题目一:BEEF MCNUGGETS
/**********************************************
**Description: Given N objects with weight w[i],
** calculate the MAXIMAL weight which can't be made with these objects
** If NONE can't be made, output 0;
** If there exists a weight of INFINITE can't be made, output 0;
**Algorithm: KNAPSACK/DP
**Firstly, it's KNAPSACK problem
**In MATHEMATICS, we know that a equation
** ax+by=c, where gcd(a,b)=1, a>0, b>0;
** a posivesolution exists if c>=a*b
** PROOF: from Extended Euclidean, we know that there exists a solution ax0+by0=c
** we want to find xn, yn, where xn>0, yn>0. xn = x0 + b*t, yn = y0 - a*t;
** -x0/b <= t <= y0/a。for c >= a*b, we know that x0/b+y0/a>=1, so it exists
**For this problem, if GCD(w[i]) > 1 || w[i] == 1, we should print 0;
**Otherwise, the UPPER LIMIT is the maximal two numbers' multiplication.
**So, 256*256*10 = 655360, can be worked out in 0.00s
***********************************************/
题目二:FENCE RAILS
/**************************************************************************************************************
**Description: Given N bags and M objects, find the maximal objects that can be put into bags
** N <= 50, M <= 1023, Mi <= 128
**Analysis: it's a "high dimentionally multiple knapsack problem" which can't be solved by DP.
** **DFS works** so we use DFS* to search which CUT can be used effectively
** BUT it's not enough, we use binary search for DFS*
**CUT(TREE-PRUNING)
** 1. if K objects work, then we choose the minest K objects optimally;
** 2. "finding a board from which to cut a longer rail is more difficult than finding a board for a shorter rail,
** we will perform the search in such that the longest rail is cut first"
** 3. "ALSO,if two rails are of the same length, then cutting the first from board A and the second from board B
is the same as cutting the first from board B and the second from board A, so within sets of rails of the same length,
we will ensure that the rails are cut from boards of non-decreasing index."
** 4. (A REALLY EFFICIENT CUT which makes time to 0.00s)
If, when cutting a board, we get a length of board less than the shortest rail,
it is USELESS, and we can discard it from consideration.
This reduces the total amount of board-feet left from which to cut the rest of the rails.
****************************************************************************************************************/
题目三: FENCE LOOPS
/*********************************************************************************************
**Desctiption: Given the edge relationships, work out the MINIMAL CYCLE(without direction)
**Analysis: 1.The first hard thing is to transform the format of the graph from edges to points.
1. let every edge have two points, k*2 and k*2-1, and store the relationship of edges
in con[i][j] = 1 or 2, with 1 means j is the left side of i, then 2 in reverse
2. MERGE THE POINTS
some points are the same so we have to merge them, we have two different ways
ver[i] means that the point i in the original is ver[i] in the new graph
1.use recursive method to color the vertex in a new number, which makes up a function
2.MERGE, first deal with edges
their efficiency is different, M1 use (2E)^2 and M2 use O(aE), so M2 is better, but M1 also OK as it doesn't affect the final complexity
2.How to get the MINIMAL CYCLE with MATRIX formed
1. use dijsktra, first remove one edge, then get the shortest distance between these points plused edge weight to update the minimal
2. use FOLYED, before updating using k as a undirect vertex, use k as a neccesary point in a cycle, the PROOF is based on
that in a optimal cycle, there exists a vertex whose number is maxest, so when k is this number, we must find the cycle
M1 use O(EN^2), and M2 use O(N^3), of course M2 is better
**********************************************************************************************/
USE DIJKSTRA
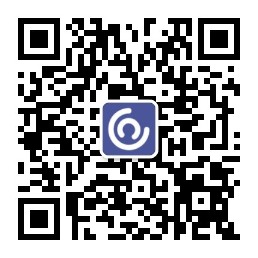
USE FLOYED(带路径输出版)
题目四: CTYPTCOWGRAPH
/*******************************************************************************************
**Firstly, I wan to say that this is a really difficult, but a fairly excellent SEARCH problem.
**There are several CUTS:
**1.the number of C, O, W must be the same
**2.the other characters must be same with the original
**3.the part before 'C' is same as the original, also the suffix with 'W'
**4.the part between single C, O, W must be same with the original
**5.use HASH to identify (Hint : As for it, we know that the first step and the last step are not
neccesary, so we don't use HASH for them.
**6.use decreasing for 'W' all accelerate
**With all the 6 cuts we can cut the time within 1.5s
**From this problem, I have learnt that
**1.the HASH for STRING
**2.the function of STRING, such as
s.size(), s.find(), s.find_first_of, s.find_last_of, s.substr()
**3.cin.getline(c), is for char array, so use s=string(C) to get s
************************************************************************************************
***The time used for last two cases
Test 9: TEST OK [1.107 secs, 12800 KB]
Test 10: TEST OK [1.350 secs, 12800 KB]
***********************************************************************************************/
总结一下4.1 这四道题目都很不错,
A题是带数论的背包;
B题是多为背包;要用搜索去剪枝,而且用的剪枝很巧,这种技巧也很常用;
C题设计图的转化,也很有思想性,自己想出了并查集的方法建图,然后就是求无向图的最小环,是我对FLOYED算法有了更深入的理解;
D题是需要很多剪枝的搜索,写了3遍,现在基本上可以默写出来了,设计到任何可以的剪枝,以及字符串HASH, 枚举顺序问题;
这个难关也艰难地派过来了,主要是以学习为主,在此感谢 NOCOW 网站激烈的讨论和多样的思路 和 maigo 的精简的程序。