项目需要展示隐私协议,想用内嵌网页的形式实现。
根据网上获取信息来看,用Cocos引擎的Webview实现起来比较复杂,且不同机型还存在BUG,
遂放弃了这种方式,采用系统原生的Webview实现该功能。
相关代码如下:
iOS平台:
#include "privacyPolicyView.h"
#include "AppController.h"
static privacyPolicyView *instance;
@implementation privacyPolicyView
- (void)loadView:(NSString *)url title:(NSString *)title
{
CGRect screenRect=[UIScreen mainScreen].applicationFrame;
_privacyView=[[UIView alloc]initWithFrame:screenRect];
_privacyView.backgroundColor=[UIColor colorWithRed:1 green:1 blue:1 alpha:1];
[self.rootViewC.view addSubview:_privacyView];
int offsetY=40,lineHight=2;
CGRect rect=screenRect;
rect.origin.y=rect.origin.y+offsetY-lineHight;
rect.size.height=lineHight;
UIView *lineView=[[UIView alloc]initWithFrame:rect];
lineView.backgroundColor=[UIColor colorWithRed:0.5 green:0.5 blue:0.5 alpha:1];
[_privacyView addSubview:lineView];
rect=screenRect;
rect.origin.y=rect.origin.y+offsetY;
rect.size.height=screenRect.size.height-offsetY;
_webView = [[UIWebView alloc] initWithFrame:rect];
NSURL *urlS=[NSURL URLWithString:url];
[_webView loadRequest:[NSURLRequest requestWithURL:urlS]];
[_privacyView addSubview:_webView];
[_webView setScalesPageToFit:YES];
_webView.delegate=self;
UIButton *backbtn = [UIButton buttonWithType:UIButtonTypeRoundedRect];
backbtn.frame = CGRectMake(screenRect.size.width-60, 5, 30, 30);
UIImage *btnImg=[UIImage imageNamed:@"zRes_back.png"];
[backbtn setBackgroundImage:btnImg forState:UIControlStateNormal];
[backbtn setBackgroundImage:btnImg forState:UIControlStateHighlighted];
[backbtn addTarget:self action:@selector(btnClick:) forControlEvents:UIControlEventTouchUpInside];
[_privacyView addSubview:backbtn];
rect=CGRectMake(20, 0, 300, 40);
UILabel *lbl=[[UILabel alloc]initWithFrame:rect];
lbl.font=[UIFont systemFontOfSize:20];
lbl.text=title;
[_privacyView addSubview:lbl];
}
@end
NSString * toNSStr(std::string str)
{
NSString *str_ns = [[NSString alloc] initWithCString:str.c_str()
encoding:NSUTF8StringEncoding];
return str_ns;
}
void loadPrivacyPolicyView(const char* url,const char* title)
{
if (instance) {
[instance loadView:toNSStr(url) title:toNSStr(title)];
}
}
Android平台:
private static String _url="";
private static String _titleStr="";
private static ViewGroup m_layout;
private static LinearLayout m_polBgView,m_lineView;
private static TextView m_titleT;
private static Button m_polBackBtn;
private static WebView m_webView;
public static void loadPolicyView(String url,String titleStr){
_activity._url=url;
_activity._titleStr=titleStr;
_activity.runOnUiThread(new Runnable() {
@Override
public void run() {
//获取屏幕大小
DisplayMetrics dm=new DisplayMetrics();
_activity.getWindowManager().getDefaultDisplay().getMetrics(dm);
int width=dm.widthPixels;
int height=dm.heightPixels;
//获取当前显示view
FrameLayout contentView=(FrameLayout)_activity.getWindow().findViewById(android.R.id.content);
m_layout = (ViewGroup)contentView.getChildAt(0);
int titleHight=40;
m_polBgView=new LinearLayout(_activity);
m_polBgView.setBackgroundColor(Color.rgb(255,255,255));
m_layout.addView(m_polBgView,width,height);
m_lineView=new LinearLayout(_activity);
m_lineView.setBackgroundColor(Color.rgb(128,128,128));
m_lineView.setX(0);
m_lineView.setY(titleHight-2);
m_layout.addView(m_lineView,width,2);
m_titleT=new TextView(_activity);
m_titleT.setText(_activity._titleStr);
m_titleT.setTextSize(12);
m_titleT.setTextColor(Color.rgb(0,0,0));
m_titleT.setX(0);
m_titleT.setY(5);
m_layout.addView(m_titleT,width,titleHight);
m_polBackBtn=new Button(_activity);
m_polBackBtn.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View v){
if(m_layout!=null){
m_layout.removeView(m_polBgView);
m_layout.removeView(m_lineView);
m_layout.removeView(m_titleT);
m_layout.removeView(m_polBackBtn);
m_layout.removeView(m_webView);
}
}
});
m_polBackBtn.setBackgroundResource(R.drawable.zres_back);
m_polBackBtn.setX(width-50);
m_polBackBtn.setY(5);
m_layout.addView(m_polBackBtn,34,30);
m_webView = new WebView(_activity);
WebSettings settings = m_webView.getSettings();
settings.setJavaScriptEnabled(true);
settings.setDefaultTextEncodingName("UTF-8");
//自适应手机屏幕
settings.setUseWideViewPort(true);
settings.setLoadWithOverviewMode(true);
m_webView.loadUrl(_activity._url);
m_webView.setY(titleHight);
m_layout.addView(m_webView,width,height-titleHight);
m_webView.setWebViewClient(new WebViewClient() {
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
view.loadUrl(url);
return super.shouldOverrideUrlLoading(view, url);
}
public void onReceivedSslError(WebView view, SslErrorHandler handler, SslError error) {
//handler.cancel(); // Android默认的处理方式
handler.proceed(); // 接受所有网站的证书
//handleMessage(Message msg); // 进行其他处理
}
});
}
});
}
APP内调用展示网页:
#if (CC_TARGET_PLATFORM==CC_PLATFORM_IOS)
#import "privacyPolicyViewCpp.h"
#endif
#if(CC_TARGET_PLATFORM == CC_PLATFORM_ANDROID)
#include <jni.h>
#include "platform/android/jni/JniHelper.h"
#endif
void HelloWorld::menuCloseCallback(Ref* pSender)
{
const char* url="https://www.baidu.com";
const char* title="Privacy Privacy";
#if (CC_TARGET_PLATFORM==CC_PLATFORM_IOS)
loadPrivacyPolicyView(url, title);
#endif
#if (CC_TARGET_PLATFORM == CC_PLATFORM_ANDROID)
JniMethodInfo t;
if (JniHelper::getStaticMethodInfo(t, "org/cocos2dx/cpp/AppActivity", "loadPolicyView", "(Ljava/lang/String;Ljava/lang/String;)V")) {
jstring str0 = t.env->NewStringUTF(url);
jstring str1 = t.env->NewStringUTF(title);
t.env->CallStaticVoidMethod(t.classID, t.methodID, str0, str1);
t.env->DeleteLocalRef(str0);
t.env->DeleteLocalRef(str1);
t.env->DeleteLocalRef(t.classID);
}
#endif
}
实现的效果:
完整代码 点击获取
扫描二维码关注公众号,回复:
13226506 查看本文章
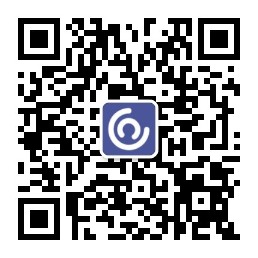
参考资料: