c++实现栈的顺序存储结构的方法和实现顺序线性表的操作差不多,甚至要比实现线性表还要简单一点,因为栈可以认为是只允许在表尾(栈顶)进行插入和删除操作的线性表,只在表尾就很方便操作
话不多说,直接代码:
首先是头文件部分:
#pragma once
#include <iostream>
#define MAXSIZE 100
using namespace std;
template <class ElemType>
class MyStack
{
public:
MyStack();
virtual ~MyStack();
bool isEmpty() const;
bool isFull() const;
int getSize() const;
void push(ElemType &e);
void pop();
void display();
ElemType stack[MAXSIZE];
int top;
int size;
};
然后是函数的定义:
#include "MyStack.h"
template <class ElemType>
MyStack<ElemType>::MyStack(){
this->top = -1;
this->size = 0;
}
template <class ElemType>
MyStack<ElemType>::~MyStack(){
}
template<class ElemType>
bool MyStack<ElemType>::isEmpty() const
{
if (this->top == -1) {
return true;
}else {
return false;
}
}
template<class ElemType>
bool MyStack<ElemType>::isFull() const{
if (top >= MAXSIZE) {
cout << "栈满" << endl;
return true;
}else {
return false;
}
}
template <class ElemType>
int MyStack<ElemType>::getSize() const {
return this->size;
}
template<class ElemType>
void MyStack<ElemType>::push(ElemType & e){
if (top == MAXSIZE - 1) {
cout << "栈满,不能插入" << endl;
return;
}
this->top++;
stack[top] = e;
this->size++;
}
template <class ElemType>
void MyStack<ElemType>::pop() {
if (this->top == -1) {
cout << "栈空" << endl;
}
ElemType e;
e = stack[this->top];
this->top--;
--size;
}
template<class ElemType>
void MyStack<ElemType>::display(){
for (size_t i = 0; i < size; ++i) {
cout << stack[i] << " ";
}
cout << endl;
}
最后是验证部分(main函数):
#include "MyStack.h"
#include "MyStack.cpp"
#include <iostream>
using namespace std;
int main()
{
MyStack<int> stack;
cout << "**********************" << endl;
cout << "初始化的栈:" << endl;
cout << "栈空?" << boolalpha << stack.isEmpty() << endl;
cout << "栈满?" << boolalpha << stack.isFull() << endl;
cout << "**********************" << endl;
cout << "执行进栈操作后:" << endl;
for (int i = 0; i < 10; ++i) {
stack.push(i);
}
stack.display();
cout << "栈中共有" << stack.getSize() << "个元" << endl;
cout << "栈空?" << boolalpha << stack.isEmpty() << endl;
cout << "栈满?" << boolalpha << stack.isFull() << endl;
cout << "***********************" << endl;
cout << "执行出栈操作后:" << endl;
stack.pop();
stack.display();
system("pause");
return 0;
}
程序运行结果如下:
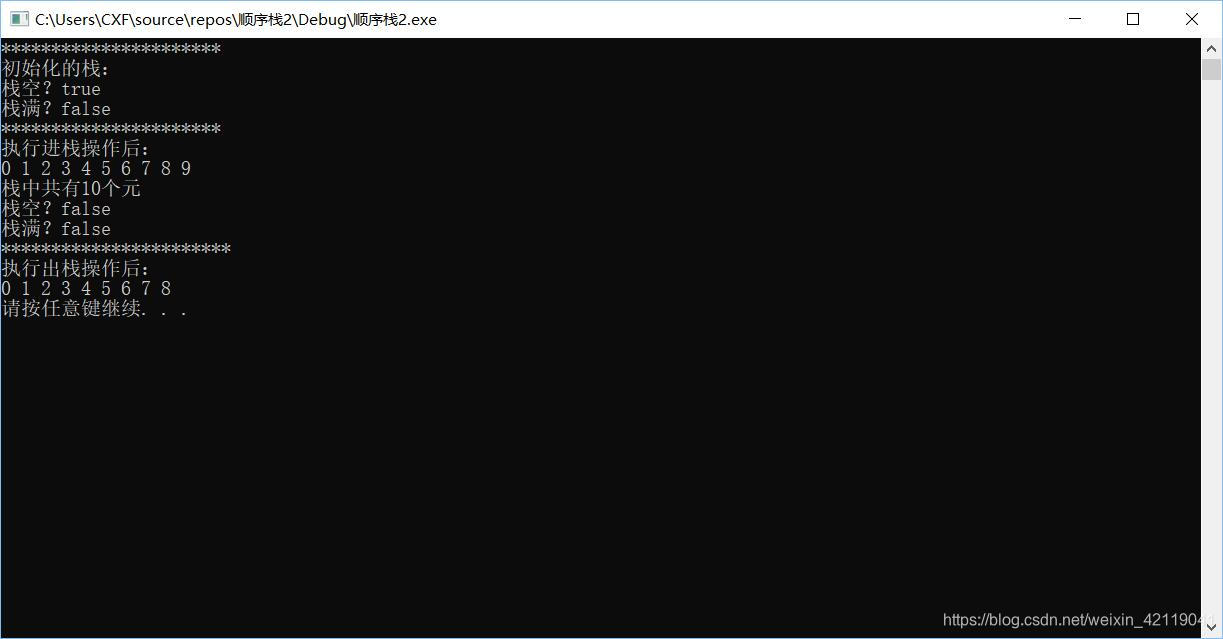