本部分内容是用c++实现队列的顺序存储结构:
一、首先我们要弄明白的几个内容:
1、实现队列的顺序存储结构是非常的简单的,是用固定长度的数组进行实现。
2、明白两个指针:front和rear。
front:队头指针,rear:队尾指针;tips:rear不一定大于front.如下图:
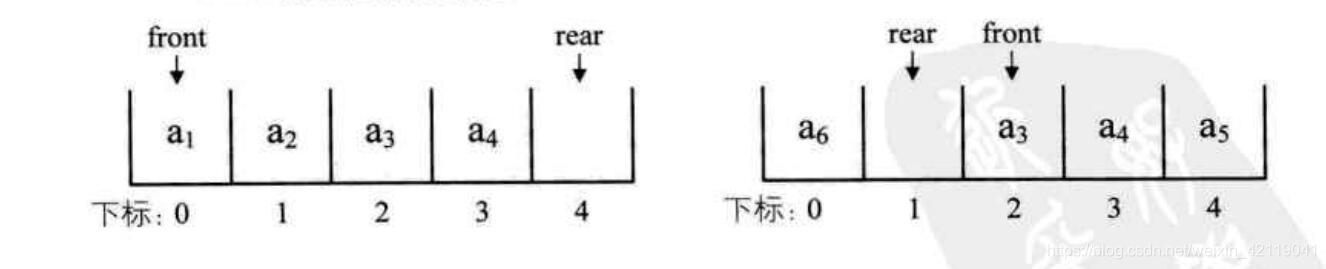
3、三个等式:
(1)队列为空的条件:
front == rear
(2)队列满的条件:
(rear + 1)% QueueSize == front
(3)队列长度计算:
size = (rear - front + QueueSize) % QueueSize
二、实现代码:
1、首先是头文件(类定义)部分:
#pragma once
#include <iostream>
#define QUEUESIZE 100
using namespace std;
template <class ElemType>
class Queue
{
public:
Queue();
~Queue();
int getSize();
void enQueue(ElemType &e);
void deQueue();
void display() const;
bool isEmpty() const;
bool isFull() const;
private:
ElemType queue[QUEUESIZE];
int front;
int rear;
int m_size;
};
2、其次是函数定义部分:
#include "Queue.h"
template <class ElemType>
Queue<ElemType>::Queue():front(0),rear(0),m_size(0){
}
template <class ElemType>
Queue<ElemType>::~Queue(){
}
template<class ElemType>
int Queue<ElemType>::getSize(){
this->m_size = (rear - front + QUEUESIZE) % QUEUESIZE;
return m_size;
}
template<class ElemType>
void Queue<ElemType>::enQueue(ElemType &e){
if ((rear + 1) % QUEUESIZE == front) {
cout << "队列已满,不能插入新元素" << endl;
return;
}else {
queue[rear] = e;
rear = (rear + 1) % QUEUESIZE;
}
}
template<class ElemType>
void Queue<ElemType>::deQueue(){
if (front == rear) {
cout << "空队列,无元素" << endl;
return;
}
ElemType e;
e = queue[front];
this->front = (this->front + 1) % QUEUESIZE;
}
template<class ElemType>
void Queue<ElemType>::display() const{
if (front == rear) {
cout << "队列中无元素" << endl;
return;
}else if (rear > front) {
for (size_t i = front; i < rear; ++i)
cout << queue[i] << " ";
}else {
for (size_t j = front; j < QUEUESIZE -1; ++j)
cout << queue[j] << " ";
for (size_t k = 0; k < rear; ++k)
cout << queue[k] << " ";
}
}
template<class ElemType>
bool Queue<ElemType>::isEmpty() const {
if (this->m_size == 0)
return true;
return false;
}
template<class ElemType>
bool Queue<ElemType>::isFull() const{
if (this->m_size == 0)
return false;
return true;
}
3、最后是函数测试部分(main函数):
#include "Queue.cpp"
int main()
{
Queue<int> queue;
cout << "*********************" << endl;
cout << "新初始化的队列的长度为:" << queue.getSize() << endl;
cout << "队是否为空:" << boolalpha << queue.isEmpty() << endl;
cout << "*********************" << endl;
for (int i = 0; i < 10; ++i)
queue.enQueue(i);
cout << "插入元素后队列的长度:" << queue.getSize() << endl;
cout << "队是否为空:" << boolalpha << queue.isEmpty() << endl;
cout << "整个队列元素:" << endl;
queue.display();
cout << endl;
cout << "*********************" << endl;
queue.deQueue();
cout << "删除元素后的的队列的长度:" << queue.getSize() << endl;
cout << "队是否为空:" << boolalpha << queue.isEmpty() << endl;
cout << "整个队列元素:" << endl;
queue.display();
cout << endl;
system("pause");
return 0;
}
4、最后是测试结果:
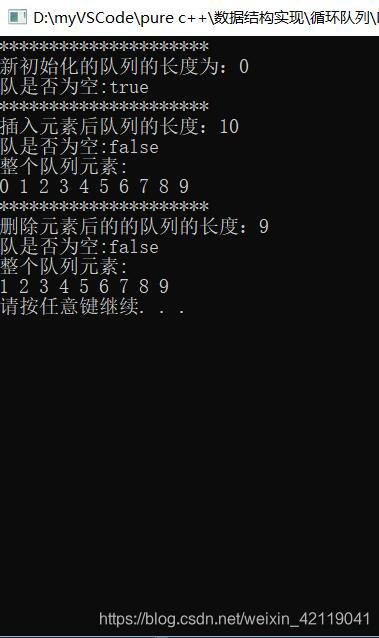