= t; tt++) {
std::cerr << tt << "\n";
printf("Case #%d: %lld\n", tt, solve());
}
return 0;
}