加载收盘价和成交量
获取数据
close,amount=np.loadtxt("data.csv",delimiter=",",usecols=(6,7),unpack=True)
print("收盘价:\n",close)----------------------->
收盘价:
[336.1 339.32 345.03 344.32 343.44 346.5 351.88 355.2 358.16 354.54
356.85 359.18 359.9 363.13 358.3 350.56 338.61 342.62 342.88 348.16
353.21 349.31 352.12 359.56 360. 355.36 355.76 352.47 346.67 351.99]
print("成交量:\n",amount)---------------------->
成交量:
[21144800. 13473000. 15236800. 9242600. 14064100. 11494200. 17322100.
13608500. 17240800. 33162400. 13127500. 11086200. 10149000. 17184100.
18949000. 29144500. 31162200. 23994700. 17853500. 13572000. 14395400.
16290300. 21521000. 17885200. 16188000. 19504300. 12718000. 16192700.
18138800. 16824200.]
1->成交量加权平均价:np.average(arg1,weights=arg2)
arg1:价格,arg2:成交量为权重。
某个价格的成交量越高,该价格所占的权重就越大。VWAP就是以成交量
为权重计算出来的加权平均值,常用于算法交易
average=np.average(close,weights=amount)
print("成交量加权平均价:\n",average)------------>成交量加权平均价:350.5895493532009
2->计算算术平均值:np.mean(ndarry)
ndarry:价格数组
mean=np.mean(close)
average=np.average(close)
print("收盘价的平均价格:",mean)----------------->收盘价的平均价格: 351.0376666666667
print(average)------------------------------------------------>351.0376666666667
3->求最大值和最小值 np.max(arg1),np.min(arg1)
max=np.max(close)
min=np.min(close)
print("成交量最大值:",max)--------------------->成交量最大值: 363.13
print("成交量最小值:",min)--------------------->成交量最小值: 336.1
4.->计算数组中的极差(最大值与最小值的差)np.ptp()
获取数据
high=np.loadtxt("data.csv",delimiter=",",usecols=(4,))
print(high)------------------------------->
[344.4 340.04 345.65 345.25 344.24 346.7 353.25 355.52 359. 360.
357.8 359.48 359.97 364.9 360.27 359.5 345.4 344.64 345.15 348.43
355.05 355.72 354.35 359.79 360.29 361.67 357.4 354.76 349.77 352.32]
计算股票最高价的极差
ptp=np.ptp(high)
print("股票的极差:",ptp)------------------>股票的极差: 24.859999999999957
利用最大值和最小值得到极差
ptp2=np.max(high)-np.min(high)
print(ptp2)------------------------------>24.859999999999957
5.->求数组的中位数np.median()
close=np.loadtxt("data.csv",delimiter=",",usecols=(6,))
median=np.median(close)
print("中位数:",median)------------------->中位数: 352.055
验证352.055是否为中位数?
if len(close)%2==0:
result=(close[len(close)//2]+close[len(close)//2-1])/2
else:
result=close[len(close)//2]
print(result)--------------------------->352.055
6.->计算数据样本的方差 np.var()
方差越小代表越稳定
close=np.loadtxt("data.csv",delimiter=",",usecols=(6,))
open=np.loadtxt("data.csv",delimiter=",",usecols=(3,))
print("收盘价的方差:",np.var(close))------>收盘价的方差: 50.126517888888884
print("开盘价的方差:",np.var(open))------->开盘价的方差: 50.402024888888896
7.计算股票收益率、年波动率及月波动率
获取股票数据
close=np.loadtxt("data.csv",delimiter=",",usecols=(6,))
print(close)----------------------------->
[336.1 339.32 345.03 344.32 343.44 346.5 351.88 355.2 358.16 354.54
356.85 359.18 359.9 363.13 358.3 350.56 338.61 342.62 342.88 348.16
353.21 349.31 352.12 359.56 360. 355.36 355.76 352.47 346.67 351.99]
(1)计算简单的收益率:相邻两天的差除以前一天的价格。returns = np.diff( arr ) / arr[ : -1]
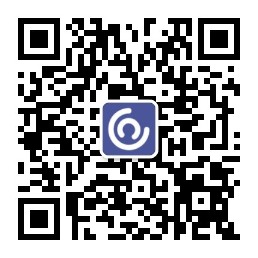
returns=np.diff(close)/close[:-1]
print(np.diff(close).size)--------------->29 #查看元素个数
print(returns)--------------------------->
[ 0.00958048 0.01682777 -0.00205779 -0.00255576 0.00890985 0.0155267
0.00943503 0.00833333 -0.01010721 0.00651548 0.00652935 0.00200457
0.00897472 -0.01330102 -0.02160201 -0.03408832 0.01184253 0.00075886
0.01539897 0.01450483 -0.01104159 0.00804443 0.02112916 0.00122372
-0.01288889 0.00112562 -0.00924781 -0.0164553 0.01534601]
(2)对数收益率#对收益取以e为底的对数
logReturns=np.diff(np.log(close))
print(logReturns)----------------------->
[ 0.00953488 0.01668775 -0.00205991 -0.00255903 0.00887039 0.01540739
0.0093908 0.0082988 -0.01015864 0.00649435 0.00650813 0.00200256
0.00893468 -0.01339027 -0.02183875 -0.03468287 0.01177296 0.00075857
0.01528161 0.01440064 -0.011103 0.00801225 0.02090904 0.00122297
-0.01297267 0.00112499 -0.00929083 -0.01659219 0.01522945]
(3)对数收益率的标准差
std=np.std(np.log(close))
print(std)----------------------------->0.02024386952568129
(4)年波动率
年波动率等于对数收益率的标准差除以其均值,再除以交易日倒
数的平方根,通常交易日取252天。
year_annual_volatility=std/np.mean(logReturns)/np.sqrt(1/252)
(5)月波动率
月波动率等于对数收益率的标准差除以其均值,再除以交易月倒数的平
方根,交易月为12月。
month_annual_volatility=std/np.mean(logReturns)/np.sqrt(1./12.)
筛选出对数收益率大于0的所有元素索引
indices=np.where(logReturns>0)
print("收益率大于0的索引为:\n",indices)---->收益率大于0的索引为:
(array([ 0, 1, 4, 5, 6, 7, 9, 10, 11, 12, 16, 17, 18, 19, 21, 22, 23,
25, 28], dtype=int64),)
根据索引值,获取相应的数组元素
result=np.take(logReturns,indices)
print("收益率大于0的值有:\n",result)----->收益率大于0的值有:
[[0.00953488 0.01668775 0.00887039 0.01540739 0.0093908 0.0082988
0.00649435 0.00650813 0.00200256 0.00893468 0.01177296 0.00075857
0.01528161 0.01440064 0.00801225 0.02090904 0.00122297 0.00112499
0.01522945]]
8.阶乘
(1)求阶乘 np.prod()
arr=np.array([5,2,7,4])
prod=np.prod(arr)
print("阶乘:",prod)----------------->阶乘: 280
(2)累积乘积 np.cumprod()
cumarr=np.array([2,3,4,5])
cumprod=np.cumprod(cumarr)
print(cumprod)---------------------->[ 2 6 24 120]#将前面所有数相乘的乘积赋值给这个元素
(3)将数组中所有比给定最大值还大的元素全部设定为给定的最大值,而所有比给定最小值还小的元素全部设定为给定的最小值
np.clip(num1,num2) # 介于num1和num2之间的值保持不变
test=np.array([3,56,7,44,22,18])
clip=test.clip(20,48)
print(clip)------------------------>[20 48 20 44 22 20]
(4)np.compress()返回根据一定条件筛选后的数组
close=np.loadtxt("data.csv",delimiter=",",usecols=(6,))
logReturns=np.diff(np.log(close))
print("对数收益率:",logReturns)------>对数收益率:
[ 0.00953488 0.01668775 -0.00205991 -0.00255903 0.00887039 0.01540739
0.0093908 0.0082988 -0.01015864 0.00649435 0.00650813 0.00200256
0.00893468 -0.01339027 -0.02183875 -0.03468287 0.01177296 0.00075857
0.01528161 0.01440064 -0.011103 0.00801225 0.02090904 0.00122297
-0.01297267 0.00112499 -0.00929083 -0.01659219 0.01522945]
<—–>筛选出对数收益率大于0的元素
“`
mask=logReturns>0
print(“mask\n”,mask)—————>mask
[ True True False False True True True True False True True True
True False False False True True True True False True True True
False True False False True]
result=logReturns.compress(logReturns>0)
print(“对数收益率大于0的元素:\n”,result)—>对数收益率大于0的元素:
[0.00953488 0.01668775 0.00887039 0.01540739 0.0093908 0.0082988
0.00649435 0.00650813 0.00200256 0.00893468 0.01177296 0.00075857
0.01528161 0.01440064 0.00801225 0.02090904 0.00122297 0.00112499
0.01522945]
“`-