界面截图: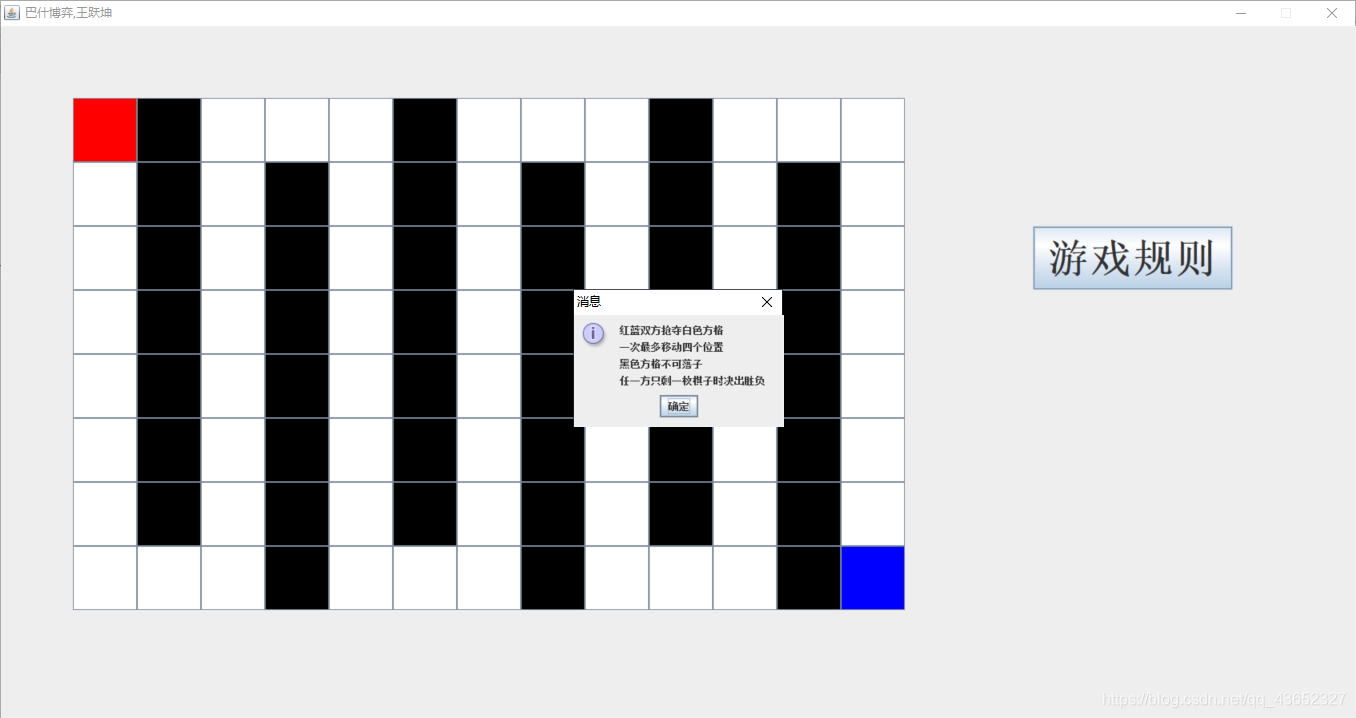
代码:
package 巴什博弈;
import java.awt.*;
import java.awt.event.*;
import java.util.ArrayList;
import javax.swing.*;
public class test extends JFrame implements ActionListener {
private static int width = 13, height = 8, who = 2, maxLen = 4, curplayer1 = 1, curplayer2 = 120702;
private static ArrayList<Integer> list = new ArrayList<Integer>();
private static boolean[][] buf = new boolean[width][height];
private static JButton[][] checker = new JButton[width][height];
private static JButton rule = new JButton("游戏规则");
private static int[][] judge = new int[width][height];
Font font = new Font("宋体", Font.BOLD, 50);
public test() {
setBounds(100, 50, 1700, 900);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
setTitle("巴什博弈,王跃坤");
setLayout(null);
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
if (i % 2 == 0) {
judge[i][j] = 3;
}
}
if (i == 1 || i == 5 || i == 9)
judge[i][7] = 3;
if (i == 3 || i == 7 || i == 11)
judge[i][0] = 3;
}
judge[0][0] = 1;
judge[width - 1][height - 1] = 2;
for (int a = 0; a < width; a++) {
for (int b = 0; b < height; b++) {
checker[a][b] = new JButton();
checker[a][b].setBounds(90 + 80 * a, 90 + 80 * b, 80, 80);
checker[a][b].addActionListener(this);
add(checker[a][b]);
}
}
showImg();
Init(0, 0);
rule.setFont(font);
rule.setBounds(90 + 80 * 15, 90 + 80 * 2, 250, 80);
rule.addActionListener(this);
add(rule);
repaint();
}
public void actionPerformed(ActionEvent event)
{
JButton batton = (JButton) event.getSource();
if (batton == rule) {
JOptionPane.showMessageDialog(this, "红蓝双方抢夺白色方格"+"\n"+"一次最多移动四个位置"+"\n"+"黑色方格不可落子"+"\n"+"任一方只剩一枚棋子时决出胜负");
}
for (int a = 0; a < width; a++) {
for (int b = 0; b < height; b++) {
if (batton == checker[a][b]) {
if (judge[a][b] != 3 && judge[a][b] != who) {
JOptionPane.showMessageDialog(this, "此位置不可落子!");
} else if (len(a, b, who) > maxLen) {
JOptionPane.showMessageDialog(this, "移动距离超出最大长度!");
} else {
change(a, b, who, len(a, b, who));
showImg();
}
}
}
}
if (check() != 0) {
int choice = JOptionPane.showConfirmDialog(this, (check() == 1 ? "红" : "蓝") + "棋赢!!!" + '\n' + "是否重玩游戏。",
"游戏结束", JOptionPane.YES_NO_OPTION);
if (choice == JOptionPane.NO_OPTION) {
this.dispose();
}
if (choice == JOptionPane.YES_OPTION) {
this.dispose();
new test();
}
}
who = 3 - who;
machineDown();
if (check() != 0) {
int choice = JOptionPane.showConfirmDialog(this, (check() == 1 ? "红" : "蓝") + "棋赢!!!" + '\n' + "是否重玩游戏。",
"游戏结束", JOptionPane.YES_NO_OPTION);
if (choice == JOptionPane.NO_OPTION) {
this.dispose();
}
if (choice == JOptionPane.YES_OPTION) {
this.dispose();
new test();
}
}
who = 3 - who;
}
public static void machineDown() {
int count = 0;
for (int t : list) {
if (t % 100 == 3)
count++;
}
int mylen = count % (maxLen + 1);
int find = -1;
if (who == 1) {
find = list.get(list.indexOf(curplayer1) + mylen);
} else {
find = list.get(list.indexOf(curplayer2) + mylen);
}
change(find / 10000, (find / 100) % 100, who, mylen);
showImg();
}
public static int check() {
if (list.get(1) % 100 == 2)
return 2;
else if (list.get(list.size() - 2) % 100 == 1)
return 1;
else
return 0;
}
public static void change(int x, int y, int who, int len) {
int begin = 0;
if (who == 1)curplayer1 = (int) (x * 10000 + y * 100 + who);
else curplayer2 = (int) (x * 10000 + y * 100 + who);
int p = 0;
int t;
int tail = 1;
for (int i = 0; i < list.size(); i++) {
t = list.get(i);
t /= 100;
t *= 100;
if (t / 100 == curplayer2 / 100) {
tail = 2;
}
t += tail;
judge[t / 10000][(t / 100) % 100] = tail;
list.set(i, t);
if (t == curplayer1) {
tail = 3;
}
}
}
public static void showImg() {
for (int a = 0; a < width; a++) {
for (int b = 0; b < height; b++) {
if(judge[a][b]==0){
checker[a][b].setBackground(Color.black);
}else if(judge[a][b]==1){
checker[a][b].setBackground(Color.red);
}else if(judge[a][b]==2){
checker[a][b].setBackground(Color.blue);
}else if(judge[a][b]==3){
checker[a][b].setBackground(Color.white);
}
}
}
}
public static int len(int x, int y, int who) {
int t = list.indexOf((int) (x * 10000 + y * 100 + judge[x][y]));
if (who == 1) {
return Math.abs(list.indexOf(curplayer1) - t);
} else {
return Math.abs(list.indexOf(curplayer2) - t);
}
}
public static void Init(int x, int y) {
buf[x][y] = true;
list.add(x * 10000 + y * 100 + judge[x][y]);
if (x - 1 >= 0 && x - 1 < width && judge[x - 1][y] > 0 && (buf[x - 1][y] == false)) {
Init(x - 1, y);
}
if (x + 1 >= 0 && x + 1 < width && judge[x + 1][y] > 0 && (buf[x + 1][y] == false)) {
Init(x + 1, y);
}
if (y - 1 >= 0 && y - 1 < height && judge[x][y - 1] > 0 && (buf[x][y - 1] == false)) {
Init(x, y - 1);
}
if (y + 1 >= 0 && y + 1 < height && judge[x][y + 1] > 0 && (buf[x][y + 1] == false)) {
Init(x, y + 1);
}
}
public static void main(String[] args) {
new test();
}
}