Among all the factors of a positive integer N, there may exist several consecutive numbers. For example, 630 can be factored as 3×5×6×7, where 5, 6, and 7 are the three consecutive numbers. Now given any positive N, you are supposed to find the maximum number of consecutive factors, and list the smallest sequence of the consecutive factors.
Input Specification:
Each input file contains one test case, which gives the integer N ( 1 1 1<N< 2 31 2^{31} 231).
Output Specification:
For each test case, print in the first line the maximum number of consecutive factors. Then in the second line, print the smallest sequence of the consecutive factors in the format factor[1]*factor[2]*...*factor[k]
, where the factors are listed in increasing order, and 1 1 1 is NOT included.
Sample Input:
630
Sample Output:
3
5*6*7
Method:
题目阅读理解
Now given any positive N, you are supposed to find the maximum number of consecutive factors,
and list the smallest sequence of the consecutive factors.
给定整数N,寻找最长的连乘因子,如果存在多种方案,输出开始数字最小的方案。
首先判断边界条件,当 n 为素数时,则 n 仅能被 1 和 n 整除,此时连乘因子为 1 和 n,其中 1 不输出。
bool IsPrime(int n) {
if (n < 2) return false;
int limit = int(sqrt(n * 1.0));
for (int i = 2; i <= limit; i++)
if (n % i == 0) return false;
return true;
}
if (IsPrime(n)) {
// 边界条件:n == 素数
cout << "1" << endl;
cout << n << endl;
return 0;
}
然后寻找最小连乘因子的方法难以想到,可以先寻找最小因子。
int i;
for (i = 2; i <= n; i++)
if (n % i == 0) break;
cout << i << endl;
寻找连乘因子需要将 n % i == 0
中因子 i
改为 连乘因子的积 temp
,同时判断条件改为 n % temp != 0
确定连乘因子的右边界。同时记录连乘因子的左边界 first
和连乘因子的长度 len
,以便输出。
int first = 0, len = 0;
for (int j = 2; j <= n; j++) {
int i, temp = 1;
for (i = j; i <= n; i++) {
temp *= i; // 连乘因子的积
if (n % temp != 0) break; // 连乘因子的右边界
}
}
题目仅要求输出最长的连乘因子,所以更新左边界和长度的方法可以使用查找最值的方法,成功AC。
int first = 0, len = 0;
for (int j = 2; j <= n; j++) {
int i, temp = 1;
for (i = 2; i <= n; i++) {
temp *= i;
if (n % temp != 0) break;
}
if (i - j > len) {
first = j;
len = i - j;
}
}
优化判断边界条件为判断 len == 0
,样例 6 会超时。
if (len == 0) {
cout << 1 << endl;
cout << n << endl;
return 0;
}
因为 n 不可能被大于 n \sqrt{n} n 的数整除,可以改进搜索上限为 n \sqrt{n} n,再次AC。
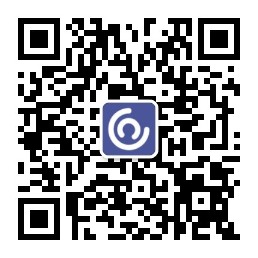
int first = 0, len = 0, limit = int(sqrt(n * 1.0));
for (int j = 2; j <= limit; j++) {
int i, temp = 1;
for (i = 2; i <= limit; i++) {
temp *= i;
if (n % temp != 0) break;
}
if (i - j > len) {
first = j;
len = i - j;
}
}
Solution:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
int n = 0;
cin >> n;
int first = 0, len = 0, limit = int(sqrt(n * 1.0));
for (int j = 2; j <= limit; j++) {
int i, temp = 1;
for (i = j; i <= limit; i++) {
temp *= i;
if (n % temp != 0) break;
}
if (i - j > len) {
first = j;
len = i - j;
}
}
if (len == 0) {
cout << 1 << endl;
cout << n << endl;
return 0;
}
cout << len << endl;
for (int i = 0; i < len; i++) {
cout << first+i;
if (i != len-1) cout << "*";
}
return 0;
}