Directory
What is algorithm analysis?
The theoretical study of computer-program performance and resource usage,especailly for performance.Formally speaking,it is to predict the resource that the algorithms required,including resource for memory as well as for calculating.
- sub-question 1)-> What is more important than algorithms’ performance for engineer?
- memeory cost
- maintainability
- futures
- correctness/preciseness
- security
- conciseness
- function
- modular construction
- ...
- sub-question 2)->Why we have to study algorithm performance?
Ok,this question has reminded me of what the prefessor said in the course.As he said,we can be an excellent c.s. engineer for ten years by solely programing.However, we can achieve such goal just for five years or less if we can study some algorithm while programing.That’s funning,and that’s the point.
Starting from an usual example - Insertion Sort
As we all konw, Insertion-Sort is a kind of algorithm which can sort an disorder array in Θ(n^2).(default from smallest to largest).The pseudocode is as follows:
// InsertionSort
for j ← 2 to n
do key ← A[j]
i ← j-1
while i > 0 and A[i] >key
do A[i+1] ← A[i]
i ← i-1
A[i+1] ← key
It’s easy to find some rules of running time as follows:
1) Depends on the input distribution (e.g. The arrays has already been sorted. VS disorder)
2) Depends on the input size (e.g. 6 elems VS 6*10^9 elems)
3) We ofen foucs on the upper running time for it's a guarteen to users.
A Insertion-Sort code using java is provided.
package nov19;
import java.util.Arrays;
public class InsortionSortTester {
public static void main(String[] args) {
int[] a = {1,0,5,2,6,3,6,8,1};
InsertionSort(a);
System.out.println(Arrays.toString(a));
}
public static void InsertionSort(int[] A)
{
for(int i=0;i<A.length;i++)
{
int j = i-1;
int key = A[i];
while(j>0 && key<A[j])
{
A[j+1] = A[j];
j--;
}
A[j+1] = key;
}
}
}
Anaylsis method for running time
- The worst-case running time(focus on usually)
In this case,T(n) = max time on any case of size n
The reasons why this case is often focus on is as follows:
1. It's an upper bound on the running time for any input,knowing that gives us a guarteen that the algorithm will never take any longer.
2. The worst-case fairly often,such as seraching for absent element in an array.
3. Average-case is like worst-case honestly.
What’s more, relative speed is more relaible than absolute speed.
-
The average-case
In this case,T(n) = excepted time over all inputs of size n.
There,excepted time,which means the sum of each input multiples its corresponding probability of occurance.Here,we make an assumption that the input distribution is an uniform distribution. -
The best-case (bogus)
It’s meaningless,althogh it can cheat sometimes. -
A big idea->Asymptotic anaylsis
- Ignore machine dependent constrain.
- Look at the growth of T(n)
- Asymptotic notation Θ
- drop low-order terms
- ignore leading constants
- Ex:T(n) = 4n^3+5n ^2+3n+1000 = Θ(n ^3)
Ending with MergeSort
Sorting steps:
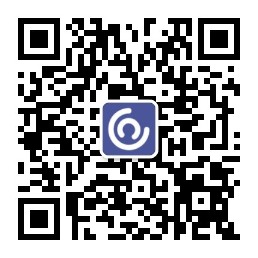
- When n is 1, the sorting is done.
- When n is greater than 1, then recursively sort A[1~n/2] and A[n/2+1 ~n].
- Merge the sorted two tables, compare the two tables, and take the smallest one into the output sequence.