<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="../jquery-3.4.1.min.js"></script>
<link rel="stylesheet" href="../css/植物大战僵尸.css">
</head>
<body>
<div id="container">
<button >stop</button>
<button>begin</button>
<button>加速</button>
<span class="text">boss来临还有:</span>
<span id="countdown"></span>
<div id="bg1" class="bg"></div>
<div id="bg2" class="bg"></div>
<div id="name" onselectstart="return false">植物大战僵尸2.0</div>
<div id="begin"><span>开始游戏</span></div>
<div id="plane"></div>
<div id="img"></div>
<div id="hp"><div class="hpw"></div></div>
<div id="imga"></div>
<div id="big_boss"></div>
</div>
<script src="../js/植物大战僵尸.js"></script>
</body>
</html>
//生成随机数
function randomNum(min, max) {
let num = parseInt(Math.random() * (max - min) + min);
return num;
}
let T = -50;
let ttop = -150;
(function ($) {
$.fn.extend({
//背景循环
bgCycle: function () {
setInterval(function () {
$('#bg1,#bg2').css({top: '+=1'});
if (parseInt($('#bg1').css('top')) > 600) {
$('#bg1').css('top', '0')
}
if (parseInt($('#bg2').css('top')) > 0) {
let count = -600;
$('#bg2').css({top: count})
}
}, 20)
},
//小蜜蜂移动
move: function () {
$("#plane").on('mousedown', function (e) {
let Left = Math.round($("#container").offset().left);//container距离页面left的值
let W = Math.round($('#container').width());
let H = Math.round($('#container').height());
let w = Math.round($('#plane').width());
let h = Math.round($('#plane').height());
let l = parseInt($(this).css("left"));
let t = parseInt($(this).css("top"));
//鼠标和div的间距
let disX = e.pageX - Left - l;
let disY = e.pageY - t;
$(document).on('mousemove', function (e) {
let x = e.pageX - disX - Left;
let y = e.pageY - disY;
if (x < -10) {
x = -10
}
if (x > W - w + 10) {
x = W - w + 10
}
if (y < 0) {
y = 0
}
if (y > H - h) {
y = H - h
}
$('#plane').css({left: x, top: y});
});
$(document).on('mouseup', function () {
$(document).off('mousemove');
})
})
},
//生成子弹
shoot: function () {
creat_time = setInterval(function () {
let newel = $('<div class="shoot"></div>');
let x = parseInt($("#plane").css("left")) + 25;
let y = parseInt($("#plane").css("top"));
newel.css({left: x, top: y});
newel.appendTo($('#container'));
}, 200)
},
//子弹运动
shoot_move: function () {
let s_timer = setInterval(function () {
$('.shoot').css({top: '-=10'});
let allShoot = document.querySelectorAll('.shoot');
for (let i = 0; i < allShoot.length; i++) {
if (allShoot[i].offsetTop < 0) {
allShoot[i].parentNode.removeChild(allShoot[i]);
}
}
}, 30);
},
//点击stop
stop: function () {
$(this).on('click', function () {
for (let i = 0; i < 100; i++) {
clearInterval(i);
}
let audio = document.getElementById('audio');
if (audio.played) {
audio.pause();
}
});
},
//生成敌方
bad: function () {
timer_two = setInterval(() => {
let bad = $('<div class="bad"></div>');
bad.appendTo($('#container'));
bad.css({left: randomNum(0, 350), top: T});
}, 900)
},
//敌方移动
bad_move: function () {
setInterval(() => {
$('.bad').css({top: '+=1'});
if (parseInt($('.bad').css('top')) > 550) {
let over_window = $('<div class="over_window">Game over</div>');
over_window.appendTo($('#container'));
for (let i = 0; i < 100; i++) {
clearInterval(i);
}
let audio = document.getElementById('audio');
if (audio.play) {
audio.pause();
}
$('button').hide();
$('<button class="restart">Try again</button>').appendTo($('#container'));
$(".restart").animate({borderRadius: 30, left: 25, width: 350}, 1000);
$('.restart').on('mouseup', function () {
location.reload();
})
}
}, 10)
},
//boss生成
boss: function () {
// setTimeout(() => {
timer_one = setInterval(() => {
let B = $('<div class="boss"></div>');
B.appendTo($("#container"));
//必须设置B添加到container中,下行用B来设置样式,用$('.boss')设置存在问题
B.css({left: randomNum(0, 250), top: ttop});
}, 1000);
// }, 2000);
},
//boss移动
boss_move: function () {
setInterval(() => {
$('.boss').css({top: '+=1'});
//到达下方
if (parseInt($(".boss").css('top')) > 450) {
$('<div class="over_window">Game over</div>').appendTo($('#container'));
for (let i = 0; i < 100; i++) {
clearInterval(i);
}
let audio = document.getElementById('audio');
if (audio.play) {
audio.pause();
}
$('button').hide();
$('<button class="restart">Try again</button>').appendTo($('#container'));
$(".restart").animate({borderRadius: 30, left: 25, width: 350}, 1000);
$('.restart').on('mouseup', function () {
location.reload();
})
}
}, 10)
},
//boss碰撞检测
boss_check: function () {
function check(obj1, obj2) {
let obj1Left = obj1.offsetLeft;
let obj1Width = obj1.offsetWidth + obj1Left;
let obj1Top = obj1.offsetTop;
let obj1Height = obj1Top + obj1.offsetHeight;
let obj2Left = obj2.offsetLeft;
let obj2Width = obj2.offsetWidth + obj2Left;
let obj2Top = obj2.offsetTop;
let obj2Height = obj2Top + obj2.offsetHeight;
if (!(obj1Left > obj2Width || obj1Width < obj2Left || obj1Top > obj2Height || obj1Height < obj2Top)) {
return true;
} else {
return false;
}
}
setInterval(() => {
let shoot = document.getElementsByClassName('shoot');
let con = document.getElementById('container');
let boss = document.getElementsByClassName('boss');
for (let i = 0; i < shoot.length; i++) {
for (let j = 0; j < boss.length; j++) {
let a = shoot[i];
let b = boss[j];
if (check(a, b)) {
//console.log(parseInt($('.boss').css('fontSize')));
con.removeChild(a);
$('.boss').css({fontSize: '-=20'});
}
if (parseInt($('.boss').css('fontSize')) <= 0) {
con.removeChild(b);
}
}
}
}, 10)
},
//碰撞检测+计分
check_block_collision: function () {
function check(obj1, obj2) {
let obj1Left = obj1.offsetLeft;
let obj1Width = obj1.offsetWidth + obj1Left;
let obj1Top = obj1.offsetTop;
let obj1Height = obj1Top + obj1.offsetHeight;
let obj2Left = obj2.offsetLeft;
let obj2Width = obj2.offsetWidth + obj2Left;
let obj2Top = obj2.offsetTop;
let obj2Height = obj2Top + obj2.offsetHeight;
if (!(obj1Left > obj2Width || obj1Width < obj2Left || obj1Top > obj2Height || obj1Height < obj2Top)) {
return true;
} else {
return false;
}
}
setInterval(() => {
let shoot = document.getElementsByClassName('shoot');
let bad = document.getElementsByClassName('bad');
let con = document.getElementById('container');
for (let i = 0; i < shoot.length; i++) {
for (let j = 0; j < bad.length; j++) {
let a = shoot[i];
let b = bad[j];
if (check(a, b)) {
con.removeChild(a);
con.removeChild(b);
}
}
}
}, 10)
},
//小蜜蜂变身
changebg: function () {
setInterval(function () {
$('#plane').css({
background: 'url("../img/mifeng1.png") no-repeat 5px',
backgroundSize: '100% 100%'
})
}, 500)
},
//背景音乐
mucis: function () {
},
//stop/begin切换
begin: function () {
$('button:first').on('click', function () {
$(this).hide();
$("button:eq(2)").hide();
$('button:eq(1)').css({display: 'block'}).animate({
width: 400,
height: 100,
opacity: 0.8,
fontSize: 50,
top: 200
})
});
$('button:eq(1)').on('click', function () {
$('button:first').show();
$("button:eq(2)").show();
$(this).animate({width: 50, height: 30, top: 0, left: 0, fontSize: 1, opacity: 0});
setTimeout(function () {
for (let i = 0; i < 100; i++) {
clearInterval(i);
}
$('#container').bgCycle();
$('#plane').move();
$('#plane').shoot();
$('#plane').shoot_move();
$('#img').bad();
$('#container').bad_move();
$('#container').check_block_collision();
// $("#plane").changebg();
$('button:first').stop();
$("#button:eq(1)").begin();
$('#img').boss();
$("#img").boss_move();
$("#img").boss_check();
let audio = document.getElementById('audio');
if (audio.pause) {
audio.play();
}
$("#big_boss").big_boss_check();
}, 0)
});
},
//加速
speed: function () {
$('button:eq(2)').on('mousedown', function () {
$('#container').bgCycle();
$('#plane').shoot();
$('#plane').shoot_move();
$('#img').bad();
$('#container').bad_move();
$('#container').boss();
$("#container").boss_move();
})
},
//big boss来临倒计时+boss出现
countdown: function () {
setTimeout(() => {
$(".text").show();
function countDown(maxtime, fn) {
var timer = setInterval(function () {
if (!!maxtime) {
var seconds = Math.floor(maxtime % 60),
msg = seconds;
fn(msg);
--maxtime;
} else {
clearInterval(timer);
fn("boss来临!");
$(".text").hide();
$("#countdown").animate({left: 140, fontSize: 30,})
.animate({fontSize: 40})
.animate({fontSize: 30}).slideToggle();
//boss出现+其他怪消失
clearInterval(timer_one);
clearInterval(timer_two);
setTimeout(() => {
$('#big_boss').show();
$("#hp").show();
$(".hpw").show();
//big_boss出现后点击begin事件
$('button:eq(1)').off().on('click', function () {
$('button:first').show();
$("button:eq(2)").show();
$(this).animate({width: 50, height: 30, top: 0, left: 0, fontSize: 1, opacity: 0});
setTimeout(function () {
for (let i = 0; i < 100; i++) {
clearInterval(i);
}
$('#container').bgCycle();
$('#plane').move();
$('#plane').shoot();
$('#plane').shoot_move();
$('button:first').stop();
let audio = document.getElementById('audio');
if (audio.pause) {
audio.play();
}
$("#big_boss").big_boss_check();
}, 0)
});
//big_boss出现后加速按钮事件变化
$('button:eq(2)').off().on('click', function () {
$('#container').bgCycle();
$('#plane').shoot();
$('#plane').shoot_move();
});
//big_boss_attack
setTimeout(() => {
setInterval(() => {
console.log();
let t = parseInt($('#big_boss').css('bottom'));
let b = $('<div class="attack"></div>');
b.appendTo($('#big_boss'));
b.css({top: t - 200, left: randomNum(0, 250)});
}, 1500)
}, 2000)
}, 2000);
}
}, 1000);
}
let span = document.querySelector('#countdown');
countDown(3, function (msg) {
span.innerHTML = msg;
});
}, 2000)
},
//big_boss check collision
big_boss_check: function () {
function check(obj1, obj2) {
let obj1Left = obj1.offsetLeft;
let obj1Width = obj1.offsetWidth + obj1Left;
let obj1Top = obj1.offsetTop;
let obj1Height = obj1Top + obj1.offsetHeight;
let obj2Left = obj2.offsetLeft;
let obj2Width = obj2.offsetWidth + obj2Left;
let obj2Top = obj2.offsetTop;
let obj2Height = obj2Top + obj2.offsetHeight;
if (!(obj1Left > obj2Width || obj1Width < obj2Left || obj1Top > obj2Height || obj1Height < obj2Top)) {
return true;
} else {
return false;
}
}
tt = setInterval(() => {
let shoot = document.getElementsByClassName('shoot');
let big_boss = document.getElementById('big_boss');
let con = document.getElementById('#container');
for (let i = 0; i < shoot.length; i++) {
let a = shoot[i];
if (check(a, big_boss)) {
a.parentNode.removeChild(a);
$(".hpw").css({width: '-=1'});
}
if (parseInt($(".hpw").css('width')) <= 0) {
clearInterval(tt);
$('#big_boss').animate({opacity: 0}, 2000);
$('#hp').animate({opacity: 0}, 2000);
$('<div class="pass">恭喜过关!</div>').appendTo($("#container"));
clearInterval(creat_time);
}
}
}, 10)
},
//big_boss攻击路线
big_boss_attack: function () {
big_boss_time = setInterval(() => {
if (parseInt($('.attack').css('top')) > 500) {
$('<div class="over_window">Game over</div>').appendTo($('#container'));
for (let i = 0; i < 100; i++) {
clearInterval(i);
}
let audio = document.getElementById('audio');
if (audio.play) {
audio.pause();
}
$('button').hide();
$('<button class="restart">Try again</button>').appendTo($('#container'));
$(".restart").animate({borderRadius: 30, left: 25, width: 350}, 1000);
$('.restart').on('mouseup', function () {
location.reload();
})
}
$('.attack').css({top: '+=1'})
}, 10)
},
//big_boss_attack collision
big_boss_attack_collision: function f() {
function check(obj1, obj2) {
let obj1Left = obj1.offsetLeft;
let obj1Width = obj1.offsetWidth + obj1Left;
let obj1Top = obj1.offsetTop;
let obj1Height = obj1Top + obj1.offsetHeight;
let obj2Left = obj2.offsetLeft;
let obj2Width = obj2.offsetWidth + obj2Left;
let obj2Top = obj2.offsetTop;
let obj2Height = obj2Top + obj2.offsetHeight;
if (!(obj1Left > obj2Width || obj1Width < obj2Left || obj1Top > obj2Height || obj1Height < obj2Top)) {
return true;
} else {
return false;
}
}
attack_time = setInterval(() => {
let shoot = document.getElementsByClassName('shoot');
let attack = document.getElementsByClassName('attack');
let con = document.getElementById('#container');
for (let i = 0; i < shoot.length; i++) {
for (let j = 0; j < attack.length; j++) {
let a = shoot[i];
let b = attack[j];
if (check(a, b)) {
a.parentNode.removeChild(a);
b.parentNode.removeChild(b);
}
if (parseInt($('#big_boss').css('opacity'))<=0){
clearInterval(attack_time);
clearInterval(big_boss_time);
}
}
}
}, 10)
}
})
})(jQuery);
//开始游戏
$('#begin').on('mouseup', function () {
$(this).animate({opacity: 0, top: 800}, 500);
$("#img").animate({opacity: 0}, 1000);
$('#plane').show();
$('#container').bgCycle();
$('#container').move();
$('#plane').shoot();
$('#container').shoot_move();
$('#img').bad();
$('#container').bad_move();
$('#container').check_block_collision();
// $("#plane").changebg();
$('button:first').stop();
$("#button:eq(1)").begin();
$('<audio id="audio" src="../img/植物大战僵尸bgm.mp3" autoplay></audio>').appendTo($('#container'))
$('#name').animate({left: -400}, 1000);
$('button:eq(2)').speed();
$('#imga').animate({top: 600}, 1000);
$('#container').boss();
$("#container").boss_move();
$("#container").boss_check();
$(".text").countdown();
$("#big_boss").big_boss_check();
$("#big_boss").big_boss_attack();
$("#big_boss").big_boss_attack_collision();
});
* {
margin: 0;
padding: 0
}
#container {
position: relative;
width: 400px;
height: 600px;
margin: 0 auto;
background: #a3a3a3;
overflow: hidden;
box-shadow: 0 0 2px 2px rgba(100,100,100,0.5);
}
.bg {
width: 400px;
height: 600px;
position: absolute;
/*background: url("../img/bgthree.jpg");*/
background: url("../img/gamebg_03.png");
/*transform: rotate(90deg);*/
/*background-size: 100% 100%;*/
}
#bg2 {
top: -600px;
}
button:first-child {
width: 50px;
height: 30px;
position: absolute;
z-index: 2;
filter: opacity(50%);
border-radius: 10px;
color: white;
background-color: #11213b;
font-size: 1rem;
}
button:nth-child(2) {
width: 400px;
height: 100px;
position: absolute;
z-index: 1;
filter: opacity(80%);
border-radius: 10px;
color: white;
background-color: #11213b;
font-size: 4rem;
font-weight: bold;
display: none;
top: 200px;
/*background: url("../img/zhiwuda.jpg");*/
/*background-size: 100% 100%;*/
}
button:nth-child(2):hover{
color: #46ffff;
}
button:nth-child(3){
position:absolute;
top: 0;
left: 55px;
width: 50px;
height: 30px;
z-index: 1;
filter: opacity(50%);
border-radius: 10px;
color: white;
background-color: #11213b;
}
#plane {
width: 100px;
height: 80px;
position: absolute;
background: url("../img/豌豆.png") no-repeat;
transform: rotate(-90deg);
background-size: 100% 100%;
top: 400px;
left: 150px;
display: none;
}
.shoot {
width: 20px;
height: 20px;
border-radius: 50%;
background: radial-gradient(farthest-side at 60% 10%, greenyellow, black);
position: absolute;
/*border-top-left-radius: 5px;*/
/*border-top-right-radius: 5px;*/
}
.bad {
width: 50px;
height: 80px;
background: url("../img/bad.png") no-repeat;
background-size: 100%;
position: absolute;
}
.over_window {
position: absolute;
top: 30%;
left: -50px;
width: 500px;
height: 100px;
background: linear-gradient(white 10%, greenyellow 10%, #46ffff);
font-weight: bold;
font-size: 50px;
color: #a3a3a3;
text-align: center;
transform: rotate(-20deg);
}
body {
background: url("../img/gamebg_03.png");
}
#begin {
position: absolute;
top: 300px;
left: -5px;
width: 410px;
height: 100px;
text-align: center;
font-size: 60px;
cursor: pointer;
font-family: 方正粗黑宋简体, serif;
background: linear-gradient(200deg, orange, orchid, wheat, #f4511e, blue);
text-shadow: 0 0 0 #cccccc, 1px 1px 0 #3e8e41, 2px 2px 0 #92bde7, 3px 3px 0 #cccccc, 4px 4px 0 #cccccc, 5px 5px 0 #cccccc;
text-decoration: none;
outline: none;
color: #fff;
border: none;
border-radius: 15px;
box-shadow: 0 9px #999;
}
#begin:hover {
background: linear-gradient(90deg, #727d8f, wheat, black);
}
#begin:active {
background-color: #3e8e41;
box-shadow: 0 5px #666;
transform: translateY(4px);
}
#begin span{
}
#name{
color: yellow;
font-size: 30px;
font-family: "Adobe Arabic";
position: absolute;
top: 20px;
width: 400px;
text-align: center;
font-weight: bold;
}
#img{
position: absolute;
top: 80px;
width: 400px;
height: 220px;
background: url("../img/zhiwuda.jpg");
background-size: 100% 100%;
}
#imga{
position: absolute;
top: 400px;
width: 400px;
height: 200px;
background: url("../img/zhiwuda2.jpg");
background-size: 100% 100%;
}
.restart{
position: absolute;
top: 400px;
width: 250px;
height: 100px;
/*box-shadow: 0 0 1px 1px rgba(000,100,255,0.6);*/
left: 50px;
background: linear-gradient(60deg,darkslateblue 10%,green 50%,darkmagenta);
text-align: center;
font-size: 30px;
font-weight: bold;
color: #d7ddde;
filter: hue-rotate(200deg);
outline: none;
}
.restart:hover{
filter: none;
}
.boss{
position: absolute;
width: 150px;
height: 150px;
background: url("../img/僵尸.png") -10px -50px;
background-size: 135% 130%;
font-size: 100px;
}
.text{
position: absolute;
top: 0;
left: 140px;
z-index: 2;
color: #ffffff;
display: none;
}
#countdown{
position: absolute;
top:0;
left: 250px;
color: #ff0000;
font-size: 15px;
font-weight: bold;
z-index: 2;
}
#big_boss{
position: absolute;
top: 20px;
left: 70px;
width: 320px;
height: 210px;
background: url("../img/僵尸big.png") no-repeat;
background-size: 240% 100%;
background-position: -230px;
display: none;
}
#hp{
position: absolute;
top: 2px;
left: 150px;
border: 1px solid #333333;
width: 100px;
height: 5px;
display: none;
}
.hpw{
width: 100px;
height: 5px;
background: #ff0000;
display: none;
}
.pass{
position:absolute;
width: 400px;
height: 150px;
background: linear-gradient(90deg,violet,green);
top: 200px;
text-align: center;
font-size: 50px;
line-height: 150px;
font-weight: bold;
color: #FFFFFF;
z-index: 5;
}
.attack{
position: absolute;
background: url("../img/feitianjianshi.png") -100px;
background-size: 100% 100%;
width: 100px;
height: 100px;
}
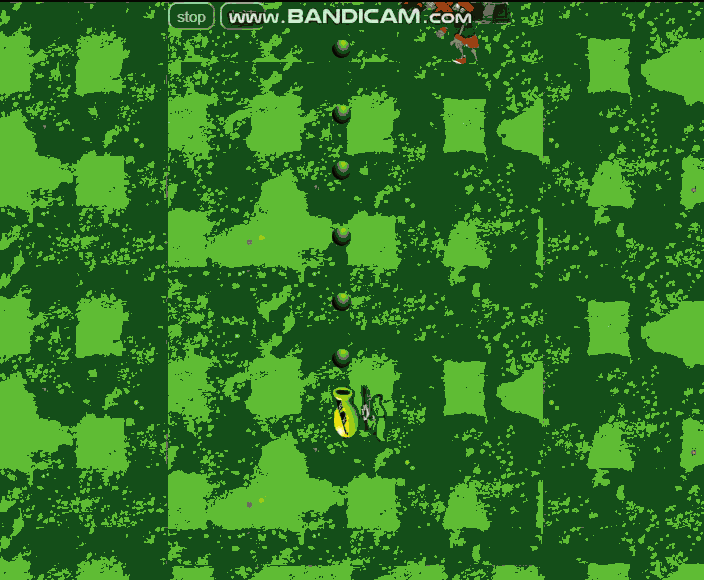