实例场景
点击Save按钮后,查看保存的文件
点击Load按钮后加载文档数据
Json介绍https://www.json.org/json-zh.htmlUnity中自带的JsonUtility可以将可序列化对象与Json格式相互转换。
将对象转为可序列化对象需要添加[SerializeField],且为public,然后才可以被转为Json格式。
JsonUtility内部API
JsonUtility.ToJson将object对象转为Json格式
//两种重载
public static string ToJson(object obj);
public static string ToJson(object obj, bool prettyPrint);
//obj为被转换为Json格式的对象
//prettyPrint为是否将输出的Json文本转为适合阅读的格式,默认false,尽量不选true,对性能有影响
//返回值为Json格式的string数据
JsonUtility.FromJson将Json格式转为object格式
public static T FromJson<T>(string json);
//T为泛型,代各类数据
//json为json格式的数据
//返回值为某格式的对象
JsonUtility.FromJsonOverwrite通过读取对象的 JSON 表示形式覆盖其数据
扫描二维码关注公众号,回复:
14312079 查看本文章
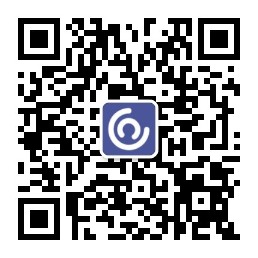
public static void FromJsonOverwrite(string json, object objectToOverwrite);
//json为对象的json格式
//objectToOverwrite为被重写的对象
这个方法与JsonUtility.FromJson不同在于:不产生新的对象加载Json格式,而是在已有的对象内加载Json格式,无需进行任何分配即可更新存储在类或对象中的值。
输入输出流
命名空间:using System.IO
File.WriteAllText 写入文件
public static void WriteAllText (string path, string ?contents);
//path:文件路径
//contents:文件内容
path一般指定为:Application.persistentDataPath,避免平台不同发生错误
该值是目录路径;此目录中可以存储每次运行要保留的数据。在 iOS 和 Android 上发布时,persistentDataPath 指向设备上的公共目录。应用程序更新不会擦除此位置中的文件。用户仍然可以直接擦除这些文件。
如果文件已经存在,则会将第二次输入的内容覆盖到原文件中,不会创建新文件。
File.ReadAllText 读取文件
public static string ReadAllText (string path);
//path:文件路径
//返回值为json格式的字符串
File.ReadAllText 读取文件
public static void ReadAllText (string path);
try—catch语句
源码
PlayerSystem
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class PlayerSystem : MonoBehaviour
{
public List<Text> text = new List<Text>();
[SerializeField] string playerName;
[SerializeField] string playerMoney;
[SerializeField] string playerLevel;
[SerializeField] string playerScore;
const string PLAYER_FILE_NAME = "playerFile";
void Update() {
text[0].text = playerName;
text[1].text = playerMoney;
text[2].text = playerLevel;
text[3].text = playerScore;
}
public void Save(){
var player = new Player();
player.playerName = playerName;
player.playerMoney = playerMoney;
player.playerLevel = playerLevel;
player.playerScore = playerScore;
SaveFile.SaveByJson(PLAYER_FILE_NAME,player);
}
public void Load(){
Player savePlayer = SaveFile.LoadFromJson<Player>(PLAYER_FILE_NAME);
playerName = savePlayer.playerName;
playerMoney = savePlayer.playerMoney;
playerLevel = savePlayer.playerLevel;
playerScore = savePlayer.playerScore;
}
[UnityEditor.MenuItem("Developer/Delete Player Prefabs")]
public static void DeletePlayerSavaFiles(){
SaveFile.DeleteSaveFile(PLAYER_FILE_NAME);
}
[SerializeField] class Player{
public string playerName;
public string playerMoney;
public string playerLevel;
public string playerScore;
}
}
SaveSystem
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
public class SaveFile
{
//存储文件
public static void SaveByJson(string fileName,object data){
var json = JsonUtility.ToJson(data);
var path = Path.Combine(Application.persistentDataPath,fileName);
try{
File.WriteAllText(path,json);
Debug.Log("存储成功");
}
catch(System.Exception e){
Debug.Log("存储失败");
}
}
//加载文件
public static T LoadFromJson<T>(string fileName){
var path = Path.Combine(Application.persistentDataPath,fileName);
try{
var json = File.ReadAllText(path);
var data = JsonUtility.FromJson<T>(json);
Debug.Log("读取成功");
return data;
}
catch(System.Exception e){
Debug.Log("读取失败");
return default;
}
}
//删除文件
public static void DeleteSaveFile(string fileName){
var path = Path.Combine(Application.persistentDataPath,fileName);
try{
File.Delete(path);
Debug.Log("删除成功");
}
catch(System.Exception e){
Debug.Log("删除失败");
}
}
}