概述
- 学习地址:https://tianchi.aliyun.com/specials/promotion/aicampml?invite_channel=3&accounttraceid=baca918333cb45008b70655b544a5aeadgkm
- 学习内容:机器学习算法(一): 基于逻辑回归的分类预测
- 问题:逻辑回归计算中存在很多算法,单对具体的算法过程不清楚,只知道逻辑回归的使用步骤。比如:
LogisticRegression
中设置项有一项random_state
,但我不清楚设置这个随机数对算法的影响与作用。 - 总结:复习整理了sklearn中逻辑回归的应用,用实践的练习对理论知识做了补充。
逻辑回归算法
一、逻辑回归的优缺点
- 优点:实现简单,易于理解和实现;计算代价不高,速度很快,存储资源低;
- 缺点:容易欠拟合,分类精度可能不高
二、sklearn的调用
逻辑回归模型函数: sklearn.linear_model.LogisticRegression
from sklearn.linear_model import LogisticRegression
lr = LogisticRegression()
(一)Method:
1. fit
lr.fit(X, y[, sample_weight])
Fit the model according to the given training data.
- Ruturn: 这一命令,返回的是
self
2. predict_proba(X)
lr.predict_proba(X)
Probability estimates.
The returned estimates for all classes are ordered by the label of classes.
- Ruturn: array-like of shape (n_samples, n_classes)
Returns the probability of the sample for each class in the model, where classes are ordered as they are inself.classes_
(二)Attributes
1. classes_
lr_classes_
classes_: ndarray of shape (n_classes, )
A list of class labels known to the classifier.
2. coef_
lr_coef_
coef_: ndarray of shape (1, n_features) or (n_classes, n_features)
Coefficient of the features in the decision function.
coef_
is of shape (1, n_features) when the given problem is binary. In particular, when multi_class='multinomial'
, coef_
corresponds to outcome 1 (True) and -coef_
corresponds to outcome 0 (False).
3. intercept_
lr.intercept_
intercept_: ndarray of shape (1,) or (n_classes,)
Intercept (a.k.a. bias) added to the decision function.
If fit_intercept
is set to False, the intercept is set to zero. intercept_
is of shape (1,) when the given problem is binary. In particular, when multi_class='multinomial'
, intercept_
corresponds to outcome 1 (True) and -intercept_
corresponds to outcome 0 (False).
三、代码实现
Part 1 Demo实践
Step1:库函数导入
## 基础函数库
import numpy as np
## 导入画图库
import matplotlib.pyplot as plt
import seaborn as sns
## 导入逻辑回归模型函数
from sklearn.linear_model import LogisticRegression
Step2:模型训练
##Demo演示LogisticRegression分类
## 构造数据集
x_fearures = np.array([[-1, -2], [-2, -1], [-3, -2], [1, 3], [2, 1], [3, 2]])
y_label = np.array([0, 0, 0, 1, 1, 1])
## 调用逻辑回归模型
lr_clf = LogisticRegression()
## 用逻辑回归模型拟合构造的数据集
lr_clf = lr_clf.fit(x_fearures, y_label) #其拟合方程为 y=w0+w1*x1+w2*x2
Step3:模型参数查看
## 查看其对应模型的w
print('the weight of Logistic Regression:',lr_clf.coef_)
## 查看其对应模型的w0
print('the intercept(w0) of Logistic Regression:',lr_clf.intercept_)
输出:
the weight of Logistic Regression: [[0.73455784 0.69539712]]
the intercept(w0) of Logistic Regression: [-0.13139986]
Step4:数据和模型可视化
1. 可视化构造的数据样本点
## 可视化构造的数据样本点
plt.figure()
plt.scatter(x_fearures[:,0],x_fearures[:,1], c=y_label, s=50, cmap='viridis')
plt.title('Dataset')
plt.show()
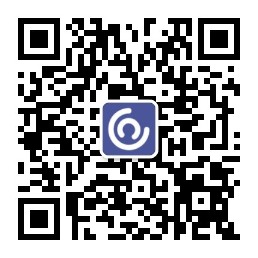
2.可视化决策边界
# 可视化决策边界
plt.figure()
plt.scatter(x_fearures[:,0],x_fearures[:,1], c=y_label, s=50, cmap='viridis')
plt.title('Dataset')
nx, ny = 200, 100
x_min, x_max = plt.xlim() # return the current xlim
y_min, y_max = plt.ylim()
x_grid, y_grid = np.meshgrid(np.linspace(x_min, x_max, nx),np.linspace(y_min, y_max, ny))
z_proba = lr_clf.predict_proba(np.c_[x_grid.ravel(), y_grid.ravel()])
z_proba = z_proba[:, 1].reshape(x_grid.shape)
plt.contour(x_grid, y_grid, z_proba, [0.5], linewidths=2., colors='blue')
plt.show()
思路:其中选择横纵坐标构成的所有点带入预测好的等高线中,选择logistic回归中概率都为0.5的等高线做分割。
- 注:
np.meshgrid(*xi, **kwargs)
Return coordinate matrices from coordinate vectors. 从坐标向量中返回坐标矩阵。
二维坐标系中,X轴可以取三个值1,2,3, Y轴可以取三个值7,8, 请问可以获得多少个点的坐标?
显而易见是6个:
(1,7)(2,7)(3,7)
(1,8)(2,8)(3,8)
#coding:utf-8
import numpy as np
# 坐标向量
a = np.array([1,2,3])
# 坐标向量
b = np.array([7,8])
# 从坐标向量中返回坐标矩阵
# 返回list,有两个元素,第一个元素是X轴的取值,第二个元素是Y轴的取值
res = np.meshgrid(a,b)
#返回结果: [array([ [1,2,3] [1,2,3] ]), array([ [7,7,7] [8,8,8] ])]
np.r_
是按列连接两个矩阵,就是把两矩阵上下相加,要求列数相等。
np.c_
是按行连接两个矩阵,就是把两矩阵左右相加,要求行数相等。
3. 可视化预测新样本
### 可视化预测新样本
plt.figure()
## new point 1
x_fearures_new1 = np.array([[0, -1]])
plt.scatter(x_fearures_new1[:,0],x_fearures_new1[:,1], s=50, cmap='viridis')
plt.annotate(s='New point 1',xy=(0,-1),xytext=(-2,0),color='blue',arrowprops=dict(arrowstyle='-|>',connectionstyle='arc3',color='red'))
## new point 2
x_fearures_new2 = np.array([[1, 2]])
plt.scatter(x_fearures_new2[:,0],x_fearures_new2[:,1], s=50, cmap='viridis')
plt.annotate(s='New point 2',xy=(1,2),xytext=(-1.5,2.5),color='red',arrowprops=dict(arrowstyle='-|>',connectionstyle='arc3',color='red'))
## 训练样本
plt.scatter(x_fearures[:,0],x_fearures[:,1], c=y_label, s=50, cmap='viridis')
plt.title('Dataset')
# 可视化决策边界
plt.contour(x_grid, y_grid, z_proba, [0.5], linewidths=2., colors='blue')
plt.show()
注:plt.annotate()
arrowprops
: The properties used to draw a FancyArrowPatch arrow between the positions xy and xytext.- 创建两个点之间的连接路径。 这由
connectionstyle
键值控制。
Step5:模型预测
## 在训练集和测试集上分别利用训练好的模型进行预测
y_label_new1_predict = lr_clf.predict(x_fearures_new1)
y_label_new2_predict = lr_clf.predict(x_fearures_new2)
print('The New point 1 predict class:\n',y_label_new1_predict)
print('The New point 2 predict class:\n',y_label_new2_predict)
## 由于逻辑回归模型是概率预测模型(前文介绍的 p = p(y=1|x,\theta)),所以我们可以利用 predict_proba 函数预测其概率
y_label_new1_predict_proba = lr_clf.predict_proba(x_fearures_new1)
y_label_new2_predict_proba = lr_clf.predict_proba(x_fearures_new2)
print('The New point 1 predict Probability of each class:\n',y_label_new1_predict_proba)
print('The New point 2 predict Probability of each class:\n',y_label_new2_predict_proba)
- 结果:
The New point 1 predict class:
[0]
The New point 2 predict class:
[1]
The New point 1 predict Probability of each class:
[[0.67507358 0.32492642]]
The New point 2 predict Probability of each class:
[[0.11029117 0.88970883]]
可以发现训练好的回归模型将X_new1预测为了类别0(判别面左下侧),X_new2预测为了类别1(判别面右上侧)。其训练得到的逻辑回归模型的概率为0.5的判别面为上图中蓝色的线。
Part 2 基于鸢尾花(iris)数据集的逻辑回归分类实践
- 数据介绍:本次我们选择鸢花数据(iris)进行方法的尝试训练,该数据集一共包含5个变量,其中4个特征变量,1个目标分类变量。共有150个样本,目标变量为 花的类别 其都属于鸢尾属下的三个亚属,分别是山鸢尾 (Iris-setosa),变色鸢尾(Iris-versicolor)和维吉尼亚鸢尾(Iris-virginica)。包含的三种鸢尾花的四个特征,分别是花萼长度(cm)、花萼宽度(cm)、花瓣长度(cm)、花瓣宽度(cm),这些形态特征在过去被用来识别物种。
变量 | 描述 |
---|---|
sepal length | 花萼长度(cm) |
sepal width | 花萼宽度(cm) |
petal length | 花瓣长度(cm) |
petal width | 花瓣宽度(cm) |
target | 鸢尾的三个亚属类别,‘setosa’(0), ‘versicolor’(1), ‘virginica’(2) |
Step1:库函数导入
## 基础函数库
import numpy as np
import pandas as pd
## 绘图函数库
import matplotlib.pyplot as plt
import seaborn as sns
Step2:数据读取/载入
## 我们利用 sklearn 中自带的 iris 数据作为数据载入,并利用Pandas转化为DataFrame格式
from sklearn.datasets import load_iris
data = load_iris() #得到数据特征
iris_target = data.target #得到数据对应的标签
iris_features = pd.DataFrame(data=data.data, columns=data.feature_names) #利用Pandas转化为DataFrame格式
Step3:数据信息简单查看
## 利用.info()查看数据的整体信息
iris_features.info()
<class ‘pandas.core.frame.DataFrame’>
RangeIndex: 150 entries, 0 to 149
Data columns (total 4 columns):
sepal length (cm) 150 non-null float64
sepal width (cm) 150 non-null float64
petal length (cm) 150 non-null float64
petal width (cm) 150 non-null float64
dtypes: float64(4)
memory usage: 4.8 KB
## 进行简单的数据查看,我们可以利用 .head() 头部.tail()尾部
iris_features.head()
iris_features.tail()
## 其对应的类别标签为,其中0,1,2分别代表'setosa', 'versicolor', 'virginica'三种不同花的类别。
iris_target
## 利用value_counts函数查看每个类别数量
pd.Series(iris_target).value_counts()
2 50
1 50
0 50
dtype: int64
## 对于特征进行一些统计描述
iris_features.describe()
Step4:可视化描述
1. pairplot
从上图可以发现,在2D情况下不同的特征组合对于不同类别的花的散点分布,以及大概的区分能力。
## 合并标签和特征信息
iris_all = iris_features.copy() ##进行浅拷贝,防止对于原始数据的修改
iris_all['target'] = iris_target
iris_all.rename(columns={
"sepal length (cm)": "萼片长",
"sepal width (cm)":"萼片宽",
"petal length (cm)":"花瓣长",
"petal width (cm)":"花瓣宽",
"target":"种类"},inplace=True)
## 特征与标签组合的散点可视化
sns.set_style('white',{
'font.sans-serif':['simhei','Arial']})
sns.pairplot(data=iris_all,diag_kind='hist', hue= "种类")
plt.show()
注:
sns.pairplot
-
pairplot中pair是成对的意思,pairplot主要展现的是变量两两之间的关系(线性或非线性,有无较为明显的相关关系);
-
可以看到对角线上是各个属性的直方图(分布图),而非对角线上是两个不同属性之间的相关图;
-
kind
:用于控制非对角线上的图的类型,可选"scatter"与"reg" -
diag_kind
:控制对角线上的图的类型,可选"hist"与"kde"(直方图与核密度图) -
hue
:针对某一字段进行分类(本例中针对 ’种类‘)
2. 箱型图
利用箱型图我们也可以得到不同类别在不同特征上的分布差异情况。
for col in iris_all.columns[0:4]:
sns.boxplot(x='种类', y=col, saturation=0.5,palette='pastel', data=iris_all)
plt.title(col)
plt.show()
注:
sns.boxplot
箱形图(Box-plot)又称为盒须图、盒式图或箱线图,是一种用作显示一组数据分散情况资料的统计图。它能显示出一组数据的最大值、最小值、中位数及上下四分位数。
3. 三维散点图
# 选取其前三个特征绘制三维散点图
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure(figsize=(10,8))
ax = fig.add_subplot(111, projection='3d')
iris_all_class0 = iris_all[iris_all['种类']==0].values
iris_all_class1 = iris_all[iris_all['种类']==1].values
iris_all_class2 = iris_all[iris_all['种类']==2].values
# 'setosa'(0), 'versicolor'(1), 'virginica'(2)
ax.scatter(iris_all_class0[:,0], iris_all_class0[:,1], iris_all_class0[:,2],label='setosa')
ax.scatter(iris_all_class1[:,0], iris_all_class1[:,1], iris_all_class1[:,2],label='versicolor')
ax.scatter(iris_all_class2[:,0], iris_all_class2[:,1], iris_all_class2[:,2],label='virginica')
plt.legend()
plt.show()
Step5: 利用 逻辑回归模型 在二分类上 进行训练和预测
## 为了正确评估模型性能,将数据划分为训练集和测试集,并在训练集上训练模型,在测试集上验证模型性能。
from sklearn.model_selection import train_test_split
## 选择其类别为0和1的样本 (不包括类别为2的样本)
iris_features_part = iris_features.iloc[:100] #是基于索引位来选取数据集,0:4就是选取 0,1,2,3这四行
iris_target_part = iris_target[:100]
## 测试集大小为20%, 80%/20%分
x_train, x_test, y_train, y_test = train_test_split(iris_features_part, iris_target_part, test_size = 0.2, random_state = 2020)
注:
参数解释:
train_data
:待划分样本数据
train_target
:待划分样本数据的结果(标签)
test_size
:测试数据占样本数据的比例,若整数则样本数量
random_state
:设置随机数种子,保证每次都是同一个随机数。若为0或不填,则每次得到数据都不一样
## 从sklearn中导入逻辑回归模型
from sklearn.linear_model import LogisticRegression
## 定义 逻辑回归模型
clf = LogisticRegression(random_state=0, solver='lbfgs')
注:
solver
:{’newton-cg’ , ‘lbfgs’, ‘liblinear’, ‘sag’, ‘saga’}, 分别为几种优化算法。默认为‘liblinear’。random_state
:int型,RandomState或None,默认为‘None’,仅在算法为 ‘sag’ 或 ’liblinear’ 时使用。
查看其对应的参数
## 查看其对应的w
print('the weight of Logistic Regression:',clf.coef_)
## 查看其对应的w0
print('the intercept(w0) of Logistic Regression:',clf.intercept_)
在训练集和测试集上分布利用训练好的模型进行预测
## 在训练集和测试集上分布利用训练好的模型进行预测
train_predict = clf.predict(x_train)
test_predict = clf.predict(x_test)
测量准确度
from sklearn import metrics
## 利用accuracy(准确度)【预测正确的样本数目占总预测样本数目的比例】评估模型效果
print('The accuracy of the Logistic Regression is:',metrics.accuracy_score(y_train,train_predict))
print('The accuracy of the Logistic Regression is:',metrics.accuracy_score(y_test,test_predict))
## 查看混淆矩阵 (预测值和真实值的各类情况统计矩阵)
confusion_matrix_result = metrics.confusion_matrix(test_predict,y_test)
print('The confusion matrix result:\n',confusion_matrix_result)
# 利用热力图对于结果进行可视化
plt.figure(figsize=(8, 6))
sns.heatmap(confusion_matrix_result, annot=True, cmap='Blues')
plt.xlabel('Predicted labels')
plt.ylabel('True labels')
plt.show()
The accuracy of the Logistic Regression is: 1.0
The accuracy of the Logistic Regression is: 1.0
The confusion matrix result:
[[ 9 0]
[ 0 11]]
我们可以发现其准确度为1,代表所有的样本都预测正确了。
注:
精确率Precision=a/(a+c)=TP/(TP+FP),
召回率recall=a/(a+b)=TP/(TP+FN),
准确率accuracy=(a+d)/(a+b+c+d)=(TP+TN)/(TP+FN+FP+TN),
annot
: 默认为False,为True的话,会在格子上显示数字
Step6: 利用 逻辑回归模型 在三分类(多分类)上 进行训练和预测
测试集大小为20%, 80%/20%分
## 测试集大小为20%, 80%/20%分
x_train, x_test, y_train, y_test = train_test_split(iris_features, iris_target, test_size = 0.2, random_state = 2020)
定义 逻辑回归模型
## 定义 逻辑回归模型
clf = LogisticRegression(random_state=0, solver='lbfgs')
在训练集上训练逻辑回归模型
# 在训练集上训练逻辑回归模型
clf.fit(x_train, y_train)
查看估计参数
## 查看其对应的w
print('the weight of Logistic Regression:\n',clf.coef_)
## 查看其对应的w0
print('the intercept(w0) of Logistic Regression:\n',clf.intercept_)
## 由于这个是3分类,所有我们这里得到了三个逻辑回归模型的参数,其三个逻辑回归组合起来即可实现三分类。
the weight of Logistic Regression:
[[-0.43476516 0.88611834 -2.18912786 -0.94014751]
[-0.38565072 -2.66413298 0.75758301 -1.38261087]
[-0.00811281 0.11312037 2.52971372 2.35084911]]
the intercept(w0) of Logistic Regression:
[ 6.26404984 8.32611826 -16.63593196]
模型预测:
## 在训练集和测试集上分布利用训练好的模型进行预测
train_predict = clf.predict(x_train)
test_predict = clf.predict(x_test)
## 由于逻辑回归模型是概率预测模型(前文介绍的 p = p(y=1|x,\theta)),所有我们可以利用 predict_proba 函数预测其概率
train_predict_proba = clf.predict_proba(x_train)
test_predict_proba = clf.predict_proba(x_test)
print('The test predict Probability of each class:\n',test_predict_proba)
## 其中第一列代表预测为0类的概率,第二列代表预测为1类的概率,第三列代表预测为2类的概率。
## 利用accuracy(准确度)【预测正确的样本数目占总预测样本数目的比例】评估模型效果
print('The accuracy of the Logistic Regression is:',metrics.accuracy_score(y_train,train_predict))
print('The accuracy of the Logistic Regression is:',metrics.accuracy_score(y_test,test_predict))
查看混淆矩阵
## 查看混淆矩阵
confusion_matrix_result = metrics.confusion_matrix(test_predict,y_test)
print('The confusion matrix result:\n',confusion_matrix_result)
# 利用热力图对于结果进行可视化
plt.figure(figsize=(8, 6))
sns.heatmap(confusion_matrix_result, annot=True, cmap='Blues')
plt.xlabel('Predicted labels')
plt.ylabel('True labels')
plt.show()
通过结果我们可以发现,其在三分类的结果的预测准确度上有所下降,其在测试集上的准确度为: 86.67% ,这是由于’versicolor’(1)和 ‘virginica’(2)这两个类别的特征,我们从可视化的时候也可以发现,其特征的边界具有一定的模糊性(边界类别混杂,没有明显区分边界),所有在这两类的预测上出现了一定的错误。