写在前面:上一篇博客已经完成使用c++实现队列的链式存储结构,参见链接:c++ 实现队列的顺序存储结构
自己认为无论是线性表、栈还是队列等等结构,链式结构总是要比顺序结构难一点,因为顺序存储结构就是玩数组嘛,数组大家都能玩的转;
但链式结构肯定是指针操作,而有了指针,程序必将变得复杂,所以实现起来可能稍微有点困难,但是换句话说,链式不就是玩指针嘛,相信大家指针也一定都能玩的转.
话不多说,进入正题:
一、首先还是要搞清楚三个问题:
1、实现队列的链式存储结构就是实现简单的链表:只允许在尾部插入元素,只允许在头部删除元素,这里分别叫入队和出队,
2、明白两个指针:front和rear。
front:队头指针,rear:队尾指针;
3、三个等式:
(1)队列为空的条件依然是:
front == rear;
(2)因为是链式存储,就不存在队满的现象:
二、实现过程(保证可复现):
1、首先是头文件定义(类定义)部分:
#pragma once
#include <iostream>
#include <string>
using namespace std;
template <class ElemType>
struct QueueNode {
ElemType data;
QueueNode *next;
};
template <class ElemType>
class LinkQueue {
public:
LinkQueue();
~LinkQueue();
void enQueue(const ElemType &e);
void deQueue();
bool isEmpty();
void showQueue();
int getSize();
private:
QueueNode<ElemType> *front;
QueueNode<ElemType> *rear;
int size;
};
2、其次是函数定义部分:
#include "linkQueue.h"
template<class ElemType>
inline LinkQueue<ElemType>::LinkQueue(){
this->front = new QueueNode<ElemType>;
if (!front) return;
this->rear = this->front;
this->size = 0;
}
template<class ElemType>
inline LinkQueue<ElemType>::~LinkQueue(){
delete this->front;
}
template<class ElemType>
void LinkQueue<ElemType>::enQueue(const ElemType & e){
QueueNode<ElemType> *s = new QueueNode<ElemType>;
s->data = e;
s->next = nullptr;
this->rear->next = s;
this->rear = s;
this->size++;
}
template<class ElemType>
void LinkQueue<ElemType>::deQueue(){
if (isEmpty()) {
cout << "队列为空队列!" << endl;
return;
}
QueueNode<ElemType> *ptr = this->front->next;
ElemType e;
e = ptr->data;
this->front->next = ptr->next;
if (rear == ptr) {
this->rear = this->front;
}
this->size--;
delete ptr;
}
template<class ElemType>
bool LinkQueue<ElemType>::isEmpty(){
if (front == rear)
return true;
return false;
}
template<class ElemType>
void LinkQueue<ElemType>::showQueue(){
QueueNode<ElemType> *ptr = front->next;
if (isEmpty()) {
cout << "队列为空队列" << endl;
return;
}
while (ptr) {
cout << ptr->data << " ";
ptr = ptr->next;
}
}
template<class ElemType>
int LinkQueue<ElemType>::getSize(){
return this->size;
}
3、最后是函数测试部分(main):
#include <iostream>
#include "linkQueue.cpp"
using namespace std;
int main()
{
LinkQueue<int> lq;
cout << "************************" << endl;
cout << "实例化的空队列:" << endl;
cout << "队列的长度为:" << lq.getSize() << endl;
cout << "************************" << endl;
cout << "执行入队操作后:" << endl;
for (int i = 0; i < 10; ++i) {
lq.enQueue(i);
}
cout << "队列的长度为:" << lq.getSize() << endl;
lq.showQueue();
cout << "************************" << endl;
cout << "执行出队操作后:" << endl;
lq.deQueue();
cout << endl;
cout << "队列的长度为:" << lq.getSize() << endl;
lq.showQueue();
cout << endl;
system("pause");
return 0;
}
4、实现结果:
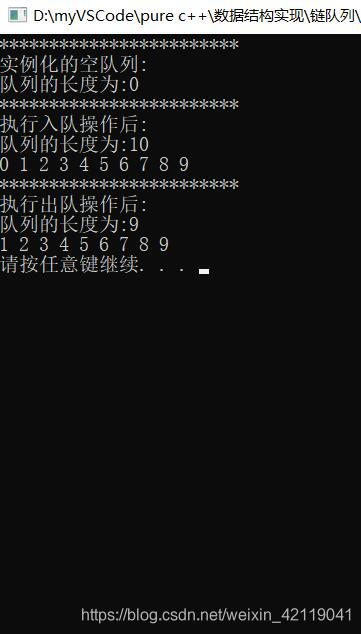