1. 引入依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-activemq</artifactId>
<version>2.1.5.RELEASE</version>
</dependency>
2. application.properties 中书写相关配置
server.port=8081
spring.activemq.broker-url=tcp://192.168.174.8888:61616
spring.activemq.user=admin
spring.activemq.password=admin
#默认是false,为true开启发布订阅模式,同时点对点模式失效
spring.jms.pub-sub-domain=false
3. 相关代码
ActiveMqConf.java
@Component
public class ActiveMqConf {
@Autowired
private JmsTemplate jmsTemplate;
//发送队列消息
public void sendQueueMsg(String destination,String msg) {
jmsTemplate.convertAndSend(destination, msg);
}
//发布订阅消息
public void sendTopicMsg(String destinationMsg,String msg){
Destination destination = new ActiveMQTopic(destinationMsg);
jmsTemplate.convertAndSend(destination,msg);
}
}
ConsumerListener.java
@Component
public class ConsumerListener {
//监听 springboot-queue
@JmsListener(destination = "springboot-queue")
public void consumer1(Message message){
TextMessage textMessage = (TextMessage) message;
System.out.println("监听到的消息: "+textMessage);
}
//监听 springboot-topic
@JmsListener(destination = "springboot-topic")
public void consumer2(Message message){
TextMessage textMessage = (TextMessage) message;
System.out.println("监听到的Topic消息: "+textMessage);
}
@Bean
public Destination getDestination(){
Destination destination = new ActiveMQTopic("springboot-topic");
return destination;
}
}
ActiveMqController.java
@RestController
@RequestMapping("queue")
public class ActiveMqController {
@Autowired
ActiveMqConf activeMqConf;
//发布queue
@RequestMapping("send")
public void send(String destination,String msg){
activeMqConf.sendQueueMsg(destination,msg);
}
//发布Topic
@RequestMapping("sendTopic")
public void sendTopic(String destination,String msg){
activeMqConf.sendTopicMsg(destination,msg);
}
}
4. 启动application,测试结果
(1)浏览器访问:
http://localhost:8081/queue/send?destination=springboot-queue&msg=xiaopang
ConsumerListener一直在监听着相关订阅的发布,一旦监听到了订阅的发布,则立即执行 consumer 的代码
(2)浏览器访问:
http://localhost:8081/queue/sendTopic?destination=springboot-topic&msg=xiaohei
发现控制台什么也没有打印,也就是没有监听到,原因是配置文件这个属性要改为true
#默认是false,为true开启发布订阅模式,同时点对点模式失效 spring.jms.pub-sub-domain=true
改完重新启动,再次访问,控制台就要监听到的消息了
扫描二维码关注公众号,回复:
8495824 查看本文章
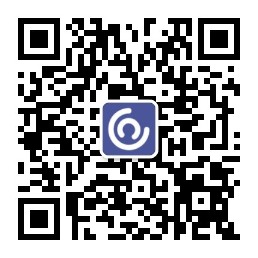
注意:改配置文件只能同时使用 queue 或者 topic 其中一种模式,两者不能同时使用,如果要配置同时使用,参考这篇博客