ActiveMQ
ActiveMQ 是Apache出品,最流行的,能力强劲的开源消息总线。ActiveMQ 是一个完全支持JMS1.1和J2EE 1.4规范的 JMS Provider实现,尽管JMS规范出台已经是很久的事情了,但是JMS在当今的J2EE应用中间仍然扮演着特殊的地位。
特性
多种语言和协议编写客户端。语言: Java,C,C++,C#,Ruby,Perl,Python,PHP。应用协议: OpenWire,Stomp REST,WS Notification,XMPP,AMQP
完全支持JMS1.1和J2EE 1.4规范 (持久化,XA消息,事务)
对Spring的支持,ActiveMQ可以很容易内嵌到使用Spring的系统里面去,而且也支持Spring2.0的特性
通过了常见J2EE服务器(如 Geronimo,JBoss 4,GlassFish,WebLogic)的测试,其中通过JCA 1.5 resource adaptors的配置,可以让ActiveMQ可以自动的部署到任何兼容J2EE 1.4 商业服务器上
支持多种传送协议:in-VM,TCP,SSL,NIO,UDP,JGroups,JXTA
支持通过JDBC和journal提供高速的消息持久化
从设计上保证了高性能的集群,客户端-服务器,点对点
支持Ajax
支持与Axis的整合
可以很容易的调用内嵌JMS provider,进行测试
更多关于 ActiveMQ 的内容可以点击这里。
首先添加依赖:
<!--ActiveMq依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-activemq</artifactId>
</dependency>
<!-- 启用JMS 的池化, 就一定要加上这个 jar-->
<dependency>
<groupId>org.apache.activemq</groupId>
<artifactId>activemq-pool</artifactId>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jms</artifactId>
</dependency>
<dependency>
<groupId>org.apache.activemq</groupId>
<artifactId>activemq-client</artifactId>
</dependency>
<!--ActiveMq依赖结束-->
application.yml添加如下内容
# activemq
activemq:
broker-url: tcp://localhost:61616
user: admin
password: admin
这里的端口号:61616 是默认的端口号,有些类似于es的和网页访问不一样网页访问的默认端口号:8161,写错误了会报47号错误
配置中还可以添加很多的东西 有兴趣的可以自行研究拓展下
添加
ActivemqConfig config类
package com.springboot2.config;
import javax.jms.ConnectionFactory;
import javax.jms.Destination;
import org.apache.activemq.command.ActiveMQQueue;
import org.apache.activemq.command.ActiveMQTopic;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jms.config.DefaultJmsListenerContainerFactory;
import org.springframework.jms.config.JmsListenerContainerFactory;
@Configuration
public class ActivemqConfig {
/**
* 自定义了4个Destination,两个queue,两个topic
*/
@Bean
public Destination queue1() {
return new ActiveMQQueue("queue-1");
}
@Bean
public Destination queue2() {
return new ActiveMQQueue("queue-2");
}
@Bean
public Destination topic1() {
return new ActiveMQTopic("topic-1");
}
@Bean
public Destination topic2() {
return new ActiveMQTopic("topic-2");
}
/**
* JmsListener注解默认只接收queue消息,如果要接收topic消息,需要设置containerFactory
*/
@Bean
public JmsListenerContainerFactory<?> topicListenerContainer(ConnectionFactory activeMQConnectionFactory) {
DefaultJmsListenerContainerFactory topicListenerContainer = new DefaultJmsListenerContainerFactory();
topicListenerContainer.setPubSubDomain(true);
topicListenerContainer.setConnectionFactory(activeMQConnectionFactory);
return topicListenerContainer;
}
}
注意这里bean下面的内容非常重要,不写的话接受普通的queue消息没有问题,但是接收不到topic的消息
下面写生产类,也就是消息的产生者发送类:
ProviderController
package com.springboot2.controller;
import javax.annotation.Resource;
import javax.jms.Destination;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jms.core.JmsTemplate;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* 通过页面访问的方式生产消息
*/
@RestController
public class ProviderController {
@Autowired
private JmsTemplate jmsTemplate;
@Resource(name = "queue1")
private Destination queue1;
@Resource(name = "topic1")
private Destination topic1;
@GetMapping("/send/queue1")
public String send1() {
jmsTemplate.convertAndSend(queue1, "hello queue-1");
return "ok";
}
@GetMapping("/send/topic1")
public String send2() {
jmsTemplate.convertAndSend(topic1, "hello topic-1");
return "ok";
}
}
下面是消费类,也就是接收消息类
MessageHandler
package com.springboot2.controller;
import org.springframework.jms.annotation.JmsListener;
import org.springframework.stereotype.Component;
/**
* 接收处理消息
*/
@Component
public class MessageHandler {
@JmsListener(destination="queue-1")
public void recieve1(String message) {
System.out.println("###################" + message + "###################");
}
@JmsListener(destination="topic-1", containerFactory="topicListenerContainer")
public void recieve2(String message) {
System.out.println("###################" + message + "###################");
}
}
可以看到上面的接收queue的消息和下面接收topic的消息有些不同
启动项目后先去生产消息,这里的jmslister会自动去打印消息
这里其实还有很多的拓展,例如:接收到消息后可以返回消息到mq中等等,可以根据自己的项目需求进行相关的修改,mq在mac下的安装方法已经在我的博客中了,有需要可以自取
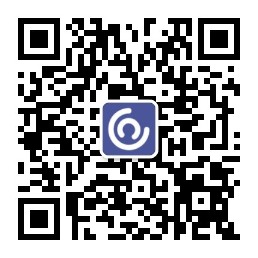
项目地址:https://download.csdn.net/download/qq_34077993/10751216